Introduction
Most aspects of balloons message can be controlled through the plugin...
In this tip, I will show how to create a balloon message in a simple way using JavaScript & CSS.
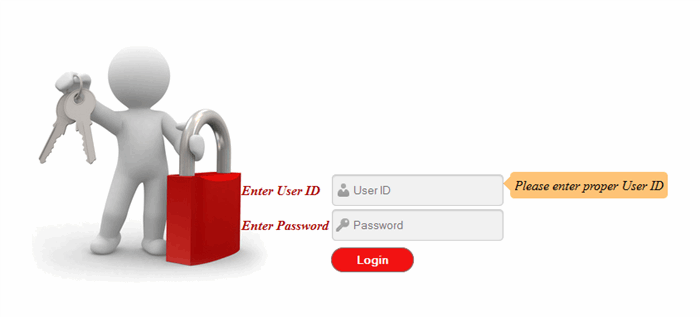
Using the Code
How to achieve balloon message without using any plugin:
Step A: Find coordinates of control where to show balloon message.
Step A.1: Write function to find coordinates of control like TextBox
, dropdown
, etc.
function getOffsetRect(elem)
{
var box = elem.getBoundingClientRect()
var body = document.body
var docElem = document.documentElement
var scrollTop = window.pageYOffset || docElem.scrollTop || body.scrollTop
var scrollLeft = window.pageXOffset || docElem.scrollLeft || body.scrollLeft
var clientTop = docElem.clientTop || body.clientTop || 0
var clientLeft = docElem.clientLeft || body.clientLeft || 0
var top = box.top + scrollTop - clientTop
var left = box.left + scrollLeft - clientLeft
return { top: Math.round(top), left: Math.round(left) }
}
Step A.2: Write a function to show balloon message & validate your control with the help of CSS & JavaScript.
The below function requires three parameters:
ControlID
like TextBox
, DropDown
, etc. SpanID
-->(span tag ID) for adding a hook to a part of a balloon message - Duration -->Hide Error message after specific time
- Error Message
function RequiredFieldValidation(TextBoxID, SpanID,Duration,ErrorMsg) {
var j = getOffsetRect(TextBoxID);
AutoHide(SpanID, Duration);
var Width = TextBoxID.clientWidth + 10 + j.left;
if (TextBoxID.value == "") {
SpanID.className = "ShowBubble";
SpanID.style.top = j.top + "px";
SpanID.style.left = Width + "px";
SpanID.innerHTML = ErrorMsg;
return false;
}
return true;
}
CSS-->
.ShowBubble
{
z-index: 1;
position: absolute;
margin-top: -3px;
padding: 6px 5px;
background: #ffc477;
-webkit-border-radius: 6px;
-moz-border-radius: 6px;
border-radius: 5px;
width: Auto;
height: Auto;
font-style:italic;
}
.HideBubble
{
display: none;
}
.ShowBubble:after
{
content: '';
position: absolute;
border-style: solid;
border-width: 15px 15px 15px 0;
border-color: transparent #ffc477 transparent;
display: block;
width: 0;
z-index: 1;
left: -9px;
top: -1px;
}
If you would like to learn more about JavaScript coordinates, refer to the below link:
History
- 14th February, 2015: Initial version