Introduction
In modern web development, one of the key challenges is effectively managing data access while maintaining clean, modular code. ASP.NET MVC WebAPI provides a robust framework for building RESTful APIs, but integrating it with a data access layer can sometimes be complex. However, by leveraging class libraries and Entity Framework, we can streamline this process, making data access in our WebAPI projects efficient and maintainable.
In this article, we'll walk through the steps of setting up a class library for data access, integrating it with an ASP.NET MVC WebAPI project, and creating a simple API controller to fetch data from a database.
Setting up the DataAccessLayer Class Library
First, let's create a class library project named DataAccessLayer in our solution. Within this project, we'll define our data access logic using Entity Framework without the need for a .edmx file (ADO.NET).
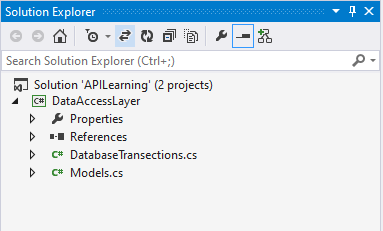
-
DatabaseTransections Class
Within the DataAccessLayer project, we'll create a class named DatabaseTransections
, inheriting from DbContext
. This class will contain methods for fetching data from the database using Entity Framework. By encapsulating database interactions within this class, we promote code reusability and maintainability.
public class DatabaseTransections : DbContext
{
public DatabaseTransections() : base("name=ConStr") { }
public virtual DbSet<ProductEntity> Product { get; set; }
protected override void OnModelCreating(DbModelBuilder modelBuilder)
{
modelBuilder.Entity<ProductEntity>().ToTable("tblProduct");
}
}
-
ProductEntity and ProductRepository Classes
Next, we'll define our entity class, ProductEntity
, to represent the data model for products in our database. Additionally, we'll create a ProductRepository
class to handle CRUD operations for products, abstracting away the database-specific logic.
public class ProductEntity
{
public int ID { get; set; }
public int ProductCategory { get; set; }
public string ProductName { get; set; }
public int UnitPrice { get; set; }
public int Quantity { get; set; }
}
public class ProductRepository
{
private readonly DatabaseTransections transection;
public ProductRepository(DatabaseTransections _transection)
{
transection = _transection;
}
public List<ProductEntity> GetALLProducts(int? _productCategory)
{
try
{
return transection.Product.Where(m => m.ProductCategory == _productCategory).ToList();
}
catch (Exception ex)
{
return null;
}
}
}
Integrating with the WebAPI Project
Now, let's create an ASP.NET MVC WebAPI project named APILearning in our solution. We'll add a WebAPI controller named ProductController
to expose endpoints for fetching product data. We'll add DataAccessLayer solution reference to access ProductRepository
Class in WebAPI controller.
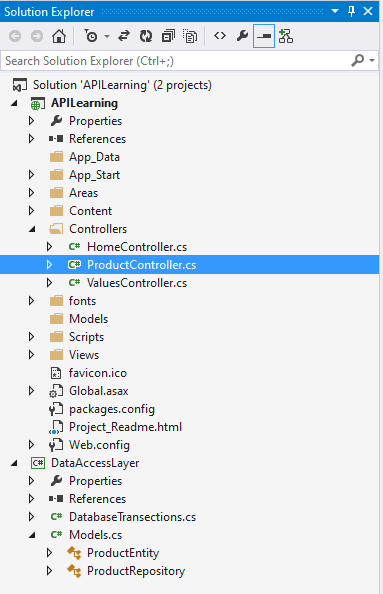
Implement controller actions (methods) that map to HTTP verbs (GET, POST, PUT, DELETE) to handle API requests. These actions will interact with the ProductRepository
to perform CRUD (Create, Read, Update, Delete) operations on product data.
public class ProductController : ApiController
{
public IEnumerable<ProductEntity> Get()
{
return new ProductRepository(new DatabaseTransections()).GetALLProducts(null);
}
}
Benefits of This Approach
- Separation of Concerns: The data access logic is encapsulated within the
DataAccessLayer
class library, promoting maintainability and reusability. - Testability: The use of dependency injection allows for easier unit testing of both the controller and the repository.
- Flexibility: The data access layer can be easily extended to support additional entities and data operations.
Conclusion
By organizing our project into separate class libraries for data access and API logic, we promote separation of concerns and maintainability. Leveraging Entity Framework simplifies database interactions, allowing us to focus on building robust APIs without worrying about low-level database operations.
In summary, by following the steps outlined in this article, developers can streamline data access in ASP.NET MVC WebAPI projects, making them more scalable and maintainable in the long run.
Results-driven Software Developer with 9 years of experience delivering innovative solutions. Managed, Developed and scaled numerous software applications from idea to finished product and beyond. A collaborative team player, adept at working closely with cross-functional teams to achieve project objectives. Demonstrated capability as a self-starter, consistently taking initiative and driving projects forward. Expertise in mission critical systems, agile methodologies, and creating robust software architectures. Committed to driving technological advancements and exceeding client expectations. Passionate about leveraging cutting-edge technologies to solve complex problems and deliver impactful software solutions.