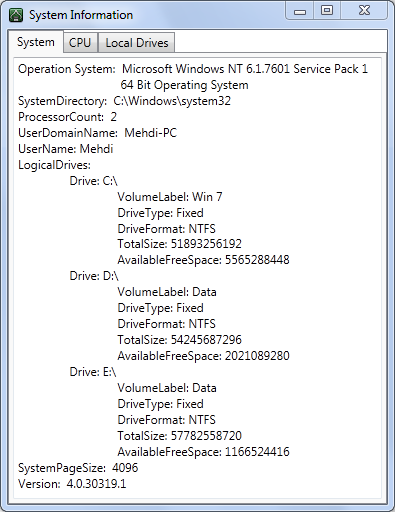
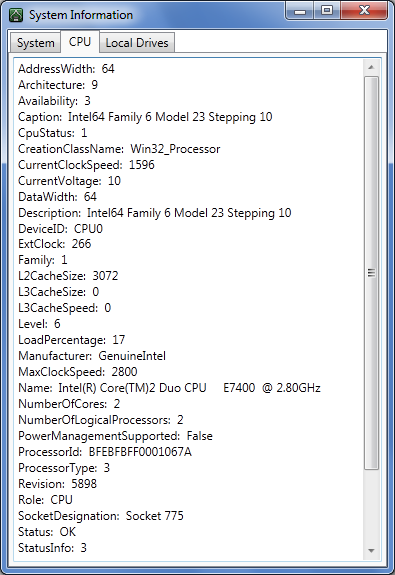
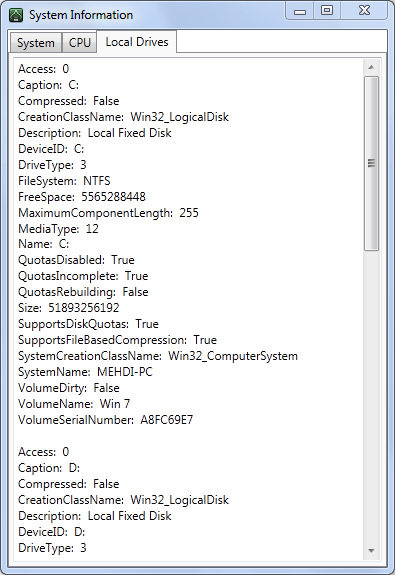
Introduction
In this application, user system information has been collected by using two classes:
System.Environment
System.Managememnt
Using the Code
The main tab in this application has three items:
- System
- CPU
- Local drives
In the System tab, the System.Environment
class has been used to gather information:
private string SystemInformation()
{
StringBuilder StringBuilder1 = new StringBuilder(string.Empty);
try
{
StringBuilder1.AppendFormat("Operation System: {0}\n", Environment.OSVersion);
if (Environment.Is64BitOperatingSystem)
StringBuilder1.AppendFormat("\t\t 64 Bit Operating System\n");
else
StringBuilder1.AppendFormat("\t\t 32 Bit Operating System\n");
StringBuilder1.AppendFormat("SystemDirectory: {0}\n", Environment.SystemDirectory);
StringBuilder1.AppendFormat("ProcessorCount: {0}\n", Environment.ProcessorCount);
StringBuilder1.AppendFormat("UserDomainName: {0}\n", Environment.UserDomainName);
StringBuilder1.AppendFormat("UserName: {0}\n", Environment.UserName);
StringBuilder1.AppendFormat("LogicalDrives:\n");
foreach (System.IO.DriveInfo DriveInfo1 in System.IO.DriveInfo.GetDrives())
{
try
{
StringBuilder1.AppendFormat("\t Drive: {0}\n\t\t VolumeLabel: " +
"{1}\n\t\t DriveType: {2}\n\t\t DriveFormat: {3}\n\t\t " +
"TotalSize: {4}\n\t\t AvailableFreeSpace: {5}\n",
DriveInfo1.Name, DriveInfo1.VolumeLabel, DriveInfo1.DriveType,
DriveInfo1.DriveFormat, DriveInfo1.TotalSize, DriveInfo1.AvailableFreeSpace);
}
catch
{
}
}
StringBuilder1.AppendFormat("SystemPageSize: {0}\n", Environment.SystemPageSize);
StringBuilder1.AppendFormat("Version: {0}", Environment.Version);
}
catch
{
}
return StringBuilder1.ToString();
}
In the CPU and Local drive tabs, the System.Management
class has been used to gather information but before using this class, it has to be added to references.
Add System.Management
to references:
- Go to Solution Explorer.
- Right click on References and select Add Reference.
- Click on .NET tab.
- Select
System.Management
. - Select the OK button.
Now we can use this class in the following function:
private string DeviceInformation(string stringIn)
{
StringBuilder StringBuilder1 = new StringBuilder(string.Empty);
ManagementClass ManagementClass1 = new ManagementClass(stringIn);
ManagementObjectCollection ManagemenobjCol = ManagementClass1.GetInstances();
PropertyDataCollection properties = ManagementClass1.Properties;
foreach (ManagementObject obj in ManagemenobjCol)
{
foreach (PropertyData property in properties)
{
try
{
StringBuilder1.AppendLine(property.Name + ": " +
obj.Properties[property.Name].Value.ToString());
}
catch
{
}
}
StringBuilder1.AppendLine();
}
return StringBuilder1.ToString();
}
Notice that this function has an input string which can be Win32_Processor
or Win32_LogicalDisk
. We use the first one for collecting
information about CPU and the second one for local drives. Now in the Window_Loaded
event, we call these functions:
textBox1.Text = SystemInformation();
textBox2.Text = DeviceInformation("Win32_Processor");
textBox3.Text = DeviceInformation("Win32_LogicalDisk");
Points of Interest
In this tip, you learned how to collect system information by using System.environment
and System.managememnt
.