In this article, I explain how to play Audio/Video and Youtube Video in our Windows application using C#. Add your Audio and Video Files to playlist and play it from your WinForm. Paste your Youtube URL and play it from your WinForm.
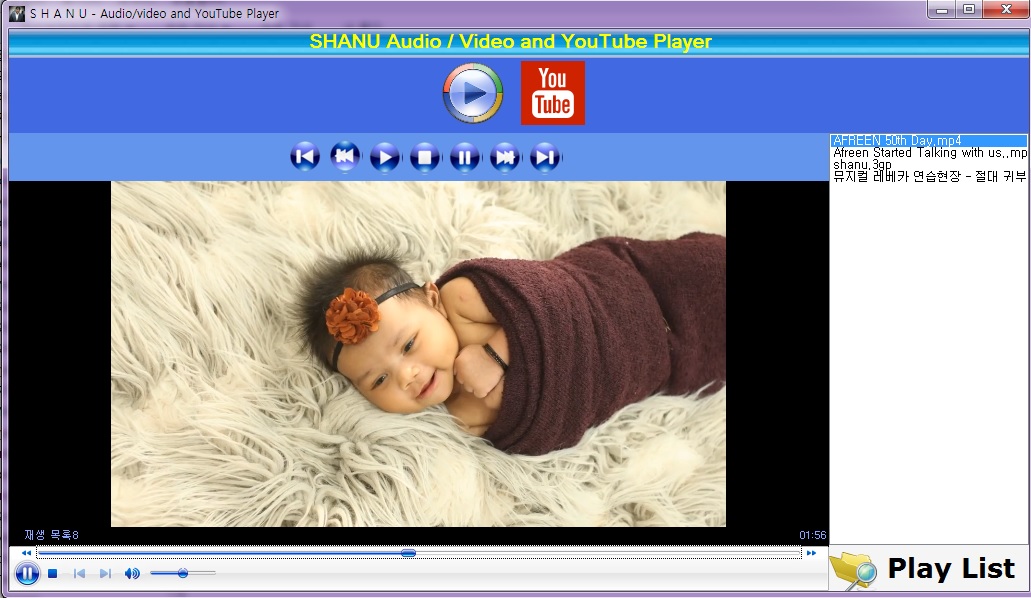
Introduction
The main purpose of this article is to explain how to create a simple Audio/Video and YouTube Video for Windows applications using C#. User can select Audio or Video file and add to the Playlist and play the songs or the video file. I have used two Com Components to play Audio /Video and for playing the YouTube Video URL. In my project, I have used the following Com Components:
- Windows Media Player object
- Shockwave flash object
Audio/Video Player
Any audio or video files which are supported by Windows Media player can be played in my application. The first and important thing is we need to add the Windows Media Player Com Component to our project.
How to add Windows Media Player Com Component to our Windows Application?
- Create your Windows Application.
- From Tools Windows, click Choose Items.
- Select Com Components Tab.
- Search for "Windows Media Player" and click OK.

Now you can see that Windows Media player will be added in your Tools windows. Just drag and drop the control to your winform.
Here, you can see my Audio/Video Player screen:

My audio/video player has features like:
- Load Audio/Video File and Add to Playlist
- Play Audio/Video File
- Pause Audio/Video File
- Stop Audio/Video File
- Play Previous Song
- Play Next Song
- Play First Song of Play List
- Play Last Song of Play List
YouTube Player
To play any YouTube URL Video in our Windows Application, we can use Shockwave Flash Object Com Component.
How to add Shockwave Flash Object Com Component to our Windows Application?
- Create your Windows Application.
- From Tools Windows, click Choose Items.
- Select Com Components Tab.
- Search for "Shockwave Flash Object" and click OK.

Now you can see the Shockwave Flash Object will be added in your Tools windows. Just drag and drop the control to your winform.
Here, you can see my YouTube screen.

* Note: To play the YouTube video in our Shockwave Flash Object, the YouTube URL should be changed edited.
For example, we have YouTube URL "https://www.youtube.com/watch?v=Ce_Ne5P02q0".
To play this video, we need to delete "watch?" from the URL and also we need to replace the "=
" next to "v" as "/
".
So here for example, the about actual URL should be edited like this "http://www.youtube.com/v/Ce_Ne5P02q0" .
If we do not edit the url like above, it will not play in the Shockwave.
Using the Code
Audio/Video Player Code
- Load Audio and Video file to our playlist. Here, using the Open File Dialog, we can filter all our Audio and Video files. Add all the File name and path to String Array and bind to the List Box.
private void btnLoadFile_Click(object sender, EventArgs e)
{
Startindex = 0;
playnext = false;
OpenFileDialog opnFileDlg = new OpenFileDialog();
opnFileDlg.Multiselect = true;
opnFileDlg.Filter = "(mp3,wav,mp4,mov,wmv,mpg,avi,3gp,flv)|*.mp3;
*.wav;*.mp4;*.3gp;*.avi;*.mov;*.flv;*.wmv;*.mpg|all files|*.*";
if (opnFileDlg.ShowDialog() == DialogResult.OK)
{
FileName = opnFileDlg.SafeFileNames;
FilePath = opnFileDlg.FileNames;
for (int i = 0; i <= FileName.Length - 1; i++)
{
listBox1.Items.Add(FileName[i]);
}
Startindex = 0;
playfile(0);
}
}
- This method will be called from First, Next, Previous, Last and from List Box Selected index Change Event with passing the “
selectedindex
” value. In this method, from the array, check for the selected file and play using the "WindowsMediaPlayer.URL
".
public void playfile(int playlistindex)
{
if (listBox1.Items.Count <= 0)
{ return; }
if (playlistindex < 0)
{
return;
}
WindowsMediaPlayer.settings.autoStart = true;
WindowsMediaPlayer.URL = FilePath[playlistindex];
WindowsMediaPlayer.Ctlcontrols.next();
WindowsMediaPlayer.Ctlcontrols.play();
}
- Windows Media Player “
PlayStateChange
” event: This is Windows Media Player event which will be triggered whenever the player plays, pauses, stops, etc. Here, I have used this method to check for the Song or video file when plays Finish or end. If the song ends, then I set the "playnext = true
". In my program, I have used the Timer
control which will check for the "playnext = true
" status and plays the next song.
private void WindowsMediaPlayer_PlayStateChange
(object sender, AxWMPLib._WMPOCXEvents_PlayStateChangeEvent e)
{
int statuschk = e.newState;
if (statuschk == 8)
{
statuschk = e.newState;
if (Startindex == listBox1.Items.Count - 1)
{
Startindex = 0;
}
else if (Startindex >= 0 && Startindex < listBox1.Items.Count - 1)
{
Startindex = Startindex + 1;
}
playnext = true;
}
- Windows Media Player has methods like
play
, pause
and stop
the player.
WindowsMediaPlayer.Ctlcontrols.play();
WindowsMediaPlayer.Ctlcontrols.pause();
WindowsMediaPlayer.Ctlcontrols.stop();
Youtube Video Player: This is simple and easy to use object. The Shockwave
object has Movie
property. Here, we can give our YouTube Video to play.
Here in button click, I give the input of textbox to Shockwave Flash
object movie
property.
private void btnYoutube_Click(object sender, EventArgs e)
{
ShockwaveFlash.Movie = txtUtube.Text.Trim();
}
History
- 20th November, 2014: Initial release