In this post, we will learn how to get started with React.js in ASP.NET MVC.
What is React?
React
is a UI library developed at Facebook. It is a JavaScript library for building user interfaces. It is used by Facebook and Instagram. JSX is the extension for the React.
React provides two features:
- Rendering DOM (Rendering the model to the DOM and keeping the DOM synchronized with changes to the model)
- Events
Top Feature
- React top feature is SPEED.
You can check or compare the react js performance features here.
What is JSX?
It is a JavaScript XML syntax transform. JSX supports XML like syntax inline in JavaScript. Each element is converted into a JavaScript function call - http://facebook.github.io/react/jsx-compiler.html
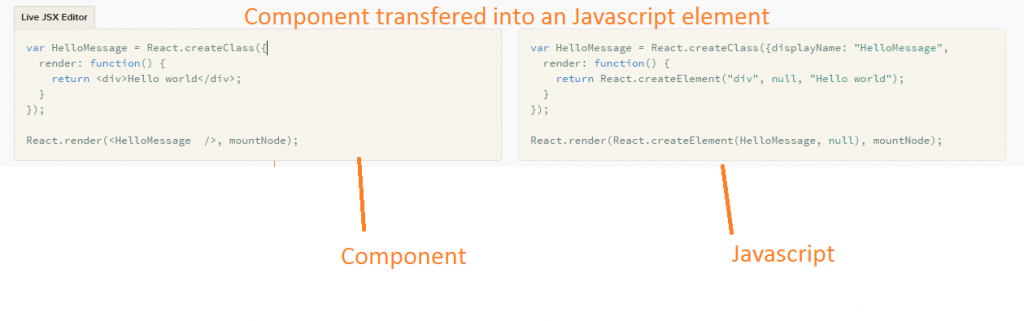
JSX
Components
A react consists of a set of components.
The inputs to the components are referred as properties called as props and state, the difference between two is the state can change at any time.
To render any element, we use renderComponent
method.
Example for renderComponent
React.renderComponent(
<h1>ReactJS.Net in ASP.NET MVC 5</h1>,
document.getElementById('myDiv')
);
In the above syntax, the first argument is the component we want to render, the second argument is the DOM node it should bind to. We can use the createClass
method to create custom component classes. It takes an object of specifications as its argument.
Example for renderClass
var Component = React.createClass({
render: function(){
return (
<h1>Hello, world!</h1>
);
}
});
After creating a class, we can render it in our HTML document.
React.renderComponent(
<Component/>,
document.getElementById('myDiv')
);
ReactJS.NET
ReactJS.NET makes it easier to use Facebook’s React and JSX from C# and other .NET languages, focusing specifically on ASP.NET MVC. It supports on-the-fly JSX to JavaScript compilation, also bundling and minification.
Create an ASP.NET MVC Application

Install React.js and ReactJS.NET from the Nuget console package manager using the following command Package Manager Console.
PM> Install-Package react.js
Now install ReactJS.NET (MVC 4 and 5):
PM> Install-Package React.Web.Mvc4
Now reference the React.js reference in Index.cshtml file:
@section Script{
<script src="~/Scripts/react/react-0.12.2.min.js"></script>
}
Hello World Example
Create HelloWorld.jsx in the scripts folder.

Now, add the HelloWorld.jsx in the View page.
<script src="@Url.Content("~/Scripts/HelloWorld.jsx")"></script>
Now, we will create a component that renders Hello World. Before that, we create a div
with id container in the index page to bind the Hello World.
<div id="content"></div>
HelloWorld.jsx
var App = React.createClass({
render: function(){
return(
<h1> Hello World! </h1>
);
}
});
React.render(<App/>, document.getElementById('content'));
Display Data from the Server Side or Controller
Now create an ActionMethod
that will return static
message in the ReactJS Controller.
public class ReactJSController : Controller
{
public ActionResult Index()
{
return View();
}
public JsonResult GetMessage()
{
return Json(new { result = "Hello World From ReactJS Controller" }, JsonRequestBehavior.AllowGet);
}
}
Now, get the data into the component and render it into the div
.
var App = React.createClass({
getInitialState: function(){
return{data: ''};
},
componentWillMount: function(){
var xhr = new XMLHttpRequest();
xhr.open('get', this.props.url, true);
xhr.onload = function() {
var response = JSON.parse(xhr.responseText);
this.setState({ data: response.result });
}.bind(this);
xhr.send();
},
render: function(){
return(
<h1>{this.state.data}</h1>
);
}
});
React.renderComponent(<App url="/ReactJS/GetMessage" />, document.getElementById('content'));
Output

Getting Started with React.js in ASP.NET MVC
Source Code
You can download the source from Github.
The post Getting Started with React.js in ASP.NET MVC appeared first on Venkat Baggu Blog.