In most applications, there is a field while registering to enter a valid password that should contain at least one digit, one number and one special symbol. In this tip, we are going to learn how to create a password strength bar that will show whether the entered password is weak, good, or strong.
Prerequisites
- Basic knowledge of Angular
- Visual Studio Code must be installed
- Angular CLI must be installed
- Node JS must be installed
Step 1
Let's create a new Angular project using the following NPM command:
ng new passwordStrengthBar
Step 2
Now, let's create a new component by using the following command:
ng g c password-strength-bar
Step 3
Now, open the password-strength-bar.component.html file and add the following code in the file:
<div id="strength" style="margin: 11px"> <small>{{barLabel}}</small>
<ul id="strengthbar">
<li> </li>
<li class="point"> </li>
<li class="point"> </li>
<li class="point"> </li>
<li class="point"> </li>
<li class="point">
Step 4
Now, open the password-strength-bar.component.ts file and add the following code in this file:
import {Component, OnChanges, Input, SimpleChange} from '@angular/core';
@Component({
selector: 'app-passoword-strength-bar',
templateUrl: './passoword-strength-bar.component.html',
styleUrls: ['./passoword-strength-bar.component.css']
})
export class PassowordStrengthBarComponent implements OnChanges {
@Input() passwordToCheck: string;
@Input() barLabel: string;
bar0: string;
bar1: string;
bar2: string;
bar3: string;
bar4: string;
private colors = ['#F00', '#F90', '#FF0', '#9F0', '#0F0'];
private static measureStrength(pass: string) {
let score = 0;
let letters = {};
for (let i = 0; i< pass.length; i++) {
letters[pass[i]] = (letters[pass[i]] || 0) + 1;
score += 5.0 / letters[pass[i]];
}
let variations = {
digits: /\d/.test(pass),
lower: /[a-z]/.test(pass),
upper: /[A-Z]/.test(pass),
nonWords: /\W/.test(pass),
};
let variationCount = 0;
for (let check in variations) {
variationCount += (variations[check]) ? 1 : 0;
}
score += (variationCount - 1) * 10;
return Math.trunc(score);
}
private getColor(score: number) {
let idx = 0;
if (score > 90) {
idx = 4;
} else if (score > 70) {
idx = 3;
} else if (score >= 40) {
idx = 2;
} else if (score >= 20) {
idx = 1;
}
return {
idx: idx + 1,
col: this.colors[idx]
};
}
ngOnChanges(changes: {[propName: string]: SimpleChange}): void {
var password = changes['passwordToCheck'].currentValue;
this.setBarColors(5, '#DDD');
if (password) {
let c = this.getColor(PassowordStrengthBarComponent.measureStrength(password));
this.setBarColors(c.idx, c.col);
}
}
private setBarColors(count, col) {
for (let _n = 0; _n < count; _n++) {
this['bar' + _n] = col;
}
}
}
Step 5
Now, open the password-strength-bar.component.css file and add the following code:
ul#strengthBar {
display:inline;
list-style:none;
margin:0;
margin-left:15px;
padding:0;
vertical-align:2px;
}
.point:last {
margin:0 !important;
}
.point {
background:#DDD;
border-radius:2px;
display:inline-block;
height:5px;
margin-right:1px;
width:20px;
}
Step 6
Now, open the app.component.html file and add the following code in this file:
Password Strength Bar
<div class="container">
<div class="row">
<div class="col-md-8 col-md-offset-2">
<div class="panel panel-default">
<div class="panel-body">
<div class="form-group"> Email
<div class="col-md-6">
<div class="form-group"> Password
<div class="col-md-6">
<app-passoword-strength-bar>
Step 7
Now, open the app.component.ts file and add the following code:
import { Component, OnInit } from '@angular/core';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
public account = {
password: null
};
public barLabel: string = "Password strength:";
constructor() { }
ngOnInit() {
}
}
Step 8
Now, open the app.module.ts file and add the following code:
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { AppComponent } from './app.component';
import { PassowordStrengthBarComponent }
from './passoword-strength-bar/passoword-strength-bar.component';
@NgModule({
declarations: [
AppComponent,
PassowordStrengthBarComponent
],
imports: [
BrowserModule,
FormsModule,
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Step 9
Now let's run the project by using 'npm start
' or 'ng serve
' command and check the output.
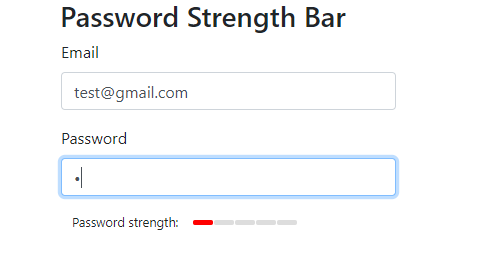




Summary
In this tip, we learned how we can create a password strength bar in Angular 8 applications.
Please give your valuable feedback/comments/questions about this post. Please let me know if you liked and understood it and how I can improve upon it.
History
- 23rd March, 2020: Initial version
This member has not yet provided a Biography. Assume it's interesting and varied, and probably something to do with programming.