Let's see now how to create a simple game in CANVAS.
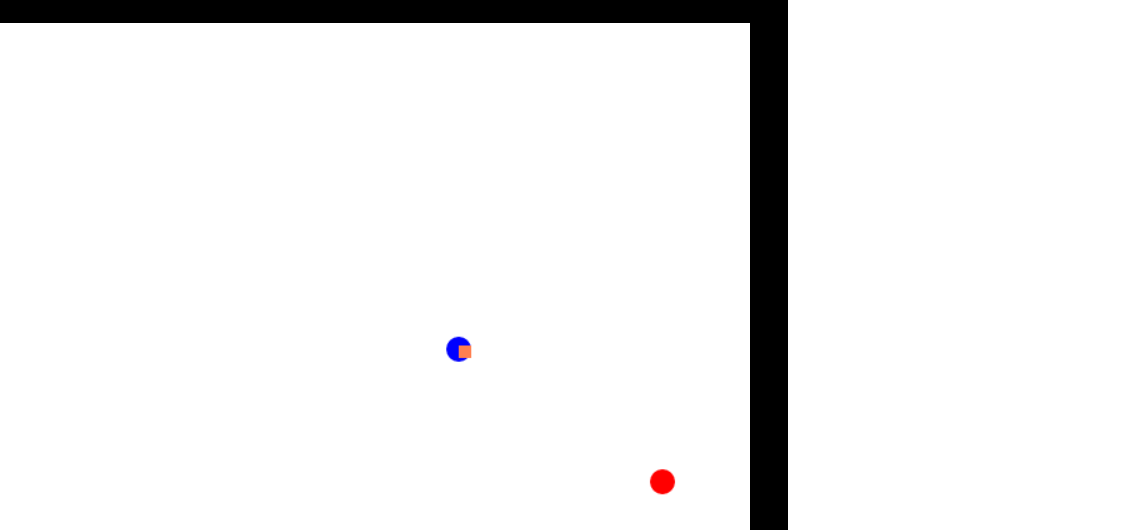
The game is basically a sort of "catch-up", in which you control the blue ball. The goal is to reach the red ball that moves erratically in the scene. For this, you click somewhere in the picture, the blue dot will go toward him.
The trick is to do this until the two balls intersect.
First, let's create a standard HTML document structure with the tag CANVAS:
<!DOCTYPE HTML>
<html lang="pt-br">
<head>
<meta charset="UTF-8">
<title>Simple game using CANVAS</title>
</head>
<h1>Simple game using CANVAS</h1>
<canvas id = "cont" height="600" width="600" >Your browser does not support canvas</canvas>
<body>
</html>
We instantiate in the script tag, the object responsible for generating animations in CANVAS. This should be done within the <head>
tag.
<script type="text/javascript">
window.onload = function(){
var element = document.getElementById("cont");
var context = element.getContext("2d");
if(context){
}
}
</script>
Now, let's create two classes:. Destiny
and Bol
. If you have no sense of direction with employee objects in Javascript, I suggest you visit the following documentation:
var Destiny = function(x,y){
this.x = x;
this.y = y;
this.w = 10;
this.h = 10;
this.update = function(x,y){
this.x = x;
this.y = y;
}
}
var destiny = new Destiny(10,10);
Well, returning to the subject, let's create another class, a class Bol
, defining their methods and attributes:
var Bol = function(x,y,r,vX,vY){
this.x = x;
this.y = y;
this.vX = vX;
this.vY = vY;
this.r = r;
this.dX = 0;
this.dY = 0;
this.move = 0;
this.sX = 1;
this.sY = 1;
this.update = function(){
this.x += this.vX;
this.y += this.vY;
}
this.checkColision = function(){
if((this.x + this.r) >= widthCanvas){
this.vX *= -1;
this.sX = -this.sX;
}else if((this.x - this.r) < 0){
this.vX *= -1;
this.sX = -this.sX;
}
if((this.y + this.r) >= heightCanvas){
this.vY *= -1;
this.sY = -this.sY;
}else if((this.y - this.r) < 0){
this.vY *= -1;
this.sY = -this.sY;
}
}
this.setDestinyX = function(x){
this.dX = x;
}
this.setDestinyY = function(y){
this.dY = y;
}
this.moveAuto = function(){
var x = returnEvenOdd();
if(x == true){
this.vX *= -1;
this.sX = -this.sX;
}else{
this.vY *= -1;
this.sY = -this.sY;
}
}
function returnEvenOdd(){
var one;
var check;
one = Math.floor( (Math.random()*6) + 1 );
check = (um % 2 == 0?true:false);
return check;
}
this.checkDestiny = function(){
if(this.move == 1){
if(this.sX == 1){
if(this.x > this.dX){
this.sX = -this.sX;
this.vX *= -1;
}
}else if(this.sX == -1){
if(this.x < this.dX){
this.sX = -this.sX;
this.vX *= -1;
}
}
if(this.sY == 1){
if(this.y > this.dY){
this.sY = -this.sY;
this.vY *= -1;
}
}else if(this.sY == -1){
if(this.y < this.dY){
this.sY = -this.sY;
this.vY *= -1;
}
}
if(( this.x > (this.dX-this.vX) && this.x <
(this.dX + this.vX) ) && ( this.y >
(this.dY-this.vY) && this.y < (this.dY + this.vY) )){
this.move = 0;
}
this.update();
}
}
}
Below, initialize some necessary variables to the game run.
var heightCanvas = element.height;
var widthCanvas = element.width;
var x = Math.floor((Math.random()*widthCanvas)+1);
var y = Math.floor((Math.random()*heightCanvas)+1);
var r = 10;
var vX = 5;
var vY = 5;
var bol = new Bol(x,y,r,vX,vY);
var blue = new Bol(Math.floor((Math.random()*widthCanvas)+1),
Math.floor((Math.random()*heightCanvas)+1),r,vX,vY);
var destiny = new Destiny(blue.x,blue.y);
var bols = new Array();
bols.push(bol);
bols.push(blue);
setInterval(function(){ bol.moveAuto(); },2000);
draw();
animate();
To understand how the method 'draw ()
' works, you must have a base knowledge as the 'context
' works.
For that, I recommend the following reading:
function draw(){
context.clearRect(0,0,widthCanvas,heightCanvas);
context.fillStyle="#FF0000";
context.beginPath();
context.moveTo(bol.x,bol.y);
context.arc(bol.x,bol.y,bol.r,0,Math.PI*2);
context.closePath();
context.fill();
context.fillStyle="#0000FF";
context.beginPath();
context.moveTo(blue.x,blue.y);
context.arc(blue.x,blue.y,blue.r,0,Math.PI*2);
context.closePath();
context.fill();
context.beginPath();
context.fillStyle = "#FF7F50";
context.fillRect(destiny.x,destiny.y,destiny.w,destiny.h);
context.fill();
}
The animate()
function is the control of the game.
It is the function that is performed within a certain range of time, to identify and define the status of the game:
function animate(){
bola.checkColision();
bola.update();
eu.checkColision();
eu.checkDestiny();
colision();
draw();
setTimeout(animate,30);
}
When you click somewhere in the CANVAS screen, the target of the blue ball changes, and it is redirected to the point where you did click.
element.onclick = function(e){
var pX;
var pY;
pX = e.pageX - this.offsetLeft;
pY = e.pageY - this.offsetTop;
eu.setDestinyX(pX);
eu.setDestinyY(pY);
eu.move = 1;
destino.update(pX,pY);
}
The function 'colision()
' is responsible for verifying whether there was a collision between the blue and the red ball.
function colision(){
for(var i = 0;i+1<bols.length;i++){
var j = i+1;
if( (
( bols[i].x + 2*bols[i].r) > bols[j].x
&&
(bols[i].x + 2*bols[i].r) < (2*bols[j].r + bols[j].x)
) &&
(
(bols[i].y + 2*bols[i].r) > bols[j].y
&&
(bols[i].y + 2*bols[i].r) < (2*bols[j].r + bols[j].y)
)
){
alert("Congratulations!!");
}
}
}
Link to download the game: https://sites.google.com/site/wagplugin/home/html5_cp.zip.
Github: https://github.com/wagnerwar/Jogos.