Introduction
Just a really simple window that allows you to change the look and feel to any of the installed ones.
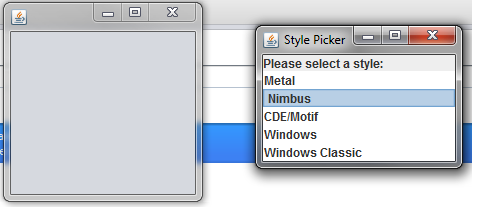
Using the code
Use the class below like this, where 'frame' is the JFrame you wish to modify the style of:
public class Entry {
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setSize(new Dimension(200,200));
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
new LookAndFeelPicker(frame);
}
}
Copy the following class into your project and edit it as you wish:
import java.awt.BorderLayout;
import javax.swing.DefaultListModel;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JList;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
import javax.swing.UIManager;
import javax.swing.UIManager.LookAndFeelInfo;
import javax.swing.UnsupportedLookAndFeelException;
import javax.swing.event.ListSelectionEvent;
import javax.swing.event.ListSelectionListener;
public class LookAndFeelPicker extends JFrame
{
public LookAndFeelPicker(JFrame target)
{
setTitle("Style Picker");
InitControls(target);
pack();
setVisible(true);
}
private void InitControls(JFrame target)
{
JPanel pnlContent = new JPanel();
pnlContent.setLayout(new BorderLayout());
getContentPane().add(pnlContent);
JLabel lblInstructions = new JLabel("Please select a style:");
pnlContent.add(lblInstructions, BorderLayout.PAGE_START);
DefaultListModel dlmListData = new DefaultListModel();
JList lstStyles = new JList(dlmListData);
LookAndFeelInfo[] lafInfo = UIManager.getInstalledLookAndFeels();
for(LookAndFeelInfo laf : lafInfo)
{
dlmListData.addElement(laf.getName());
}
lstStyles.addListSelectionListener(new StyleSelectionHandler(target));
pnlContent.add(lstStyles, BorderLayout.CENTER);
}
}
class StyleSelectionHandler implements ListSelectionListener
{
private JFrame jfrFrameToUpdate;
public StyleSelectionHandler(JFrame mainFrame)
{
jfrFrameToUpdate = mainFrame;
}
@Override
public void valueChanged(ListSelectionEvent e)
{
JList lstStyles = (JList)e.getSource();
if(!lstStyles.isSelectionEmpty())
{
try {
UIManager.setLookAndFeel(
UIManager.getInstalledLookAndFeels()[lstStyles.getSelectedIndex()].getClassName());
SwingUtilities.updateComponentTreeUI(jfrFrameToUpdate);
} catch (ClassNotFoundException | InstantiationException
| IllegalAccessException | UnsupportedLookAndFeelException e1) {
System.out.println(e1.getMessage());
}
}
}
}
History
Version 1 - Uploaded.