As an ASP.NET developer, every one experiences the most prominent Gridview
. In this article, I'm going to couple all types of "highlighting" rows/column using jQuery.
Problem
You may need to achieve any one of the following:
- Highlight the row when mouse over on it in a grid view.
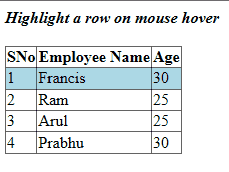
- Highlight the cell when mouse over on it:
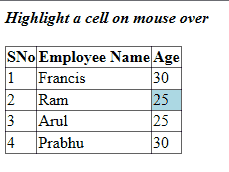
- Highlight a row of the
gridview
when click on the row.
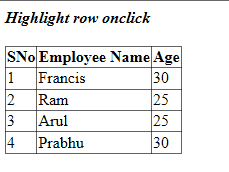
- Highlight a cell on a
gridview
when click on the cell.

Prerequisite
This tutorial rely on jQuery purely. So you need to include the jquery library on your solution. You can download jQuery from here.
Code
As a first step, we need some data to be filled in Gridview
. For this purpose, I have created a simple table "tblEmployee
" with some columns.
CREATE TABLE [dbo].[tblEmployee](
[EmpID] [int] NOT NULL,
[EmpName] [varchar](50) COLLATE SQL_Latin1_General_CP1_CI_AS NOT NULL,
[EmpAge] [int] NOT NULL,
CONSTRAINT [PK_tblEmp] PRIMARY KEY CLUSTERED
(
[EmpID] ASC
)WITH (IGNORE_DUP_KEY = OFF) ON [PRIMARY]
) ON [PRIMARY]
Use the below script to insert values into that table:
insert into tblEmployee values (1,'Francis',30);
insert into tblEmployee values (2,'Ram',25);
insert into tblEmployee values (3,'Arul',25);
insert into tblEmployee values (4,'Prabhu',30);
As a next step, create a webform and include a gridview into it as below:
<%@ Page Language="C#" AutoEventWireup="true" CodeBehind="GridviewDemo.aspx.cs"
Inherits="DemoWebApp.GridviewDemo" %>
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:GridView ID="gvEmployee" runat="server"
EmptyDataText="No Records Found" AutoGenerateColumns="false" >
<Columns>
<asp:BoundField HeaderText="SNo" DataField="EmpID" />
<asp:BoundField HeaderText="Employee Name" DataField="EmpName" />
<asp:BoundField HeaderText="Age" DataField="EmpAge" />
</Columns>
</asp:GridView>
</div>
</form>
</body>
</html>
The below code in the code-behind file is used to check the post-back and call the method to bind the data table to gridview
.
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
gvEmployee.DataSource = GetDataFromDB();
gvEmployee.DataBind();
}
}
The below function is used to get data from database and return a datatable
.
private DataTable GetDataFromDB()
{
DataTable dt = new DataTable();
string strConString = ConfigurationManager.ConnectionStrings["MyConString"].ToString();
using (SqlConnection con = new SqlConnection(strConString))
{
string strQuery = "Select * from tblEmployee";
con.Open();
SqlCommand cmd = new SqlCommand(strQuery, con);
SqlDataAdapter da = new SqlDataAdapter(cmd);
da.Fill(dt);
}
return dt;
}
We are going to define the following "style
" classes in the aspx file itself. These classes are going to be used in jQuery to highlight the row/cell.
<style type="text/css">
.selectedCell {
background-color: lightblue;
}
.unselectedCell {
background-color: white;
}
</style>
In the next step, I'm going to add the jQuery in the aspx file (between the <script>
and </script>
tag). The below jQuery snippet is used to highlight the row when mouse over on it.
$(document).ready(function () {
$('#gvEmployee tr').hover(function () {
$(this).addClass('selectedCell');
}, function () { $(this).removeClass('selectedCell'); });
});
Include the below jQuery code if you want to highlight the cell instead of row:
$(document).ready(function () {
$('#gvEmployee td').hover(function () {
$(this).addClass('selectedCell');
}, function () { $(this).removeClass('selectedCell'); });
});
The below jQuery is used to highlight the row when the particular row is clicked.
$(function () {
$(document).on('click', '#gvEmployee tr', function () {
$("#gvEmployee tr").removeClass('selectCell');
$(this).addClass('selectCell');
});
});
Include the below jQuery code if you want to highlight the cell when the particular cell is clicked.
$(function () {
$(document).on('click', '#gvEmployee tr', function () {
$("#gvEmployee tr").removeClass('selectCell');
$(this).addClass('selectCell');
});
});
Highlight Row/Cell Based On Some Condition
In some cases, you may want to highlight the row or cells based on some condition too. In that case, you can use "RowDataBound
" event of Gridview
. For example, I'm going to highlight the row based on the "age
" column value.
protected void gvEmployee_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow)
{
int age = Convert.ToInt32(e.Row.Cells[2].Text.ToString());
if (age >= 25 && age < 30)
{
e.Row.BackColor = Color.GreenYellow;
}
if (age == 30)
{
e.Row.BackColor = Color.LightBlue;
}
}
}
The output looks like below:
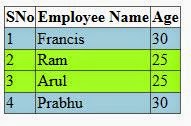
Highlight Rows on Databound
In another way, you may want to highlight the column alone while binding the data, the below code accomplishes this.
protected void gvEmployee_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.DataRow)
{
int age = Convert.ToInt32(e.Row.Cells[2].Text.ToString());
if (age >= 25 && age < 30)
{
e.Row.Cells[2].BackColor = Color.GreenYellow;
}
if (age == 30)
{
e.Row.Cells[2].BackColor = Color.LightBlue;
}
}
}
The above code output is like below:
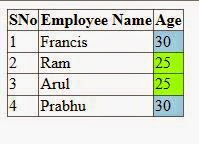
Highlight Column on Databound
If you need any clarifications, please let me know your thoughts as comments!