Introduction
I will introduce how to use Oracle with Entity Framework.
Background
You should have VS2010, .NET Framework 4 or higher, Oracle 10g or higher version, ODAC 11.2 Release 4 (11.2.0.3.0) with Oracle Developer Tools for Visual Studio (download from Oracle.org).
Using the Code
First, create a table in your Oracle database:
CREATE TABLE test_tbl (
id INT,
val VARCHAR(10),
PRIMARY KEY (id)
);
Now try to connect to Oracle with EF:
- Create project Open VS2010, create a console applicationNew | Project | | Console application
- Add ADO.NET Entity Data Model Right click the project | Add | New Item , Click "ADO.NET Entity Data Model ", and the default name is "Model1.edmx" , click "Add"
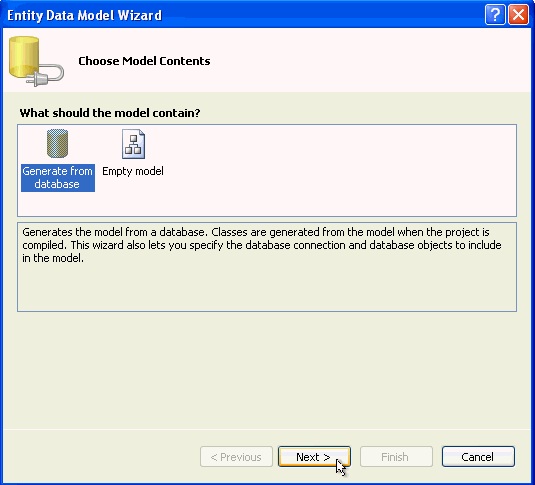
Choose "Generate from database", click "Next".

Click "New Connection... " setup the connection to the Oracle.

Choose the table and click "Finish".
VS2O1O will generate the entities for you.
Now , write code like the following:
static void Main(string[] args)
{
using (Entities ctx = new Entities())
{
var rst = from tbl in ctx.TEST_TBL
select tbl;
foreach (var tbl in rst)
{
Console.WriteLine(tbl.ID);
}
}
}
You can get values from Oracle.
Points of Interest
By now, we know how to deal with single table, further, we would research more table and the relationship between tables (one-to-one, one-to-many, etc.).
History