Introduction
Be careful when you are using PLINQ. What I found is if the computation
is very simple and non-expensive, it is better to use LINQ than PLINQ.
Using the code
Following code is tested in multicore machine (8 CPU). First
I timed a CPU intensive code with LINQ and then with PLINQ. Then I timed NON
CPU intensive code with LINQ and PLINQ.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Diagnostics;
using System.Threading;
namespace PLinqSample
{
public class Employee
{
public string Name { get; set; }
public double Salary { get; set; }
public bool ExpensiveComputation()
{
Thread.Sleep(10);
return (Salary > 2000 && Salary < 3000);
}
public bool NonExpensiveComputation()
{
return (Salary > 2000 && Salary < 3000);
}
}
class Program
{
static void Main(string[] args)
{
List<Employee> employeeList = GetData();
Stopwatch stopWatch = new Stopwatch();
stopWatch.Start();
var linqResult = employeeList.Where<Employee>(e=> e.ExpensiveComputation());
int empCount = linqResult.Count();
stopWatch.Stop();
Console.WriteLine(string.Format("Time taken by old LINQ in Expensive Computation is {0} to get {1} Employees", stopWatch.Elapsed.TotalMilliseconds, empCount));
stopWatch.Reset();
stopWatch.Start();
linqResult = employeeList.AsParallel<Employee>().Where<Employee>(e=> e.ExpensiveComputation());
empCount = linqResult.Count();
stopWatch.Stop();
Console.WriteLine(string.Format("Time taken by new PLINQ in Expensive Computation is {0} to get {1} Employees", stopWatch.Elapsed.TotalMilliseconds, empCount));
stopWatch.Reset();
Console.WriteLine();
stopWatch.Start();
linqResult = employeeList.Where<Employee>(e => e.NonExpensiveComputation());
empCount = linqResult.Count();
stopWatch.Stop();
Console.WriteLine(string.Format("Time taken by old LINQ in Non Expensive Computation is {0} to get {1} Employees", stopWatch.Elapsed.TotalMilliseconds, empCount));
stopWatch.Reset();
stopWatch.Start();
linqResult = employeeList.AsParallel<Employee>().Where<Employee>(e => e.NonExpensiveComputation());
empCount = linqResult.Count();
stopWatch.Stop();
Console.WriteLine(string.Format("Time taken by new PLINQ in Non Expensive Computation is {0} to get {1} Employees", stopWatch.Elapsed.TotalMilliseconds, empCount));
stopWatch.Reset();
Console.ReadKey();
}
static List<Employee> GetData()
{
List<Employee> employeeList = new List<Employee>();
Random random = new Random(1000);
for (int i = 0; i < 1000; i++)
{
employeeList.Add(new Employee() { Name = "Employee" + i, Salary = GetRandomNumber(random, 1000, 5000)});
}
return employeeList;
}
static double GetRandomNumber(Random random, double minimum, double maximum)
{
return random.NextDouble() * (maximum - minimum) + minimum;
}
}
}
Here is the result of above code
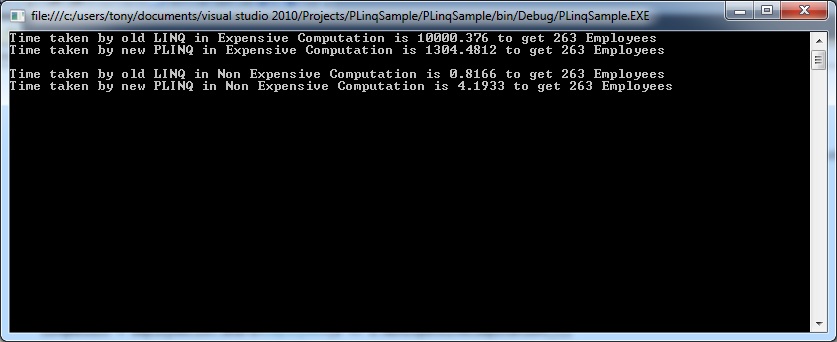