Introduction
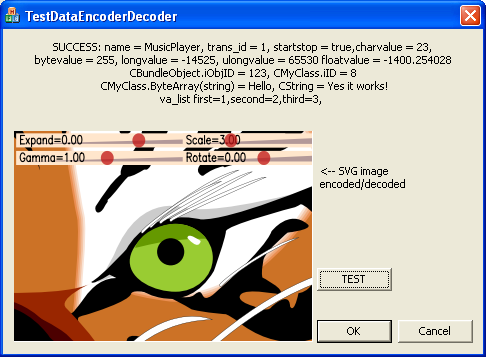
The code is a Data Encoder / Decoder library. It makes inter application communication easier, by providing a way to encode and decode a message based on a user defined protocol.
It also makes a simple compression of the "message" created by the encoder part. The decoder part will then decompress and decode message, based on the provided protocol
Background
It is using a simplified version of ASN (Abstract Syntax Notation) to encode / decode the data, and the compression algorithm is based on a simplified version of Hoffman coding
Using the code
The DataEncoderDecoder
is a standard static library and should be added to your project as a header file and a library file - the library is static which means that it will be embedded in your projects executable
Example: Creating an event message to be send to a server asking it to use method MusicPlayer
passing on a transaction id and action Start/Stop.
All data is in pCompressedData
!!! It is this memory that should be send:
DED_START_ENCODER(encoder_ptr);
DED_PUT_STRUCT_START( encoder_ptr, "event" );
DED_PUT_METHOD ( encoder_ptr, "name", "MusicPlayer" );
DED_PUT_USHORT ( encoder_ptr, "trans_id", trans_id);
DED_PUT_BOOL ( encoder_ptr, "startstop", action );
DED_PUT_STRUCT_END( encoder_ptr, "event" );
DED_GET_ENCODED_DATA(encoder_ptr,data_ptr,iLengthOfTotalData,pCompressedData,sizeofCompressedData);
CString strName,strValue;
unsigned short iValue;
bool bValue;
DED_PUT_DATA_IN_DECODER(decoder_ptr,pCompressedData,sizeofCompressedData);
if( DED_GET_STRUCT_START( decoder_ptr, "event" ) &&
DED_GET_METHOD ( decoder_ptr, "name", strValue ) &&
DED_GET_USHORT ( decoder_ptr, "trans_id", iValue) &&
DED_GET_BOOL ( decoder_ptr, "startstop", bValue ) &&
DED_GET_STRUCT_END( decoder_ptr, "event" ))
{
TRACE0(_T("FAIL!!!\n"));
}
else
{
TRACE0(_T("SUCCESS!!!\n"));
}
Points of Interest
When trying to use this type of data encoder / decoder, then remember from which type of computer you are sending / receiving, I am thinking about the Big/Little
Endian principle - so far the DataEncoderDecoder
does not take care of this (perhaps a smart reader could add this)
