This is another article in my on going learning/experimenting with PowerShell. This time, I will show how you can use PowerShell to carry out REST operations such as GET
/POST
.
Now there may be some amongst you, who go why didn’t you just use WGet, which is a downloadable thing, and I could have indeed used that, except for the part that the machine I need to run this on, is so locked down that I can only use things that are already installed. So raw PowerShell it is.
Here is the relevant PowerShell code, which allows 3 parameters to control the script:
Target
: The url Verb
: This is the http verb, GET
, PU
T, etc. Content
: This could be some content when doing a POST
request for example
It just makes use of some very standard .NET classes namely WebRequest
.
[CmdletBinding()]
Param(
[Parameter(Mandatory=$True,Position=1)]
[string] $target,
[Parameter(Mandatory=$True,Position=2)]
[string] $verb,
[Parameter(Mandatory=$False,Position=3)]
[string] $content
)
write-host "Http Url: $target"
write-host "Http Verb: $verb"
write-host "Http Content: $content"
$webRequest = [System.Net.WebRequest]::Create($target)
$encodedContent = [System.Text.Encoding]::UTF8.GetBytes($content)
$webRequest.Method = $verb
write-host "UTF8 Encoded Http Content: $content"
if($encodedContent.length -gt 0) {
$webRequest.ContentLength = $encodedContent.length
$requestStream = $webRequest.GetRequestStream()
$requestStream.Write($encodedContent, 0, $encodedContent.length)
$requestStream.Close()
}
[System.Net.WebResponse] $resp = $webRequest.GetResponse();
if($resp -ne $null)
{
$rs = $resp.GetResponseStream();
[System.IO.StreamReader] $sr = New-Object System.IO.StreamReader -argumentList $rs;
[string] $results = $sr.ReadToEnd();
return $results
}
else
{
exit ''
}
Here is an example usage (assuming the above script is saved as http.ps1 somewhere):
Http.ps1 - target "http://www.google.com" -verb "GET
"
which would give the following sort of output:
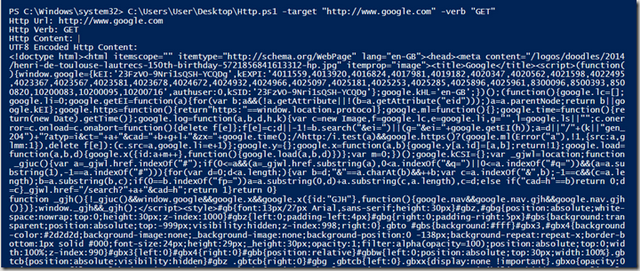
Anyway that’s it for now, hope this helps!