Introduction
The LapTimer
class discussed in this tip is a simple but object oriented implementation of a Timer
functionality, which facilitates adding intermediate time-stamp values (typically knows as laps) along with a custom comment for each such lap. Imagine an Athlete running in Olympic Games (thought of it just like that) and we need to track the timing of each of his laps. This LapTimer
class does precisely the same.
Background
To be very frank, this tip was not planned at all. Neither was this code. It has been years since I have written any code. C# a little but JavaScript? Not for many many years. So bear with me if you find any obvious mistakes.
When helping out some developers on my team, I came across a requirement to use a Lap Timer like functionality to record time after specific steps. This was mainly for debugging performance issues on the client side scripts.
Since the team was busy in some other burning issues as well, I thought it might just help them (and ourselves) out. I tried searching for a ready-made code for this but I could not find anything like that (at least not in the form I have published here.) If you do come across another solution for the same problem, please point it out here.
The LapTimer Class
If you have already guessed the methods of this class, I would say I have succeeded in keeping it simple. The class has straightforward methods like start()
, stop()
, reset()
as you would imagine with any timer type of implementation.
Again for simplicity (and for some tweaks, which I'll explain later), I have provided a default global instance of the
LapTimer
class named "defaultLapTimer
". This instance is expected to be used directly for all simple and typical lap timer needs.
Usage
Sample usage is provided in the code snippet below:
defaultLapTimer.start();
defaultLapTimer.addLap("Pass 1");
defaultLapTimer.addLap("Pass 2");
defaultLapTimer.addLap("Pass 3");
defaultLapTimer.addLap("Pass 4");
defaultLapTimer.stop();
alert(defaultLapTimer.getAllLaps());
alert(defaultLapTimer.TotalDuration);
In addition, if really needed, multiple additional instances of the LapTimer
class can be created and used across pages.
var lapTimer2 = new LapTimer();
lapTimer2.addLap("Comment 1");
lapTimer2.addLap("Comment 2");
lapTimer2.addLap("Comment 3");
alert(lapTimer2.getAllLaps());
Use the reset()
method to clear all these counters and start using the same lapTimer
instance again.
Outputs
For simplicity and as per our current needs, the string
equivalent of each lap's information is captured and stored in the LapTimer
class instances. This information is returned by the getAllLaps()
method call. The method gets this information from the member named "LapsInfoStrings
" which happens to be an array. One can easily use it as any other class member, i.e. defaultTimer.LapsInfoStrings.
If this string
representation is not useful, and there is more control needed using the direct time intervals of the lap times, then another property named "LapTimes
" can be used. This property is an array of Date()
instances, each representing the lap recorded by the method call addLap()
.
Here's what you can expect in the outputs.
alert(defaultLapTimer.getAllLaps());
This produces the following output:
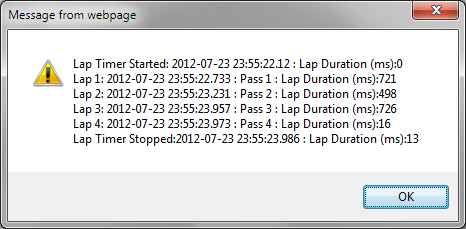
alert(defaultLapTimer.TotalDuration);
This produces the following output:

The Code
The code provided with this tip contains just two files.
- LapTimerClass.js - The file contains the source of code class
LapTimer
and some other related utility JavaScript methods used by the class. - TimerTestPage.html - This is just a plain HTML page showing the usage and working of the
LapTimer
class.
History
I'll keep you guys updated if I make any changes to this over time. Please do point out any simpler alternative or interesting solution approaches.