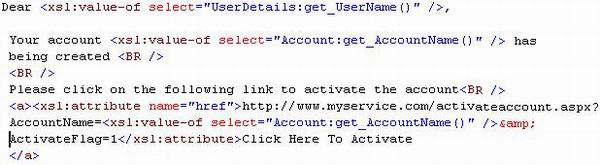
Introduction
This article uses an XSLT file as a template file to store email templates. It specifically targets on usage of Custom Objects Properties to be used in the XSLT file.
Background
Basic knowledge of XML, XPath and XSLT is needed apart from C# and ASP.NET.
Using the code
The Email.cs class in EmailProject can be segregated into various other components to make it as generic as possible. Also, the User.cs and Account.cs class files can be placed in a separate project containing the business objects for the application.
The following are the extracts from the email.cs file:
public static void SetUserDetails()
{
User myuser = new User();
myuser.UserName = "ABC";
myuser.EmailAddress = abc@someemail.com;
Account myaccount = new Account();
myaccount.AccountName = "ABC Account";
Hashtable objHash = new Hashtable();
objHash["ext:User"] = myuser;
objHash["ext:Account"] = myaccount;
SendEmail("xyz@email.com", "emailtemplate.xslt", objHash);
}
The SetUserDetails()
function sets the values of User
and Account
objects. These are objects sent to be used as arguments to the XSLT file. Hence they are added to a HashTable
object with the key name as the same as their respective xmlns
namespace identifiers in the XSLT file, as shown below.
="1.0"="UTF-8"
<xsl:stylesheet version="1.0" xmlns:xsl="http://www.w3.org/1999/XSL/Transform"
xmlns:UserDetails="ext:User"
xmlns:Account="ext:Account">
XslTransform objxslt = new XslTransform();
objxslt.Load(templatepath + xslttemplatename);
XmlDocument xmldoc = new XmlDocument();
xmldoc.AppendChild(xmldoc.CreateElement("DocumentRoot"));
XPathNavigator xpathnav = xmldoc.CreateNavigator();
XsltArgumentList xslarg = new XsltArgumentList();
if (objDictionary != null)
foreach (DictionaryEntry entry in objDictionary )
{
xslarg.AddExtensionObject(entry.Key.ToString(), entry.Value);
}
StringBuilder emailbuilder = new StringBuilder();
XmlTextWriter xmlwriter = new
XmlTextWriter(new System.IO.StringWriter(emailbuilder));
objxslt.Transform(xpathnav, xslarg, xmlwriter, null);
The above is the code snippet from SendMail
method. The arguments to the XslTransform
object are added by using the AddExtensionObject
method of XsltArgumentlist
object.
string subjecttext, bodytext;
XmlDocument xemaildoc = new XmlDocument();
xemaildoc.LoadXml(emailbuilder.ToString());
XmlNode titlenode = xemaildoc.SelectSingleNode("//title");
subjecttext = titlenode.InnerText;
XmlNode bodynode = xemaildoc.SelectSingleNode("//body");
bodytext = bodynode.InnerXml;
if (bodytext.Length > 0)
{
bodytext = bodytext.Replace("&","&");
}
SendEmail(emailto, subjecttext, bodytext);
The above code shows the title text of the XHTML doc to be used as the e-mail's subject and the body text to use used as the body section of the email. This is done by simple XPath.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.