Introduction
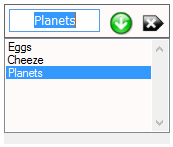
A list that doesn't drop down, and lets you add items without the risk of duplication. Hit Enter or click the Arrow to add, or click the black delete icon to delete.
It's not beautiful, but it fits a need in my programs.
Using the Code
This is not directly related to the topic, but is a point of interest:
The TableLayoutPanel
in the top area is a modified TableLayoutPanel
, that I found out there, that draws itself better than the original one. I use this modified TLP in all my projects now:
Public Class cExTLP
Inherits TableLayoutPanel
Private _components As System.ComponentModel.IContainer
Public Sub New()
Me.SetStyle(ControlStyles.AllPaintingInWmPaint, True)
Me.SetStyle(ControlStyles.OptimizedDoubleBuffer, True)
End Sub
Sub New(components As System.ComponentModel.IContainer)
_components = components
End Sub
End Class
If an item is left in the text area but not added to the list, you can detect that with the function.
Public Function ItemEnteredButNotAdded() As Boolean
If TB.Text = "" Then
Return False
ElseIf FindMyString(TB.Text) > ListBox.NoMatches Then
Return False
Else
Return True
End If
End Function
You can pass in a list of your items an ArrayList
:
Public Property A_ListBoxItems() As ArrayList
Get
If LB.Items IsNot Nothing Then
Return New ArrayList(LB.Items)
Else
Return Nothing
End If
End Get
Set(ByVal value As ArrayList)
If value IsNot Nothing AndAlso value.Count > 0 Then
For i As Integer = 0 To value.Count - 1
LB.Items.Add(value(i).ToString)
Next
End If
End Set
End Property
Or if you store some lists in your database as delimited string
s, you can pass in the items as a <font face="Courier New">Delimited String </font>
such as <font face="Courier New">*Eggs*Cheeze*Planets</font>
Public Property A_DelimitedItems(ByVal Delimeter As String) As String
Get
If LB.Items.Count = 0 Then
Return ""
Else
Dim i As Integer
Dim Item As String
Dim Out As String = ""
For i = 0 To LB.Items.Count - 1
Item = LB.Items(i).ToString
If Item <> "" Then Out += Item
If i < LB.Items.Count - 1 Then Out += Delimeter
Next
Return Out
End If
End Get
Set(ByVal value As String)
If value <> "" Then
If InStr(value, Delimeter) = 0 Then
LB.Items.Add(value)
Else
Dim Items() As String = Split(value, Delimeter)
If Items.Length > 0 Then
LB.Items.AddRange(Items)
End If
End If
End If
End Set
End Property
I originally wanted the Delete or Backspace keys to delete the current item from the list, but that would prevent using those keys to edit the text being entered, so in the end, you have to actually click the black delete button to delete the entry, which is better.
So this code that tries to detect backspace and delete - it doesn't work, as far as I can tell- haha.
Anyway, I left the code in to remind myself that it doesn't (seem to) work:
Private Sub LB_KeyDown(ByVal sender As Object, ByVal e As System.Windows.Forms.KeyEventArgs) _
Handles LB.KeyDown
If e.KeyCode = Keys.Delete OrElse e.KeyCode = Keys.Back Then
RemoveClick()
End If
End Sub
History