Introduction
I will make a series of blog posts how to use the internal Microsoft Test Manager TFS API using C# code.
The first thing you have to do in order to use the Test Manager API is to add references to the Microsoft TFS DLLs. Most of them are available only after the installation of Visual Studio 2012/2013 and Microsoft Test Manager, which is included only in the Test, PRO, Premium and Ultimate versions of the product.
The default path to the most of the DLLs that you will need is the following: C:\Program Files (x86)\Microsoft Visual Studio 11.0\Common7\IDE\ReferenceAssemblies\v2.0\.
Most of them are available in the GAC but if you are going to use your application on a machine where the Visual Studio is not installed, probably you should consider the idea to create a NuGet package for the needed DLLs.
In order to begin, first you need to create a C# class in your project and add the following code:
using Microsoft.TeamFoundation.Client;
using Microsoft.TeamFoundation.TestManagement.Client;
If you want to connect to the TFS Team Project with hard coded values, you can use the following code:
Uri tfsUri = new Uri("http:\\mytfsuri.com\teamCollection");
string teamProjectName = "TestProject";
TfsTeamProjectCollection myTfsTeamProjectCollection = new TfsTeamProjectCollection(tfsUri);
ITestManagementService service = _
(ITestManagementService)myTfsTeamProjectCollection.GetService(typeof(ITestManagementService));
ITestManagementTeamProject myTestManagementTeamProject = _
service.GetTeamProject(teamProjectName);
First, you initialize new URI object with your team collection URL which is equal to your TFS URL plus your team collection name. After that, you should create a collection object and initialize it with the URI. You use “GetService
” method to initialize the TFS service. Then, you can get the team project from it.
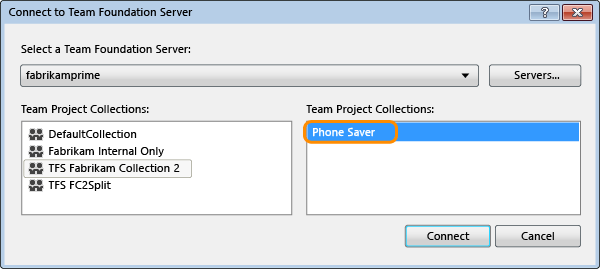
Collect TFS Settings with TeamProjectPicker Dialog
In order to capture the needed connection information, you can add TeamProjectPicker
object to your Windows app. You can use its method “ShowDialog
” in order to collect the information.
using (projectPicker)
{
var userSelected = projectPicker.ShowDialog();
if (userSelected == DialogResult.Cancel)
{
return;
}
if (projectPicker.SelectedTeamProjectCollection != null)
{
Uri tfsUri = = projectPicker.SelectedTeamProjectCollection.Uri;
string teamProjectName = projectPicker.SelectedProjects[0].Name;
TfsTeamProjectCollection myTfsTeamProjectCollection =
projectPicker.SelectedTeamProjectCollection;
ITestManagementService service =
(ITestManagementService)ExecutionContext.TfsTeamProjectCollection.GetService
(typeof(ITestManagementService));
ITestManagementTeamProject myTestManagementTeamProject = service.GetTeamProject(teamProjectName);
}
}
Dim tfsUri As New Uri(@"http:\\mytfsuri.com\teamCollection")
Dim teamProjectName As String = "TestProject"
Dim myTfsTeamProjectCollection As New TfsTeamProjectCollection(tfsUri)
Dim service As ITestManagementService =
DirectCast(myTfsTeamProjectCollection.GetService(GetType(ITestManagementService)), ITestManagementService)
Dim myTestManagementTeamProject As ITestManagementTeamProject = service.GetTeamProject(teamProjectName)
So Far in the TFS API Series
1. Connect to TFS Team Project C# Code
2. Manage TFS Test Plans C# Code
3. Manage TFS Test Cases C# Code
4. Manage TFS Test Suites C# Code
5. TFS Associate Automated Test with Test Case C# Code
6. Test Cases Statistics with SSRS and TFS Data Warehouse
7. SSRS SQL Server Reporting Services- Subscriptions for Reports
If you enjoy my publications, feel free to SUBSCRIBE
Also, hit these share buttons. Thank you!
Source Code
The post- Connect to TFS Team Project C# VB .NET Code appeared first on Automate The Planet.