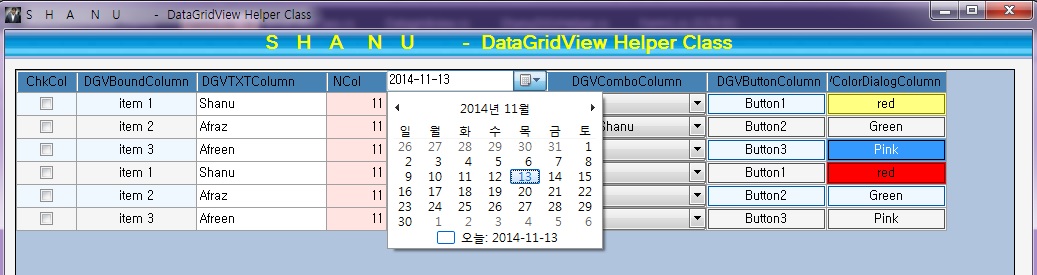
Introduction
In this article, I will explain how to create a Helper Class for DataGridView
in Winform Application. In one of my web projects, I want to create the datagridview
dynamically at runtime. When I start my project, I was looking for a Helper Class for DataGridView
and used Google to get one Helper Class. But I couldn’t find any helper class for DataGridView
. So I plan to create a Helper Class for DataGridView
. As a result, I have created a helper class for DataGridView
. I want to share my helper class with you so that you can use my class in your project and make a code that is simple and easy.
Why We Need Helper Class
- Helper Class will make our code part, Simple and Standard.
- All the Events of
DataGridview
can be defined in the helperClass
. In our form, we can call the method from helper class and make our code simple. - One Time Design in Class can be used for the whole project.
- This will be useful if we change design only in Class File and no need to redesign in each form.
What We Have in Helper Class
- Design your
Datagridview
, add the Datagridview
to your controls like Panel
, Tab
or in your form - Bound Column
CheckBox
Column TextBox
Column - Numeric
TextBox
Column ComboBox
Column DateTimePicker
Column Button
Column - Colour Dialog Column
DataGridView
Events like CellClick
, CellContentClick
, etc.
Here is an sample with Color Dialog from button Column.

Using the Code
First, we can start with the helper class and then we can see how to use the helper class in Winform. In my helper class, I have the following functions to make the design and bind simple.
Layout
Generategrid
Templatecolumn
NumeriTextboxEvents
DateTimePickerEvents
DGVClickEvents
colorDialogEvents
We can see few important functions of the class and then I will paste my full class code here.
Layout
This method will be setting the BackgroundColor
, BackColor
, AllowUserToAddRows
, etc. for the datagridView
. In this method, we pass our Datagridview
and setting all the design part for grid.
#region Layout
public static void Layouts(DataGridView ShanuDGV, Color BackgroundColor,
Color RowsBackColor, Color AlternatebackColor, Boolean AutoGenerateColumns,
Color HeaderColor, Boolean HeaderVisual, Boolean RowHeadersVisible,
Boolean AllowUserToAddRows)
{
ShanuDGV.BackgroundColor = BackgroundColor;
ShanuDGV.RowsDefaultCellStyle.BackColor = RowsBackColor;
ShanuDGV.AlternatingRowsDefaultCellStyle.BackColor = AlternatebackColor;
ShanuDGV.AutoGenerateColumns = AutoGenerateColumns;
ShanuDGV.ColumnHeadersDefaultCellStyle.BackColor = HeaderColor;
ShanuDGV.EnableHeadersVisualStyles = HeaderVisual;
ShanuDGV.RowHeadersVisible = RowHeadersVisible;
ShanuDGV.AllowUserToAddRows = AllowUserToAddRows;
}
#endregion
Generategrid
In this method, we pass our Datagridview
and set the height, width, position and bind the datagriview
to our selected control.
public static void Generategrid
(DataGridView ShanuDGV, Control cntrlName, int width, int height, int xval, int yval)
{
ShanuDGV.Location = new Point(xval, yval);
ShanuDGV.Size = new Size(width, height);
cntrlName.Controls.Add(ShanuDGV);
}
TemplateColumn
This is the important method in the helperclass
. Here, we pass the Datagriview
and define the column as Bound
, Checkbox
, Textbox DateTimePicker
, etc. Here, we set each column width, Alignment
, Visibility
, BackColor
, FontColor
, etc.
public static void Templatecolumn(DataGridView ShanuDGV, ShanuControlTypes ShanuControlTypes,
String cntrlnames, String Headertext, String ToolTipText, Boolean Visible, int width,
DataGridViewTriState Resizable, DataGridViewContentAlignment cellAlignment,
DataGridViewContentAlignment headerAlignment, Color CellTemplateBackColor,
DataTable dtsource, String DisplayMember, String ValueMember, Color CellTemplateforeColor)
{
switch (ShanuControlTypes)
{
case ShanuControlTypes.CheckBox:
DataGridViewCheckBoxColumn dgvChk = new DataGridViewCheckBoxColumn();
dgvChk.ValueType = typeof(bool);
dgvChk.Name = cntrlnames;
dgvChk.HeaderText = Headertext;
dgvChk.ToolTipText = ToolTipText;
dgvChk.Visible = Visible;
dgvChk.Width = width;
dgvChk.SortMode = DataGridViewColumnSortMode.Automatic;
dgvChk.Resizable = Resizable;
dgvChk.DefaultCellStyle.Alignment = cellAlignment;
dgvChk.HeaderCell.Style.Alignment = headerAlignment;
if (CellTemplateBackColor.Name.ToString() != "Transparent")
{
dgvChk.CellTemplate.Style.BackColor = CellTemplateBackColor;
}
dgvChk.DefaultCellStyle.ForeColor = CellTemplateforeColor;
ShanuDGV.Columns.Add(dgvChk);
break;
case ShanuControlTypes.BoundColumn:
DataGridViewColumn col = new DataGridViewTextBoxColumn();
col.DataPropertyName = cntrlnames;
col.Name = cntrlnames;
col.HeaderText = Headertext;
col.ToolTipText = ToolTipText;
col.Visible = Visible;
col.Width = width;
col.SortMode = DataGridViewColumnSortMode.Automatic;
col.Resizable = Resizable;
col.DefaultCellStyle.Alignment = cellAlignment;
col.HeaderCell.Style.Alignment = headerAlignment;
if (CellTemplateBackColor.Name.ToString() != "Transparent")
{
col.CellTemplate.Style.BackColor = CellTemplateBackColor;
}
col.DefaultCellStyle.ForeColor = CellTemplateforeColor;
ShanuDGV.Columns.Add(col);
break;
case ShanuControlTypes.TextBox:
DataGridViewTextBoxColumn dgvText = new DataGridViewTextBoxColumn();
dgvText.ValueType = typeof(decimal);
dgvText.DataPropertyName = cntrlnames;
dgvText.Name = cntrlnames;
dgvText.HeaderText = Headertext;
dgvText.ToolTipText = ToolTipText;
dgvText.Visible = Visible;
dgvText.Width = width;
dgvText.SortMode = DataGridViewColumnSortMode.Automatic;
dgvText.Resizable = Resizable;
dgvText.DefaultCellStyle.Alignment = cellAlignment;
dgvText.HeaderCell.Style.Alignment = headerAlignment;
if (CellTemplateBackColor.Name.ToString() != "Transparent")
{
dgvText.CellTemplate.Style.BackColor = CellTemplateBackColor;
}
dgvText.DefaultCellStyle.ForeColor = CellTemplateforeColor;
ShanuDGV.Columns.Add(dgvText);
break;
case ShanuControlTypes.ComboBox:
DataGridViewComboBoxColumn dgvcombo = new DataGridViewComboBoxColumn();
dgvcombo.ValueType = typeof(decimal);
dgvcombo.Name = cntrlnames;
dgvcombo.DataSource = dtsource;
dgvcombo.DisplayMember = DisplayMember;
dgvcombo.ValueMember = ValueMember;
dgvcombo.Visible = Visible;
dgvcombo.Width = width;
dgvcombo.SortMode = DataGridViewColumnSortMode.Automatic;
dgvcombo.Resizable = Resizable;
dgvcombo.DefaultCellStyle.Alignment = cellAlignment;
dgvcombo.HeaderCell.Style.Alignment = headerAlignment;
if (CellTemplateBackColor.Name.ToString() != "Transparent")
{
dgvcombo.CellTemplate.Style.BackColor = CellTemplateBackColor;
}
dgvcombo.DefaultCellStyle.ForeColor = CellTemplateforeColor;
ShanuDGV.Columns.Add(dgvcombo);
break;
case ShanuControlTypes.Button:
DataGridViewButtonColumn dgvButtons = new DataGridViewButtonColumn();
dgvButtons.Name = cntrlnames;
dgvButtons.FlatStyle = FlatStyle.Popup;
dgvButtons.DataPropertyName = cntrlnames;
dgvButtons.Visible = Visible;
dgvButtons.Width = width;
dgvButtons.SortMode = DataGridViewColumnSortMode.Automatic;
dgvButtons.Resizable = Resizable;
dgvButtons.DefaultCellStyle.Alignment = cellAlignment;
dgvButtons.HeaderCell.Style.Alignment = headerAlignment;
if (CellTemplateBackColor.Name.ToString() != "Transparent")
{
dgvButtons.CellTemplate.Style.BackColor = CellTemplateBackColor;
}
dgvButtons.DefaultCellStyle.ForeColor = CellTemplateforeColor;
ShanuDGV.Columns.Add(dgvButtons);
break;
}
}
NumerictextBoxEvent
In this method, we pass the Datagridview
and list of ColumnIndex
which needs to be set as NumericTextbox
Column
. Using the EditingControlShowing
Event of Gridview
, I check for all the columns which need to be accept only numbers from the textbox
.
public void NumeriTextboxEvents(DataGridView ShanuDGV,List<int> columnIndexs)
{
shanuDGVs = ShanuDGV;
listcolumnIndex=columnIndexs;
ShanuDGV.EditingControlShowing +=
new DataGridViewEditingControlShowingEventHandler
(dShanuDGV_EditingControlShowing);
}
private void dShanuDGV_EditingControlShowing
(object sender, DataGridViewEditingControlShowingEventArgs e)
{
e.Control.KeyPress -=
new KeyPressEventHandler(itemID_KeyPress);
if (listcolumnIndex.Contains(shanuDGVs.CurrentCell.ColumnIndex))
{
TextBox itemID = e.Control as TextBox;
if (itemID != null)
{
itemID.KeyPress += new KeyPressEventHandler(itemID_KeyPress);
}
}
}
private void itemID_KeyPress(object sender, KeyPressEventArgs e)
{
if (!char.IsControl(e.KeyChar)
&& !char.IsDigit(e.KeyChar))
{
e.Handled = true;
}
}
DatagridView helperClass Full Source Code
Here is the full source code of the Datagridview
helper class. I have created all the necessary methods which need to be used. If user needs more functions, they can add those functions as well here in this class and use in your projects.
class ShanuDGVHelper
{
#region Variables
public DataGridView shanuDGVs = new DataGridView();
List<int> listcolumnIndex;
int DateColumnIndex=0;
int ColorColumnIndex = 0;
int ClickColumnIndex = 0;
DateTimePicker shanuDateTimePicker;
String EventFucntions;
# endregion
#region Layout
public static void Layouts(DataGridView ShanuDGV, Color BackgroundColor,
Color RowsBackColor, Color AlternatebackColor, Boolean AutoGenerateColumns,
Color HeaderColor, Boolean HeaderVisual, Boolean RowHeadersVisible,
Boolean AllowUserToAddRows)
{
ShanuDGV.BackgroundColor = BackgroundColor;
ShanuDGV.RowsDefaultCellStyle.BackColor = RowsBackColor;
ShanuDGV.AlternatingRowsDefaultCellStyle.BackColor = AlternatebackColor;
ShanuDGV.AutoGenerateColumns = AutoGenerateColumns;
ShanuDGV.ColumnHeadersDefaultCellStyle.BackColor = HeaderColor;
ShanuDGV.EnableHeadersVisualStyles = HeaderVisual;
ShanuDGV.RowHeadersVisible = RowHeadersVisible;
ShanuDGV.AllowUserToAddRows = AllowUserToAddRows;
}
#endregion
#region Variables
public static void Generategrid(DataGridView ShanuDGV,
Control cntrlName, int width, int height, int xval, int yval)
{
ShanuDGV.Location = new Point(xval, yval);
ShanuDGV.Size = new Size(width, height);
cntrlName.Controls.Add(ShanuDGV);
}
#endregion
#region Templatecolumn
public static void Templatecolumn(DataGridView ShanuDGV,
ShanuControlTypes ShanuControlTypes, String cntrlnames, String Headertext,
String ToolTipText, Boolean Visible, int width, DataGridViewTriState Resizable,
DataGridViewContentAlignment cellAlignment,
DataGridViewContentAlignment headerAlignment,
Color CellTemplateBackColor, DataTable dtsource, String DisplayMember,
String ValueMember, Color CellTemplateforeColor)
{
switch (ShanuControlTypes)
{
case ShanuControlTypes.CheckBox:
DataGridViewCheckBoxColumn dgvChk = new DataGridViewCheckBoxColumn();
dgvChk.ValueType = typeof(bool);
dgvChk.Name = cntrlnames;
dgvChk.HeaderText = Headertext;
dgvChk.ToolTipText = ToolTipText;
dgvChk.Visible = Visible;
dgvChk.Width = width;
dgvChk.SortMode = DataGridViewColumnSortMode.Automatic;
dgvChk.Resizable = Resizable;
dgvChk.DefaultCellStyle.Alignment = cellAlignment;
dgvChk.HeaderCell.Style.Alignment = headerAlignment;
if (CellTemplateBackColor.Name.ToString() != "Transparent")
{
dgvChk.CellTemplate.Style.BackColor = CellTemplateBackColor;
}
dgvChk.DefaultCellStyle.ForeColor = CellTemplateforeColor;
ShanuDGV.Columns.Add(dgvChk);
break;
case ShanuControlTypes.BoundColumn:
DataGridViewColumn col = new DataGridViewTextBoxColumn();
col.DataPropertyName = cntrlnames;
col.Name = cntrlnames;
col.HeaderText = Headertext;
col.ToolTipText = ToolTipText;
col.Visible = Visible;
col.Width = width;
col.SortMode = DataGridViewColumnSortMode.Automatic;
col.Resizable = Resizable;
col.DefaultCellStyle.Alignment = cellAlignment;
col.HeaderCell.Style.Alignment = headerAlignment;
if (CellTemplateBackColor.Name.ToString() != "Transparent")
{
col.CellTemplate.Style.BackColor = CellTemplateBackColor;
}
col.DefaultCellStyle.ForeColor = CellTemplateforeColor;
ShanuDGV.Columns.Add(col);
break;
case ShanuControlTypes.TextBox:
DataGridViewTextBoxColumn dgvText = new DataGridViewTextBoxColumn();
dgvText.ValueType = typeof(decimal);
dgvText.DataPropertyName = cntrlnames;
dgvText.Name = cntrlnames;
dgvText.HeaderText = Headertext;
dgvText.ToolTipText = ToolTipText;
dgvText.Visible = Visible;
dgvText.Width = width;
dgvText.SortMode = DataGridViewColumnSortMode.Automatic;
dgvText.Resizable = Resizable;
dgvText.DefaultCellStyle.Alignment = cellAlignment;
dgvText.HeaderCell.Style.Alignment = headerAlignment;
if (CellTemplateBackColor.Name.ToString() != "Transparent")
{
dgvText.CellTemplate.Style.BackColor = CellTemplateBackColor;
}
dgvText.DefaultCellStyle.ForeColor = CellTemplateforeColor;
ShanuDGV.Columns.Add(dgvText);
break;
case ShanuControlTypes.ComboBox:
DataGridViewComboBoxColumn dgvcombo = new DataGridViewComboBoxColumn();
dgvcombo.ValueType = typeof(decimal);
dgvcombo.Name = cntrlnames;
dgvcombo.DataSource = dtsource;
dgvcombo.DisplayMember = DisplayMember;
dgvcombo.ValueMember = ValueMember;
dgvcombo.Visible = Visible;
dgvcombo.Width = width;
dgvcombo.SortMode = DataGridViewColumnSortMode.Automatic;
dgvcombo.Resizable = Resizable;
dgvcombo.DefaultCellStyle.Alignment = cellAlignment;
dgvcombo.HeaderCell.Style.Alignment = headerAlignment;
if (CellTemplateBackColor.Name.ToString() != "Transparent")
{
dgvcombo.CellTemplate.Style.BackColor = CellTemplateBackColor;
}
dgvcombo.DefaultCellStyle.ForeColor = CellTemplateforeColor;
ShanuDGV.Columns.Add(dgvcombo);
break;
case ShanuControlTypes.Button:
DataGridViewButtonColumn dgvButtons = new DataGridViewButtonColumn();
dgvButtons.Name = cntrlnames;
dgvButtons.FlatStyle = FlatStyle.Popup;
dgvButtons.DataPropertyName = cntrlnames;
dgvButtons.Visible = Visible;
dgvButtons.Width = width;
dgvButtons.SortMode = DataGridViewColumnSortMode.Automatic;
dgvButtons.Resizable = Resizable;
dgvButtons.DefaultCellStyle.Alignment = cellAlignment;
dgvButtons.HeaderCell.Style.Alignment = headerAlignment;
if (CellTemplateBackColor.Name.ToString() != "Transparent")
{
dgvButtons.CellTemplate.Style.BackColor = CellTemplateBackColor;
}
dgvButtons.DefaultCellStyle.ForeColor = CellTemplateforeColor;
ShanuDGV.Columns.Add(dgvButtons);
break;
}
}
#endregion
#region Numeric Textbox Events
public void NumeriTextboxEvents(DataGridView ShanuDGV,List<int> columnIndexs)
{
shanuDGVs = ShanuDGV;
listcolumnIndex=columnIndexs;
ShanuDGV.EditingControlShowing +=
new DataGridViewEditingControlShowingEventHandler
(dShanuDGV_EditingControlShowing);
}
private void dShanuDGV_EditingControlShowing
(object sender, DataGridViewEditingControlShowingEventArgs e)
{
e.Control.KeyPress -=
new KeyPressEventHandler(itemID_KeyPress);
if (listcolumnIndex.Contains(shanuDGVs.CurrentCell.ColumnIndex))
{
TextBox itemID = e.Control as TextBox;
if (itemID != null)
{
itemID.KeyPress += new KeyPressEventHandler(itemID_KeyPress);
}
}
}
private void itemID_KeyPress(object sender, KeyPressEventArgs e)
{
if (!char.IsControl(e.KeyChar)
&& !char.IsDigit(e.KeyChar))
{
e.Handled = true;
}
}
#endregion
#region DateTimePicker control to textbox column
public void DateTimePickerEvents
(DataGridView ShanuDGV, int columnIndexs,ShanuEventTypes eventtype)
{
shanuDGVs = ShanuDGV;
DateColumnIndex = columnIndexs;
ShanuDGV.CellClick += new DataGridViewCellEventHandler(shanuDGVs_CellClick);
}
private void shanuDGVs_CellClick(object sender, DataGridViewCellEventArgs e)
{
if (e.ColumnIndex == DateColumnIndex)
{
shanuDateTimePicker = new DateTimePicker();
shanuDGVs.Controls.Add(shanuDateTimePicker);
shanuDateTimePicker.Format = DateTimePickerFormat.Short;
Rectangle dgvRectangle =
shanuDGVs.GetCellDisplayRectangle(e.ColumnIndex, e.RowIndex, true);
shanuDateTimePicker.Size = new Size(dgvRectangle.Width, dgvRectangle.Height);
shanuDateTimePicker.Location = new Point(dgvRectangle.X, dgvRectangle.Y);
}
}
#endregion
#region Button Click Event
public void DGVClickEvents(DataGridView ShanuDGV, int columnIndexs,
ShanuEventTypes eventtype)
{
shanuDGVs = ShanuDGV;
ClickColumnIndex = columnIndexs;
ShanuDGV.CellContentClick += new DataGridViewCellEventHandler
(shanuDGVs_CellContentClick_Event);
}
private void shanuDGVs_CellContentClick_Event
(object sender, DataGridViewCellEventArgs e)
{
if (e.ColumnIndex == ClickColumnIndex)
{
MessageBox.Show("Button Clicked " +
shanuDGVs.Rows[e.RowIndex].Cells
[e.ColumnIndex].Value.ToString());
}
}
#endregion
#region Button Click Event to show Color Dialog
public void colorDialogEvents(DataGridView ShanuDGV,
int columnIndexs, ShanuEventTypes eventtype)
{
shanuDGVs = ShanuDGV;
ColorColumnIndex = columnIndexs;
ShanuDGV.CellContentClick += new DataGridViewCellEventHandler
(shanuDGVs_CellContentClick);
}
private void shanuDGVs_CellContentClick(object sender, DataGridViewCellEventArgs e)
{
if (e.ColumnIndex == ColorColumnIndex)
{
MessageBox.Show("Button Clicked " +
shanuDGVs.Rows[e.RowIndex].Cells[e.ColumnIndex].Value.ToString());
ColorDialog cd = new ColorDialog();
cd.ShowDialog();
shanuDGVs.Rows[e.RowIndex].Cells[e.ColumnIndex].Style.BackColor = cd.Color;
}
}
#endregion
}
Now, let’s see how to use this Helper Class in our winform project.
- Add the helperClass file in to your project.
- In your form
Load
, call a method to create your Datagridview
dynamically and call the functions to design, bind and set the each column of your grid.
Here, we can see that I have created a method called "generatedgvColumns
” and called this method in my Form Load
Event.
public void generatedgvColumns()
{
ShanuDGVHelper.Layouts(shanuDGV, Color.LightSteelBlue,
Color.AliceBlue, Color.WhiteSmoke, false, Color.SteelBlue, false, false, false);
ShanuDGVHelper.Generategrid(shanuDGV, pnlShanuGrid, 1000, 600, 10, 10);
ShanuDGVHelper.Templatecolumn(shanuDGV, ShanuControlTypes.CheckBox,
"Chk", "ChkCol", "Check Box Column", true,
60, DataGridViewTriState.True, DataGridViewContentAlignment.MiddleCenter,
DataGridViewContentAlignment.MiddleCenter, Color.Transparent, null,
"", "", Color.Black);
ShanuDGVHelper.Templatecolumn(shanuDGV, ShanuControlTypes.BoundColumn,
"DGVBoundColumn", "DGVBoundColumn", "Bound Column",
true, 120, DataGridViewTriState.True, DataGridViewContentAlignment.MiddleCenter,
DataGridViewContentAlignment.MiddleCenter, Color.Transparent,
null, "", "", Color.Black);
ShanuDGVHelper.Templatecolumn(shanuDGV, ShanuControlTypes.TextBox,
"DGVTXTColumn", "DGVTXTColumn", "textBox Column",
true, 130, DataGridViewTriState.True, DataGridViewContentAlignment.MiddleLeft,
DataGridViewContentAlignment.MiddleLeft, Color.White, null, "", "", Color.Black);
ShanuDGVHelper.Templatecolumn(shanuDGV, ShanuControlTypes.TextBox,
"DGVNumericTXTColumn", "NCol", "textBox Column",
true, 60, DataGridViewTriState.True, DataGridViewContentAlignment.MiddleRight,
DataGridViewContentAlignment.MiddleCenter, Color.MistyRose,
null, "", "", Color.Black);
ShanuDGVHelper.Templatecolumn(shanuDGV, ShanuControlTypes.BoundColumn,
"DGVDateTimepicker", "DGVDateTimepicker", "For Datetime Column",
true, 160, DataGridViewTriState.True, DataGridViewContentAlignment.MiddleLeft,
DataGridViewContentAlignment.MiddleLeft, Color.Transparent,
null, "", "", Color.Black);
ShanuDGVHelper.Templatecolumn(shanuDGV, ShanuControlTypes.ComboBox,
"DGVComboColumn", "ComboCol", "Combo Column",
true, 160, DataGridViewTriState.True, DataGridViewContentAlignment.MiddleCenter,
DataGridViewContentAlignment.MiddleRight, Color.Transparent, dtName,
"Name", "Value", Color.Black);
ShanuDGVHelper.Templatecolumn(shanuDGV, ShanuControlTypes.Button,
"DGVButtonColumn", "ButtonCol", "Button Column",
true, 120, DataGridViewTriState.True, DataGridViewContentAlignment.MiddleCenter,
DataGridViewContentAlignment.MiddleRight, Color.Transparent, null,
"", "", Color.Black);
ShanuDGVHelper.Templatecolumn(shanuDGV, ShanuControlTypes.Button,
"DGVColorDialogColumn", "ButtonCol", "Button Column",
true, 120, DataGridViewTriState.True, DataGridViewContentAlignment.MiddleCenter,
DataGridViewContentAlignment.MiddleRight, Color.Transparent, null,
"", "", Color.Black);
lstNumericTextBoxColumns = new List<int>();
lstNumericTextBoxColumns.Add(shanuDGV.Columns["DGVNumericTXTColumn"].Index);
objshanudgvHelper.NumeriTextboxEvents(shanuDGV, lstNumericTextBoxColumns);
objshanudgvHelper.DateTimePickerEvents
(shanuDGV, shanuDGV.Columns["DGVDateTimepicker"].Index,
ShanuEventTypes.CellClick);
objshanudgvHelper.colorDialogEvents
(shanuDGV, shanuDGV.Columns["DGVColorDialogColumn"].Index,
ShanuEventTypes.cellContentClick);
objshanudgvHelper.DGVClickEvents
(shanuDGV, shanuDGV.Columns["DGVButtonColumn"].Index,
ShanuEventTypes.cellContentClick);
shanuDGV.DataSource = objDGVBind;
}
- "
ShanuDGVHelper.Layouts()
": This method is used to set the layout of grid like autogenerated or not, BackgroundColor
, AlternatebackColor
, RowHeadersVisible
, etc. - "
ShanuDGVHelper.Generategrid()
": This method is used to set the Height
, Width
and add the datagridview
to our selected control, for example, here I have added the DGV to a panel control. - "
ShanuDGVHelper.Templatecolumn
": This method is used to define our Column type as Checkbox
, Textbox
, Combobox
, etc. To this method, we pass the Column Name, Column Header Text, Column Width, Column Back Color, etc.
Points of Interest
Hope this Datagridview
Helper Class will be useful for the readers.
History
- 13th November, 2014: Version 1.0