Introduction
A majority of web applications need a navigation menu and there are a lot of plug-ins out there to create a great menu. In my case, I had to create a vertical navigation menu with some expanding and collapsing behavior.
There are a lot of solutions for creating such a menu. I was already using jQueryUI and decided to use the Accordion for this purpose. This is what happened… Let's say that we have a simple site where we have home, projects, and About me pages and we need to create a vertical navigation menu for it using jQueryUI.
Using the Code
The first step was to create an Accordion with the navigation links:
<div id="navigationMenu">
<h3> <a href="#"> Home </a> </h3>
<div> </div>
<h3><a href="#"> Projects</a></h3>
<div>
<ul>
<li> <a href="#1"> Project 1</a> </li>
<li> <a href="#2"> Project 2 </a> </li>
<li> <a href="#3"> Project 3 </a> </li>
</ul>
</div>
<h3> <a href="#"> About me</a></h3>
<div></div>
</div>
We have to initialize the navigationMenu
as an Accordion
:
$(function () {
$('#navigationMenu').accordion({
collapsible: true,
navigation: true,
clearStyle: true
});
});
OK, now we can add some code for customizing the navigation accordion. Let's decorate the menu headers and div
s that don’t need to be collapsible and single with a class="single-menu-item"
for headers and class="single-menu-container"
for the div
s:
<div id="navigationMenu">
<h3 class="single-menu-item"> <a href="#"> Home </a> </h3>
<div class="single-menu-container"> </div>
<h3><a href="#"> Projects</a></h3>
<div>
<ul>
<li> <a href="#1"> Project 1</a> </li>
<li> <a href="#2"> Project 2 </a> </li>
<li> <a href="#3"> Project 3 </a> </li>
</ul>
</div>
<h3 class="single-menu-item"> <a href="#"> About me</a></h3>
<div class="single-menu-container"></div>
</div>
We unbind the click event from the single-menu
element:
$(function () {
...
$('.single-menu-item').unbind('click');
});
And as a last step, we have to create some CSS rules for single-menu-item
and single-menu-container
that will rewrite the standard behavior of the jQuery accordion:
#navigationMenu{ width: 270px; }
.single-menu-item .ui-icon {display: none !important;}
.single-menu-container {display: none !important;}
This was the last step.
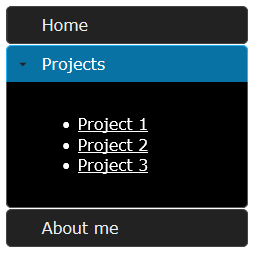
Now we have a vertical navigation menu! You can find the code of this example here.
If you have a great example of implementing a vertical navigation menu with jQuery, please provide the links in the comments section.