Introduction
I did it on the job, for my own need and I am just sharing it, should someone find it useful.
Background
Of course, there are plenty of plugins doing web controls validation. This plug in doesn't validate, but just enables or disables a control reading one of its attributes, and basing on the status of the referenced elements.
Using the Code
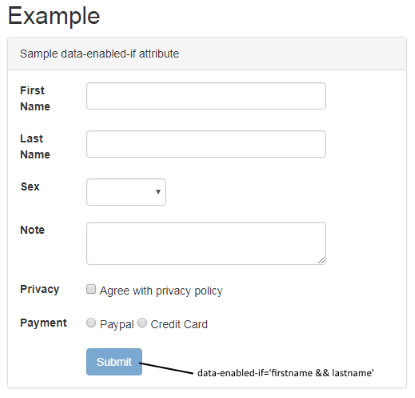
This is an example:
<input type='button' data-enabled-if='firstname && lastname' value='Submit' />
It just will check if elements having IDs firstname
and lastname
have both contents. In the attached example, they are two textbox
es.
Of course, you can combine elements ID in a logical expression, so you could write:
<input type='button' data-enabled-if='firstname && lastname && (privacy||payment ) ' value='Submit' />
In this case, privacy
is a checkbox
, and payment
is a name of a radiobutton
s group. One of them must be checked for the button to be enabled (provided that firstname
and lastname
are not empty).
The below statement is also accepted:
<input type='button' data-enabled-if='firstname && lastname && ! privacy' value='Submit' />
where the button is enabled only if firstname
and lastname
have content, and privacy
is NOT checked.
Points of Interest
The values in the data-enabled-if
attribute are searched mainly as ID
. If a value is not found as an ID
, it's searched as a name
for radiobutton
s group. Find below the behaviour for different type of elements.
TextBox | Return true if it has content |
TextArea | Return true if it has content |
CheckBox | Return true if it is checked |
Select | Return true if an item is selected |
RadioButton (name) | Return true if an item is checked |
Activate the plug in by:
$(document).ready(function(){ $(".container").enabledIf(); });
plug in code follows:
(function ($) {
$.fn.enabledIf = function () {
this.each(function () {
$("[data-enabled-if]").each(function () {
if ($(this).attr("id")==undefined)
{$(this).attr("id","obj-"+new Date().getTime());}
var att = ($(this).attr("data-enabled-if"));
var mod = att.replace(new RegExp("[&|()!]", "g"), ' ');
var r = mod.split(' ');
r = r.filter(function (entry) { return entry.trim() != ''; });
for (var i = 0; i < r.length; i++) {
var $elements = [];
if ($("#" + r[i]).length > 0)
$elements.push($("#" + r[i]));
else {
$("input:radio[name='" + r[i] + "']").each(function () {
$elements.push($(this));
});
}
for (var k = 0; k < $elements.length; k++) {
var $element = $($elements[k]);
$element.attr("data-enabling", $(this).attr("id"));
$element.attr("data-enable-group", att);
$element.on('input propertychange paste change', function () {
var logicalExpr = $(this).attr("data-enable-group");
for (var k = 0; k < r.length; k++) {
var v = isElementVal(r[k]);
var reg = new RegExp(r[k], "g");
logicalExpr = logicalExpr.replace(reg, v);
}
if (eval(logicalExpr) == true) {
$("#" + $(this).attr("data-enabling")).removeAttr("disabled");
}
else {
$("#" + $(this).attr("data-enabling")).attr
("disabled", "disabled");
}
});
$element.change();
}
}
});
});
return this;
};
function isElementVal(id) {
var el = $("#" + id);
if (el.length == 0)
return $("input:radio[name='" + id + "']").is(":checked");
if ($(el).is("input[type=checkbox]")) {
return $(el).prop("checked");
}
else{
return $.trim($("#" + id).val()) != "";
}
}
}(jQuery));
As a limitation, please note that an element can be used only once for the time being. That is, if an element ID is in a data-enabled-if
attribute, it cannot also be in another.
Author
Max Aronica is a senior .NET full-stack Developer working as external consultant for enterprise customers in Rome, Italy.
History
- 19/10/2017: First release