Introduction
This is a simple jQuery plug in to paginate the large HTML table that you can specify the number of rows, custom table head and can select columns to show per page. It is compatible with the set of PHP and MySQLi database server.
Using the plug in
- Download jrPaginator plug in.
- Add jrPaginator.min.css & jrPaginator.min.js to your project.
- Edit jrPaginationHandler.php (set the database details) and place it at your preferred location.
- Initialize the
jrPaginate
for the table you want to paginate as below:
$(document).ready(function(){
$('#yourTableId').jrPaginate({
dbTableName: 'yourDbTableName',
filePath: 'pathOfPHPFile'
});
});
Example:
$(document).ready(function(){
$('#contact-list').jrPaginate({
dbTableName: 'contact_list',
filePath: 'assets/user/contacts/jrPaginationHandler.php'
});
});
- Parameters
-
dbTableName Compulsory
Name of the mysql database table.
Example: -
dbTableName: 'contact_list'
-
filePath Compulsory
Path of jrPaginationHandler.php, you can place it at your preferred location. You can also change the file name or you can copy paste the code from this file to another PHP file, just care the $link
variable which holds the mysqli connection. Example: -
filePath: 'assets/user/contacts/jrPaginationHandler.php<sup><sub>?</sub></sup>
-
tableHead
Provide your own custom table head row. Example: -
tableHead : '<tr><th>Id</th><th>Name</th><th>Email</th><th>Contact</th><th>Address</th></tr>'
-
columnList
List of columns you want to fetch from the database table. -
columnList: {a: 'id', b: 'name', c: 'contact', d: 'email'}
-
listLength
Length of list, i.e., number of rows you want to display at a time in the table: -
listLength: 15
-
showPageBtn
Number of page buttons you want to display at a time: -
showPageBtn: 4
-
showLastPage
User cannot see the number of total pages if set to 'false
': -
showLastPage: false
-
rowAddOn
This parameter can be used to add any new common data column in the rows that is not in the database table, such as delete or edit button. -
rowAddOn: '<td><input type="button" value="Delete" onclick="delete(this);"></td>'
- Full Fledged Example
$(document).ready(function(){
$('#entity-list').jrPaginate({
dbTableName : 'pagination_test',
filePath : 'src/jrPaginationHandler.php',
listLength : 5,
showPageBtn : 4,
rowAddOn : '<td><input type='button'
value='delete'></td>',
tableHead : '<tr><th>id</th>
<th>name</th><th>contact</th>
<th>email</th></tr>',
columnList : {a : 'id', b : 'name',
c : 'contact', d : 'email'},
showLastPage: true
});
});
Points to Remember
- Don't forget to add jQuery library
- It is mandatory to give a unique 'id' to each HTML table
Demo
Visit the demo page to see the live demo of jrPaginator.
Snapshots
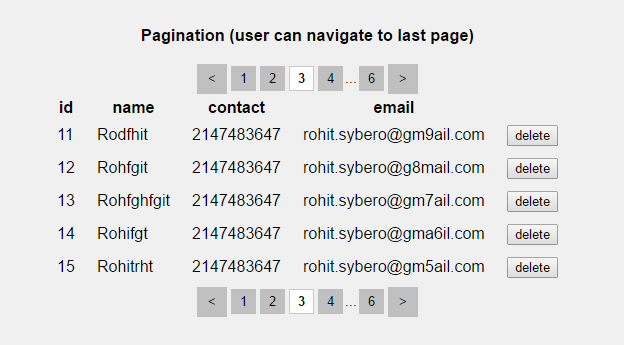

More Tips
The design of buttons can be customised using the below classes:
-
jr-top-btn
To edit the numbered buttons
-
jr-scroll-btn-left & jr-scroll-btn-left
To edit the left and right buttons
-
jr-focus
To edit the current page button
If you have variable table names for database table (Example: Each user has a different table name for the activity log), you can edit jrPaginationHandler.php.
Example:
$tableName=$_POST['dbTableName'];
$tableName= 'yourTableName';