Introduction
I recently tried to lookup information on the Linq GroupBy
, and found that it was very poorly explained. I hope that this tip helps people trying to use GroupBy
.
Here is how GroupBy
is defined in MSDN:
public static IEnumerable<IGrouping<TKey, TElement>> <code>GroupBy</code><TSource, TKey, TElement>(
this IEnumerable<TSource> source,
Func<TSource, TKey> keySelector,
Func<TSource, TElement> elementSelector
)
Not very helpful, is it?
The Classes Used for Sample
For this tip, two class types are used. The main class, Department
, has an IEnumerable
property Items
, which is of type Item
. I did this because this is a complex case, and I think it provides better visibility on how to deal with more complex cases than a simple single level hierarchy:
public class Department
{
public string Name { get; set; }
public string DepartmentType { get; set; }
public List<Item> Items { get; set; }
}
public class Item
{
public string Name { get; set; }
public string ItemType { get; set; }
}
Different Ways to Skin the Rabbit
The following all provide the same result, but do them in different ways using the GroupBy
to obtain the same result:
var results = departments
.GroupBy(o => o.DepartmentType)
.Select(x => new
{
Type = x.Key,
ItemTypes = x.SelectMany(items => items.Items)
.Select(item => item.ItemType).Distinct().OrderBy(k => k).ToList()
});
var results = departments
.GroupBy(o => o.DepartmentType, o => o)
.Select(x => new
{
Type = x.Key,
ItemTypes = x.SelectMany(items => items.Items)
.Select(item => item.ItemType).Distinct().OrderBy(k => k).ToList()
});
var results = departments
.GroupBy(o => o.DepartmentType, o => o.Items)
.Select(x => new
{
Type = x.Key,
ItemTypes = x.SelectMany(items => items)
.Select(item => item.ItemType).Distinct().OrderBy(k => k).ToList()
});
var results = departments.GroupBy(o => o.DepartmentType, o => o, (key, g) => new
{
Type = key,
ItemTypes = g.SelectMany(items => items.Items)
.Select(item => item.ItemType).Distinct().OrderBy(k => k).ToList()
});
var result1 = departments.GroupBy(o => o.DepartmentType, o => o.Items, (key, g) => new
{
Type = key,
ItemTypes = g.SelectMany(item => item)
.Select(item => item.ItemType).Distinct().OrderBy(k => k).ToList()
});
Hopefully, the code is self-explanatory.
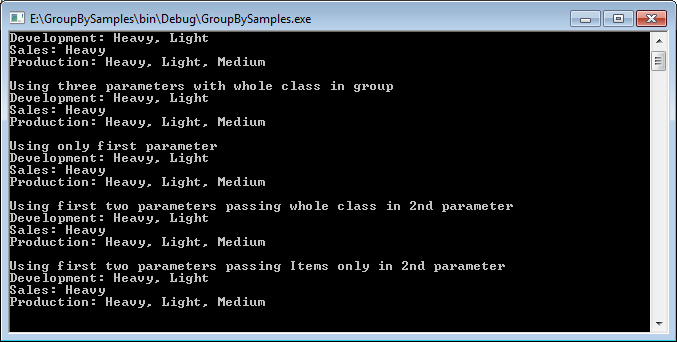
Notes:
If you have any suggestions on how this could be improved, please contact me. There are a several people that have not given this 5 stars and would like to know how I could make it more useful.
Conclusion
Hopefully, these samples will quickly help you in understanding the GroupBy
. I looked at a bunch of tips, samples, and articles that tried to explain the GroupBy
. Hopefully, this will be more useful.
History
- 2018-05-17: Initial version