Introduction
I have searched a lot of samples supporting synchronous and asynchronous serial communication, but the drawback is that I need to implement a thread or a timer to always keep reading the serial data, so I encapsulated this serial communication class with a thread to make it easy to handle the event (READ
/WRITE
/OPEN
/CLOSE
) of accessing serial port.
The SerialCtrlDemo
project illustrates how to use CSerialIO
class and is easy to present the serial communication event information as depicted in the following UI:
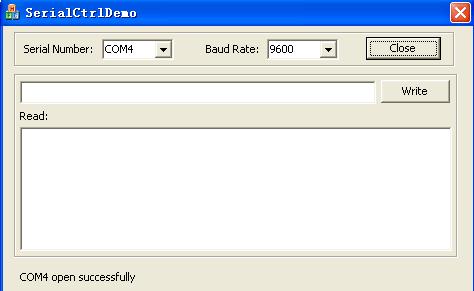
CSerialIO Class
The CSerialIO
class is defined for external API and the primary methods listed below:
class CSerialIO{
public:
CSerialIO();
virtual ~CSerialIO();
void OpenPort(CString strPortName,CString strBaudRate); virtual void OnEventOpen(BOOL bSuccess); void ClosePort();virtual void OnEventClose(BOOL bSuccess); virtual void OnEventRead(char *inPacket,int inLength); void Write(char *outPacket,int outLength); virtual void OnEventWrite(int nWritten); …
};
How to Use CSerialIO Class
Take the following steps to use the CSerialIO
class:
- Add the SerialCtrl.h & SerialCtrl.cpp files in your VC++ project.
- Add the line
#include "SerialCtrl.h"
in your dialog's header file. - Inherit the
CSerialIO
class in your dialog. - Handle the event (
READ
/WRITE
/OPEN
/CLOSE
) in your dialog class via overwrite the virtual function.
void CSerialCtrlDemoDlg::OnEventOpen(BOOL bSuccess)
{
CString str;
if (bSuccess)
{
str=m_strPortName+" open successfully";
bPortOpened=TRUE;
m_btnOpen.SetWindowText("Close");
}
else
{
str=m_strPortName+" open failed";
}
m_staticInfo.SetWindowText(str);
}
void CSerialCtrlDemoDlg::OnEventClose(BOOL bSuccess)
{
CString str;
if (bSuccess)
{
str=m_strPortName+" close successfully";
bPortOpened=FALSE;
m_btnOpen.SetWindowText("Open");
}
else
{
str=m_strPortName+" close failed";
}
m_staticInfo.SetWindowText(str);
}
void CSerialCtrlDemoDlg::OnEventRead(char *inPacket,int inLength)
{
m_listboxRead.AddString(inPacket);
CString str;
str.Format("%d bytes read",inLength);
m_staticInfo.SetWindowText(str);
}
void CSerialCtrlDemoDlg::OnEventWrite(int nWritten)
{
if (nWritten>0)
{
CString str;
str.Format("%d bytes written",nWritten);
m_staticInfo.SetWindowText(str);
}
else
{
m_staticInfo.SetWindowText("Write failed");
}
}
Note
This code has been verified with RS-232 connector, and you can easily customize the event handler in run function of SerialThread
class.
History
- 5th August, 2010: Initial post