Introduction
This article describes bit array classes using bit field and union. This implementation is fast and optimized when compared with the bitset
class and is very simple to make complex operations when compared with normal operations.
The total memory size of CByte
is only one byte, of CShort
is 2 bytes, and CInt
is 4 bytes (no extra size).
It's fast and stable in large calculations.
The usage is simple like bitset:
CByte byte1=1;
CByte byte2=9;
byte1.b4=byte2[4];
Background
Bit Fields: MASN
In addition to declarators for members of a structure or union, a structure declarator can also be a specified number of bits, called a "bit field." Its length is set off from the declarator for the field name by a colon. A bit field is interpreted as an integral type.
The following example declares a structure that contains bit fields:
struct Date
{
unsigned nWeekDay : 3; unsigned nMonthDay : 6; unsigned nMonth : 5; unsigned nYear : 8; };
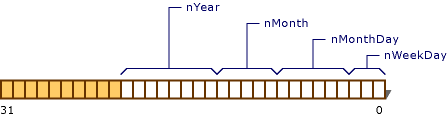
Note that nYear
is 8 bits long and would overflow the word boundary of the declared type, unsigned int. Therefore, it is begun at the beginning of a new unsigned int. It is not necessary that all bit fields fit in one object of the underlying type; new units of storage are allocated according to the number of bits requested in the declaration.
Union: MSDN
A union is a user-defined data or class type that, at any given time, contains only one object from its list of members (although that object can be an array or a class type).
union DATATYPE { char ch;
int i;
long l;
float f;
double d;
} var1;
The member-list of a union represents the kinds of data the union can contain. A union requires enough storage to hold the largest member in its member-list.
A C++ union is a limited form of the class type. It can contain access specifiers (public
, protected
, private
), member data, and member functions, including constructors and destructors. It cannot contain virtual functions or static
data members. It cannot be used as a base class, nor can it have base classes. The default access of members in a union is public
.
Part of CByte Implementation
union CByte
{
unsigned char Value;
struct {
unsigned char b0:1;
unsigned char b1:1;
unsigned char b2:1;
unsigned char b3:1;
unsigned char b4:1;
unsigned char b5:1;
unsigned char b6:1;
unsigned char b7:1;
};
.........
Value and the bits struct
refer to the same memory. When any change is made in the value, it will affect the bits and vice versa.
Using the Code
The code has the implementation for most operator overloadings like +,-,++,--,[ ],....
You can get or set any bit in one step very fast and simply.
byte1.b4=byte2[4];
Code example:
CByte byte1=1;
CByte byte2=9;
CByte byte3=5;
byte1=5;
byte2=255;
byte1.b4=1;
byte2.b7=0;
byte1.b4=byte2[4];
byte2.b7=byte1[7];
byte2.b0=byte1[7];
byte2.b0=byte1[7];
byte1.b4=byte2[4];
byte2.b7=byte1[7];
byte2.b0=byte1[7];
byte2.b0=byte1[7];
byte3=byte1+byte2;