Introduction
I was looking for a simple solution of a common problem: you have created an update of your application.
After you uploaded it to the server, you want that all the "old" applications (clients) get a message about this new version by checking for this automatically.
Background
My solution is very simple, the .NET DLL is small.
This XML file manages the updates (new Version 9.1.5 at https://leochapiro.de/data/TestApp.exe):
="1.0"="UTF-8"="yes"
<myCoolApp>
<currentVersion>
<major>9</major>
<minor>1</minor>
<build>5</build>
</currentVersion>
<path>https://myCoolApp.zip</path>
</myCoolApp>
The main function Check4Update()
reads the XML file and parses it:
XmlDocument oDom = new XmlDocument();
oDom.Load(_sXmlConfig);
string str = oDom.SelectSingleNode("//currentVersion/major").InnerText;
Int32.TryParse(str, out _nMajor);
str = oDom.SelectSingleNode("//currentVersion/minor").InnerText;
Int32.TryParse(str, out _nMinor);
str = oDom.SelectSingleNode("//currentVersion/build").InnerText;
Int32.TryParse(str, out _nBuild);
_sNewVersionPath = oDom.SelectSingleNode("//path").InnerText;
Using the Code
This solution is a .NET library (DLL) and can be used in every C# project by adding it as reference:
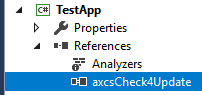
Then you only need to create an instance of it:
axcsCheck4Update.axMain oCheckClient = new axcsCheck4Update.axMain(txtXml.Text);
int nMajor = oCheckClient.GetVersion(axcsCheck4Update.enVerion.EMajor);
int nMinor = oCheckClient.GetVersion(axcsCheck4Update.enVerion.EMinor);
int nBuild = oCheckClient.GetVersion(axcsCheck4Update.enVerion.EBuild);
string strPath = oCheckClient.GetNewVersionPath();
After getting the current version's number, you can compare it to your version's number:
Assembly assembly = Assembly.GetExecutingAssembly();
FileVersionInfo fileVersionInfo = FileVersionInfo.GetVersionInfo(assembly.Location);
int nAppMajor = fileVersionInfo.FileMajorPart;
int nAppMinor = fileVersionInfo.FileMinorPart;
int nAppBuild = fileVersionInfo.FileBuildPart;
And, if they are different, you can point users to this new version like this:
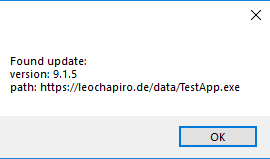
History