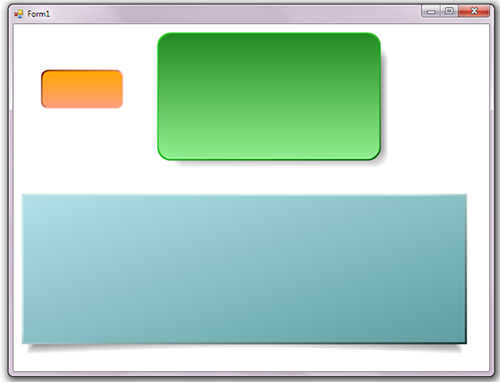
Introduction
By applying bevels, you can add depth to flat objects, it simulates the nicety of a real beveled shape. This article briefly explains how to design and implement the ability to paint the bevel onto a panel and how to apply the soft shadow for this control.
Background
The bevel effect is the simulation of two layers. The bottom layer should contain the shape, which will be split diagonally. Each half has to be filled with light and dark color. This will create edges effect. The upper level should create shape with the smaller size for the panel main area. The pictures below give detailed explanation.

Implementation
The bottom shape in the given control was drawn as a rounded rectangle with linear gradient filling. To split the area by diagonal, component uses Blend
function.
private void DrawEdges(Graphics g, ref Rectangle edgeRect)
{
Rectangle lgbRect = edgeRect;
lgbRect.Inflate(1, 1);
var edgeBlend = new Blend();
switch (Style)
{
case BevelStyle.Lowered:
edgeBlend.Positions = new float[] { 0.0f, .49f, .52f, 1.0f };
edgeBlend.Factors = new float[] { .0f, .6f, .99f, 1f };
break;
case BevelStyle.Raised:
edgeBlend.Positions = new float[] { 0.0f, .45f, .51f, 1.0f };
edgeBlend.Factors = new float[] { .0f, .0f, .2f, 1f };
break;
}
using (var edgeBrush = new LinearGradientBrush(lgbRect,
edgeColor1,
edgeColor2,
LinearGradientMode.ForwardDiagonal))
{
edgeBrush.Blend = edgeBlend;
RoundedRectangle.DrawFilledRoundedRectangle(g, edgeBrush, edgeRect, _rectRadius);
}
}
The top shape is the simple rounded rectangle.
private void DrawPanelStyled(Graphics g, Rectangle rect)
{
using (Brush pgb = new LinearGradientBrush(rect, _startColor, _endColor,
(LinearGradientMode)this.BackgroundGradientMode))
{
RoundedRectangle.DrawFilledRoundedRectangle(g, pgb, rect, _rectRadius);
}
}
The basic idea for the soft shadow effect was described in this article.
This control uses PathGradientBrush
with transparent outline color.
using (PathGradientBrush shadowBrush = new PathGradientBrush(path))
{
shadowBrush.CenterPoint = new PointF(rect.Width / 2,
rect.Height / 2);
Color[] color = { Color.Transparent };
shadowBrush.SurroundColors = color;
shadowBrush.CenterColor = _shadowColor;
graphics.FillPath(shadowBrush, path);
shadowBrush.FocusScales = new PointF(0.95f, 0.85f);
graphics.FillPath(shadowBrush, path);
}
The described technique gives control with the following features:
- Bevel Effect
- Rounded corners
- Gradients
- Soft shadow
- Edge thickness
- Shadow depth
History
- 8th August, 2017: Initial post