Introduction
A provider is a configurable service in AngularJS. The article illustrates how a Provider
looks like in simple scenarios like returning a string
, returning an object, returning an object having a function, etc. This article will help folks with little JavaScript knowledge that comes to learn AngularJS.
By the end of the article, the purpose of a Provider in AngularJS is detailed with a simple example.
Using the Code
The below HTML, index.html is used for all examples given below:
<html>
<head>
<title>Provider Demo</title>
</head>
<body ng-app="mainApp">
<div ng-controller="myController">
{{greetMessage}}
</div>
<script type="text/javascript"
src="https://code.angularjs.org/1.5.0/angular.min.js"></script>
<script type="text/javascript" src="app.js"></script>
</body>
</html>
a) Provider returns a simple string
. Below is a very simple example of a provider that returns a string
value, “Hello World
”.
var application = angular.module('mainApp', []);
application.provider('message', function() {
this.$get = function() {
return "Hello World!";
};
});
application.controller('myController', function($scope, message) {
$scope.greetMessage = message;
});
Here, message
is a provider and it has a method, this.$get
. When you call the provider, what is returned from the provider is what is returned from the this.$get
method. As such “Hello World!
” which is returned from this.$get
is returned when message provider is accessed. This is possible as the provider returns a string
.
Below is the output when the index.html is rendered in the browser.
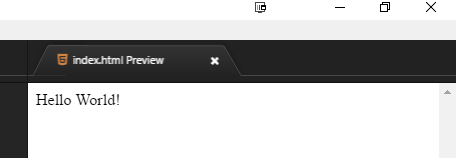
b) Provider returns a simple object.
var application = angular.module('mainApp', []);
application.provider('message', function() {
this.$get = function() {
return {
messageFromProvider: "Hello World!"
}
};
});
application.controller('myController', function($scope, message) {
$scope.greetMessage = message.messageFromProvider;
});
If the same provider needs to return an object, the below anonymous object is returned from inside the this.$get
method.
return {
messageFromProvider: "Hello World!"
}
Here, message
is a provider and it has a method, this.$get
. When you call the provider, what is returned from the provider is an object. The object has a property, messageFromProvider
. The program accessed the property using ProviderName.property
to render the output “Hello World!
”.
c) Provider returns an object having a method as one of its members:
var application = angular.module('mainApp', []);
application.provider('message', function() {
this.$get = function() {
return {
messageFromProvider: "Hello World!",
DisplayMessage: function() {
return this.messageFromProvider;
}
}
};
});
application.controller('myController', function($scope, message) {
$scope.greetMessage = message.DisplayMessage();
});
Here also, when you call the provider, what is returned from the provider is an object. The object has a method, DisplayMessage
apart from the property messageFromProvider
. The program invoked the method using ProviderName.Method()
to render the output “Hello World!
”. This example is the same as the above example but this illustrates how to invoke a method inside an object.
Why Provider
Using provider, you can supply data from config file. Below is a simple example where a custom message is being provided from config.
var application = angular.module('mainApp', []);
application.provider('message', function() {
var temp = null;
this.messageFromConfig = function(value) {
temp = value;
},
this.$get = function() {
return {
messageFromProvider: temp
}
}
});
application.config(function(messageProvider) {
messageProvider.messageFromConfig ("Hello World!")
});
application.controller('myController', function($scope, message) {
$scope.greetMessage = message.messageFromProvider;
});
So to supply a custom message to the provider from config file, a function having the Provider
as argument is supplied to application.config
function.
application.config(function(messageProvider) {
messageProvider.messageFromConfig = "Hello World!"
});
The custom value is set to Provider.PropertyName
which in this case is messageProvider.messageFromConfig = "Hello World!"
This is where a provider differs from factory and service. You can configure it from the application.config.