Introduction
Sometimes, we want to get server side date-time for checking something in its own application, example check licence key, check time using of any program, and... anything. This tip might help you!
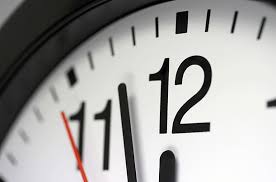
Background
I use SYSTEMTIME, HINTERNET, HttpQueryInfo, InternetOpenUrl, InternetOpen
API and must include WinInet.h.
#include <WinInet.h>
Using the Code
Using SYSTEMTIME
for storing datatime
when you get on server.
Systemtime Structure
typedef struct _SYSTEMTIME {
WORD wYear;
WORD wMonth;
WORD wDayOfWeek;
WORD wDay;
WORD wHour;
WORD wMinute;
WORD wSecond;
WORD wMilliseconds;
} SYSTEMTIME, *PSYSTEMTIME;
First, clear output buffer by using SecureZeroMemory
API.
SYSTEMTIME sysTime;
SecureZeroMemory(&sysTime, sizeof(SYSTEMTIME));
Next, open connection by using InternetOpen
API.
HINTERNET hInternetSession = InternetOpen(NULL, INTERNET_OPEN_TYPE_DIRECT, NULL, NULL, 0);
Get hInternetFile
by using InternetOpenUrl
.
HINTERNET hInternetFile = InternetOpenUrl(hInternetSession,
L"http://learn-tech-tips.blogspot.com", 0, 0,
INTERNET_FLAG_PRAGMA_NOCACHE | INTERNET_FLAG_NO_CACHE_WRITE, 0);
Okie, done. You can query datetime in server learn-tech-tips.blogspot.com now.
DWORD dwSize = sizeof(SYSTEMTIME);
if (!HttpQueryInfo(hInternetFile, HTTP_QUERY_DATE |
HTTP_QUERY_FLAG_SYSTEMTIME, &sysTime, &dwSize, NULL))
{
InternetCloseHandle(hInternetSession);
InternetCloseHandle(hInternetFile);
return sysTime;
}
Remember to clean up all resources when exiting the function, (I always forget it, hehe :), hope you don't do the same as me. :D)
InternetCloseHandle(hInternetFile);
InternetCloseHandle(hInternetSession);
Note: You can easily view the parameters below from MSDN.
Source Code
SYSTEMTIME CAutoPlayDlg::GetServerTime()
{
SYSTEMTIME sysTime;
SecureZeroMemory(&sysTime, sizeof(SYSTEMTIME));
HINTERNET hInternetSession = InternetOpen(NULL, INTERNET_OPEN_TYPE_DIRECT, NULL, NULL, 0);
if (!hInternetSession)
return sysTime;
HINTERNET hInternetFile = InternetOpenUrl(hInternetSession,
L"http://learn-tech-tips.blogspot.com", 0, 0,
INTERNET_FLAG_PRAGMA_NOCACHE | INTERNET_FLAG_NO_CACHE_WRITE, 0);
if (!hInternetFile)
{
InternetCloseHandle(hInternetSession);
return sysTime;
}
DWORD dwSize = sizeof(SYSTEMTIME);
if (!HttpQueryInfo(hInternetFile, HTTP_QUERY_DATE |
HTTP_QUERY_FLAG_SYSTEMTIME, &sysTime, &dwSize, NULL))
{
InternetCloseHandle(hInternetSession);
InternetCloseHandle(hInternetFile);
return sysTime;
}
InternetCloseHandle(hInternetFile);
InternetCloseHandle(hInternetSession);
return sysTime;
}
If you want to understand more information on this code, you can visit this link.
Points of Interest
I like coding, I like researching new technology.
My tech blog is http://learn-tech-tips.blogspot.com.
My blog sharing experiences about technology tips and trick include: language programming: C+, C#, ....
Design skills: photoshop, Office: Excel, Outlook and .... other things!
If you have any feedback about This topic , please leave your comment, we can discuss about it. 