Introduction
Some of you may have tried using the official guide of floodlight. And when you were trying to develop your own applications, some unexpected problem might pop out. Just like the following pictures.
This part of the code could be used to get all MAC addresses of the whole SDN network. And now it can also show the route information of the whole network.
And the final results are shown below:
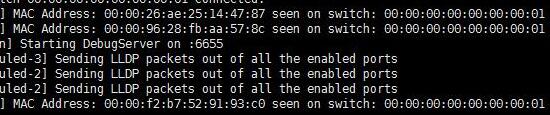
Using the Code
Step 1
Create a folder named "mactracker" in the path: floodlight/src/main/java/net/floodlightcontroller.
Step 2
Create a Java file named "MACTraker
" in the folder "mactraker".
Step3
Rebuilt floodlight controller. under the path : floodlight/. Using command "ant".
Step 4
Add "net.floodlightcontroller.mactracker.MACTracker
" to startUp mouldes profile
package net.floodlightcontroller.mactracker;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.ConcurrentSkipListSet;
import org.projectfloodlight.openflow.protocol.OFMessage;
import org.projectfloodlight.openflow.protocol.OFType;
import org.projectfloodlight.openflow.util.HexString;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import net.floodlightcontroller.core.FloodlightContext;
import net.floodlightcontroller.core.IFloodlightProviderService;
import net.floodlightcontroller.core.IOFMessageListener;
import net.floodlightcontroller.core.IOFSwitch;
import net.floodlightcontroller.core.module.FloodlightModuleContext;
import net.floodlightcontroller.core.module.FloodlightModuleException;
import net.floodlightcontroller.core.module.IFloodlightModule;
import net.floodlightcontroller.core.module.IFloodlightService;
import net.floodlightcontroller.packet.Ethernet;
public class <code>MACTracker</code> implements IOFMessageListener, IFloodlightModule {
protected IFloodlightProviderService <code>floodlightProvider</code>;
protected Set <code>macAddresses</code>;
protected static Logger <code>logger</code>;
@Override
public String getName() {
return MACTracker.class.getSimpleName();
}
@Override
public boolean isCallbackOrderingPrereq(OFType type, String name) {
return false;
}
@Override
public boolean isCallbackOrderingPostreq(OFType type, String name) {
return false;
}
@Override
public Collection<Class<? extends IFloodlightService>> getModuleServices() {
Collection<Class<? extends IFloodlightService>>
l = new ArrayList<Class<? extends IFloodlightService>>();
l.add(IFloodlightProviderService.class);
return null;
}
@Override
public Map<Class<? extends IFloodlightService>, IFloodlightService> getServiceImpls() {
return null;
}
@Override
public Collection<Class<? extends IFloodlightService>> getModuleDependencies() {
return null;
}
@Override
public void init(FloodlightModuleContext context) throws FloodlightModuleException {
floodlightProvider = context.getServiceImpl(IFloodlightProviderService.class);
macAddresses = new ConcurrentSkipListSet<Long>();
logger = LoggerFactory.getLogger(MACTracker.class);
}
@Override
public void startUp(FloodlightModuleContext context) throws FloodlightModuleException {
floodlightProvider.addOFMessageListener(OFType.PACKET_IN, this);
}
@Override
public net.floodlightcontroller.core.IListener.Command receive(IOFSwitch sw, OFMessage msg,
FloodlightContext cntx) {
Ethernet eth = IFloodlightProviderService.bcStore.get
(cntx,IFloodlightProviderService.CONTEXT_PI_PAYLOAD);
Long sourceMACHash = eth.getSourceMACAddress().toLong();
macAddresses.add(sourceMACHash);
logger.info("MAC Address: {} seen on switch: {}",
HexString.toHexString(sourceMACHash),
sw.getId());
logger.info("PcakageType: {} Rounte: {}",
eth.toString() ,
HexString.toHexString(eth.getSourceMACAddress())+"->>"+HexString.toHexString (eth.getDestinationMACAddress()));
return Command.CONTINUE;
}
}
Any Comments or Suggestions would be Embraced
Leave your messages and comments. Or send an email to jinwenqiang6668@gmail.com.