Introduction
Sometimes, you want to schedule a task in .NET Framework...
For example, you want to run a code every night at 01:00 AM for creating reports, calling a service, sending a message, checking some flows, etc.
For such work, someone creates a batch file and runs it with windows scheduled task, but in some situation, it won't work correctly,
Someone creates a timer for checking the local time and runs their task at a specific time, but it does not make good performance.
In .NET Framework , you can use System.Management.ManagementEventWatcher
library for watching on local time and raise an event at the desired time.
Using the Code
First of all, you must add reference to System.Managment.dll.
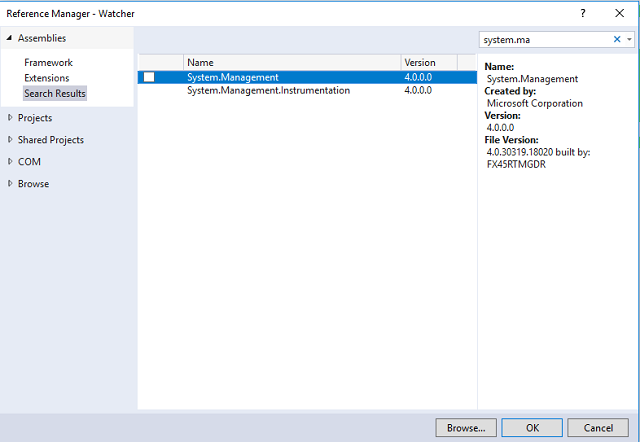
We should create an instance of System.Management.WqlEventQuery
and set the desired time on query.
In this example, I set query to run every day at 10:59:59 AM.
System.Management.WqlEventQuery query = new System.Management.WqlEventQuery_
("__InstanceModificationEvent", new System.TimeSpan(0, 0, 1), _
"TargetInstance isa 'Win32_LocalTime' AND TargetInstance.Hour=10 AND _
TargetInstance.Minute=59 AND TargetInstance.Second=59");
Create an instance of System.Management.ManagementEventWatcher
and pass your query in constructor:
System.Management.ManagementEventWatcher watcher = new System.Management.ManagementEventWatcher(query);
Now, it's time to connect event handles method to event, it will connect to onEventArrived
method.
watcher.EventArrived += new System.Management.EventArrivedEventHandler
(new System.Management.EventArrivedEventHandler(OnEventArrived));
And start the watcher
:
watcher.Start();
Finally, create onEventArrived
method, this method will run every day at the desired time (in this sample, 10:59:59 AM).
public static void OnEventArrived(object sender, System.Management.EventArrivedEventArgs e)
{
}
This is all code at a glance in Console Application.
static void Main(string[] args)
{
System.Management.WqlEventQuery query = new System.Management.WqlEventQuery_
("__InstanceModificationEvent", new System.TimeSpan(0, 0, 1), _
"TargetInstance isa 'Win32_LocalTime' AND TargetInstance.Hour=10 AND _
TargetInstance.Minute=59 AND TargetInstance.Second=59");
System.Management.ManagementEventWatcher watcher = _
new System.Management.ManagementEventWatcher(query);
watcher.EventArrived += new System.Management.EventArrivedEventHandler_
(new System.Management.EventArrivedEventHandler(OnEventArrived));
watcher.Start();
System.Console.ReadLine();
}
public static void OnEventArrived(object sender, System.Management.EventArrivedEventArgs e)
{
System.Console.WriteLine("Event Arrived");
}
I hope you'll like this code and use it.
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.