Introduction
I have not seen many tutorials on Web Api test using Frisby , most of the article available on the web starts with coding and writing your frisby tests. But here I has written this tutorial for explaining configuartion of the environment ,creating sample WebAPI using Visula Studio 2012 and writing frisby Tests.
Background
Frisby is a REST API testing framework built on node.js and Jasmine that makes
testing API endpoints easy, fast, and fun.
- Environement Setup
- Create Sample WebAPI for Test
- Create Frisby Tests for Sample Web API
Environment Setup
Install below
- NodeJs (do not install in default folder i.e. c:\Program File\, install folder like c:\NodeJs (Refer to https://nodejs.org/en/)
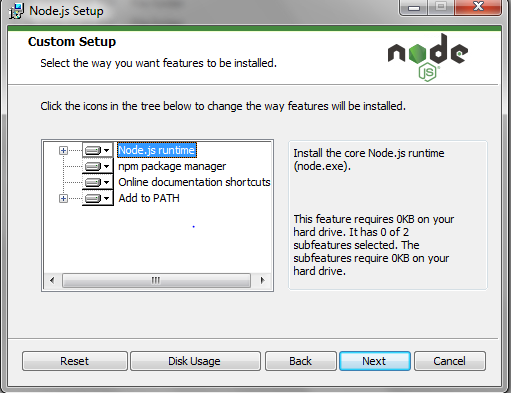
- Jasmine-Node (Refer to https://github.com/mhevery/jasmine-node)

- Frisby (Refer to http://frisbyjs.com/)

Create Sample WebAPI Service
Create a web API project using Visual Studio or download the attachment as zip and run the web API.
UserController
using FrsibyTest.Models;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
namespace FrsibyTest.Controllers
{
[Route("api/User")]
public class UserController : ApiController
{
List<userviewmodel> dataUserViewModel = new List<userviewmodel>
{
new UserViewModel{ Id=1, FirstName ="FirstName1" , LastName="LastName1"},
new UserViewModel{ Id=2, FirstName ="FirstName2" , LastName="LastName2"},
new UserViewModel{ Id=3, FirstName ="FirstName3" , LastName="LastName3"},
};
public IEnumerable<userviewmodel> Get()
{
return dataUserViewModel.ToList();
}
public UserViewModel Get(int id)
{
return dataUserViewModel.Where(x=>x.Id.Equals(id)).First();
}
[HttpPost]
public UserViewModel Post(UserViewModel userViewModel)
{
return dataUserViewModel.First();
}
[HttpPut]
public UserViewModel Put(int id, UserViewModel userViewModel)
{
var data = dataUserViewModel.Where(x => x.Id.Equals(id)).First();
data.FirstName = "Name-Updated" ;
return data;
}
[HttpDelete]
public string Delete(int id)
{
return "Deleted successfully";
}
}
}
View Model
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace FrsibyTest.Models
{
public class UserViewModel
{
public int Id { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
}
}
Verify Your Service is up and running before frisby tests.

Frisby Test
Write the Firsby Test in user_spec.js file:
var expectedGETMethodTypejson = [{
"Id": 0,
"FirstName": "string",
"LastName": "string"
}];
var exepectJSONTypeForSingleRecord = {
"Id": 0,
"FirstName": "string",
"LastName": "string"
};
var expectedJson = [
{
"Id": 1,
"FirstName": "FirstName1",
"LastName": "LastName1"
},
{
"Id": 2,
"FirstName": "FirstName2",
"LastName": "LastName2"
},
{
"Id": 3,
"FirstName": "FirstName3",
"LastName": "LastName3"
}
];
var frisby = require('frisby');
frisby.globalSetup({
request: {
headers: { 'Accept': 'application/json' },
timeout: (30 * 1000)
}
});
frisby.create('Ensure user service is up and running')
.get('http://localhost:1623/api/User')
.expectStatus(200)
.toss();
frisby.create('Ensure response has a proper JSON Content-Type header')
.get('http://localhost:1623/api/User')
.expectHeaderContains('Content-Type', 'application/json')
.toss();
frisby.create('Ensure response has proper JSON types in specified keys')
.get('http://localhost:1623/api/User')
.expectJSONTypes(expectedGETMethodTypejson)
.toss()
frisby.create('Ensure response has proper JSON data')
.get('http://localhost:1623/api/User')
.expectJSONLength(3)
.expectJSON(expectedJson)
.toss()
frisby.create('Ensure response has proper JSON types in specified keys')
.post('http://localhost:1623/api/User', {
"Id": 8,
"FirstName": "FirstName8",
"LastName": "LastName8"
})
.expectStatus(200)
.expectJSONTypes(exepectJSONTypeForSingleRecord)
.expectJSONLength(3)
.toss()
frisby.create('Ensure response has proper JSON types in specified keys for update')
.put('http://localhost:1623/api/User/1', {
"Id": 1,
"FirstName": "Name-Updated",
"LastName": "LastName1"
})
.expectStatus(200)
.expectJSONTypes(exepectJSONTypeForSingleRecord)
.expectJSONLength(3)
.toss()
frisby.create('Ensure response has proper JSON types in specified keys for update')
.delete('http://localhost:1623/api/User/1')
.expectStatus(200)
.toss()
Save the above file in the folder:
C:\NODEJS\node_modules\frisby\examples
Run the Service and Then Run the Test As Below
C:\NODEJS>jasmine-node \node_modules\frisby\examples\user_spec.js

Create Report of Test Result using Junitreport

Points of Interest
REST API/Web API Automation test using frisby JavaScript.
History
- 12th September, 2016: Initial version