Introduction
Have you ever wanted to overcome integer boundaries? If at least one time had you thought of multiplying or dividing a million digit by another, you're welcome. You're at the right place. You will make easy use of the TMember
type: a Member object.
Code Usage
m1 = TMember.FromString(txtTerm1.Text, new TRadix(10));
m2 = TMember.FromString(txtTerm2.Text, new TRadix(10));
uint n = 543215;
TMember m3 = TMember.FromInt(n, new TRadix(10));
uint[] data1 = new uint[456];
uint[] data2 = new uint[456];
for (int i = 0; i < 456; i++)
{
data1[i] = (uint)(i * i);
data2[i] = (uint)(i * i%17);
}
m1 = TMember.FromData(data1, new TRadix(uint.MaxValue));
m2 = TMember.FromData(data2, new TRadix(uint.MaxValue));
TMember mhex = TMember.FromString("4321543254362", new TRadix(16));
TMember mbinary = mhex.ToDecimal().DecimalToRadix(new TRadix(2));
public static TMember operator +(TMember term1, TMember term2)
public static TMember operator +(TMember term1, TDigit term2)
public static TMember operator -(TMember term1, TMember term2)
public static TMember operator -(TMember term1, TDigit term2)
public static TMember operator *(TMember term1, TMember term2)
public static TMember operator *(TMember term1, TDigit term2)
public static TMember operator /(TMember term1, TMember term2)
public static TMember operator /(TMember term1, TDigit term2)
public static TMember operator %(TMember term1, TMember term2)
public static TMember operator %(TMember term1, TDigit term2)
public static TMember operator <(TMember term1, TMember term2)
public static TMember operator <(TMember term1, TDigit term2)
public static TMember operator >(TMember term1, TMember term2)
public static TMember operator >(TMember term1, TDigit term2)
public static TMember operator <=(TMember term1, TMember term2)
public static TMember operator <=(TMember term1, TDigit term2)
public static TMember operator >=(TMember term1, TMember term2)
public static TMember operator >=(TMember term1, TDigit term2)
public static TMember operator ==(TMember term1, TMember term2)
public static TMember operator ==(TMember term1, TDigit term2)
txtResult.Text = "" + (m1 * m2) % TMember.FromString("54366");
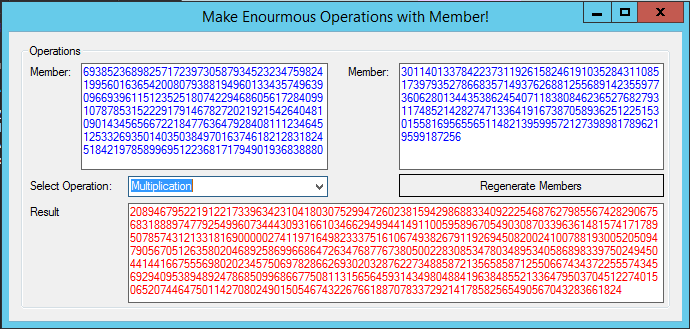
- It is so easy to initialize a Member object:
- Now you can initialize your Member instance with various radixes, like this one:
- You can also make conversion of different radixes to or from decimal using the
ToDecimal
and DecimalToRadix
methods. They're public now: - I implemented nearly all operators valid for integers:
- And you can make use of it:
- The result is simple to see; not so easy to evaluate, however:
Architecture

return SCalculator.Comparer.Gt(term1, term2);
uint n = 3;
n = SValidator<uint>.Validate(num => num > 10, n);
numstr=SValidator<string>.Validate(s => s.All<char>(ch => Char.IsNumber(ch)), numstr);
I love it 

- The code architecture could be summarized as below:
- In the
Arithmetics
namespace, the SCalculator
class is the transition layer to the outside of the namespace. It initializes all TAdder
, TComparer
etc. classes as static object instances and Types
classes make use of them like this: - As you can see, this code compares two values if
term1
> term2
and returns the result. - The
Types
namespace has these core types for Member:
TMember
: main entry class TDigit
: represents one digit of a number (with any radix) TRadix
: represents radix for digit and member ESigns
: for now, this enumeration is orphan
- The
Constraints
namespace is my favorite. Imagine a validator of any type making checks and returning the checked type in one line of code: - This line of code validates n if it is greater than or equal to (>=) 0 (now that is false because n=3). If not, it throws an exception. And here is a some more complicated example (trick: you can check if the string has only decimal digits):
- There is also another class
TSelfValidator<TType>
to validate derived classes, but that's enough explanation. If you're still with me, I appreciate your patience. Don't get afraid. I am almost finished.
Last Word
- I strongly advice you to look over my code if you're interested in using it. Mathematics imply. Computers reply.
History
- 2015.07.18 This version fixes division error.
- 2013.04.15 This version fixes not starting error. And adds start over button.
- 2011.06.11: This version supports radix conversion using the
DecimalToRadix
and ToDecimal
methods.
- 2011.06.10: This version does not provide support for floating number implementation. m1 / m2 simply returns m1 // m2 (true div: remainder trimmed).