Introduction
This article tells how to merge multiple presentation files to one file at a specified position using Office OpenXML SDK.
Overview
Let's copy one presentation to other manually. I have two presentation files: ppt1.pptx and ppt2.pptx. I would copy all slides
from ppt2.pptx into ppt1.pptx.
ppt1.pptx
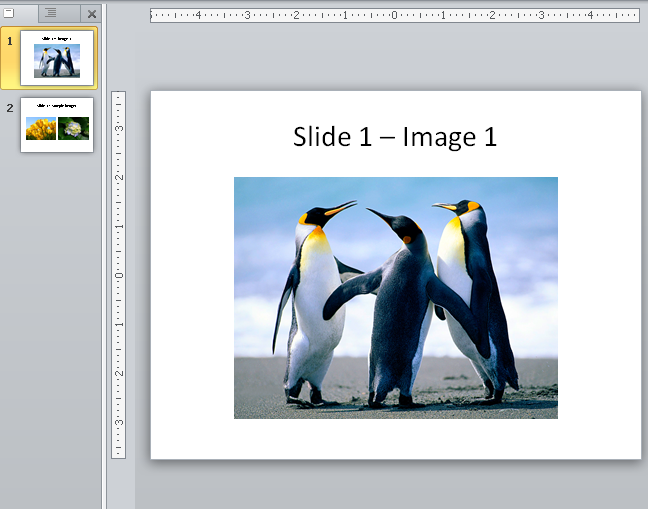
ppt2.pptx

Resultant presentation

This looks pretty easy if there are only couple of presentations to be copied. Think about the case where you need to copy/merge many presentations.
So, I thought of copying these programmatically using Office OpenXML SDK 2.0.
Using the code
The PresentationInfo
class contains information of file and its insert position in the destination file.
public class PresentationInfo
{
public string File { get; set; }
public short InsertPosition { get; set; }
}
The MergeHandler
class contains a method MergeAllSlides
which takes parameters source presentation, destination presentation and a list
of presentations to be merged, and copies all the slides in these presentations into the destination presentation.
void MergeAllSlides(string parentPresentation, string destinationFileName, List<PresentationInfo> mergingFileList);
MergeAllSlides
does two things. First, it merges all the slides keeping count of source and destination
locations of each slide. Later, it re-orders the slides.
Dictionary<int, int> reOrderPair = new Dictionary<int, int>();
foreach (PresentationInfo importedFile in mergingFileList)
{
try
{
int val = (importedFile.InsertPosition - 1) + reOrderPair.Count;
this.MergeSlides(importedFile, destinationFileName,
ref reOrderPair, ref val, out reOrderConstant);
}
catch
{
continue;
}
}
foreach (KeyValuePair<int, int> reOrderValue in reOrderPair)
{
try
{
this.ReorderSlides(destinationFileName, reOrderValue.Key, reOrderValue.Value);
}
catch
{
continue;
}
}
The caller would need to create the list of PresentationInfo
and call
MergeAllSlides
.
string ppt1 = "ppt1.pptx";
string ppt2 = "ppt2.pptx";
new MergeHandler().MergeAllSlides(ppt1, ppt1, new List<PresentationInfo>()
{
new PresentationInfo()
{
File = ppt2,
InsertPosition = 2
}
});
Using the above code, the slides would be inserted in second position.

Point Of Interest
- As you have observed, during the copy of a slide, the referred master and layout slide is also copied each time. This results in increased
file size and additional time overhead. So, the code can further be re-factored to copy master
and layout slides only once.
- I have taken an approach where all slides from ppt2.pptx are copied into ppt1.pptx.
This can further be refined to copy only the selected files from ppt2.pptx.
- To copy multiple presentation files into one file, all we need to do is create multiple
PresentationInfo
in the list.
new MergeHandler().MergeAllSlides(ppt1, ppt1, new List<PresentationInfo>()
{
new PresentationInfo()
{
File = ppt2,
InsertPosition = 2
},
new PresentationInfo()
{
File = @"c:\wip\ppt3",
InsertPosition = 3
},
new PresentationInfo()
{
File = @"c:\wip\ppt4",
InsertPosition = 6
},
new PresentationInfo()
{
File = @"c:\wip\ppt5",
InsertPosition = 1
}
});