In this tip, you will find an implementation of control CComboBox with checkbox list.
Introduction
This example shows how to implement your own combobox
control with check boxes like a list item.
Using the Code
There was a need to create a combobox
with the ability to select several elements.
Since the MFC does not have such a control, I had to implement my control on the basis of a combobox
.
This control is based on standard CComboBox
.
For using it, just create a combobox
in your form with special attributes:
Owner Draw
- Variable
Has Strings
- True
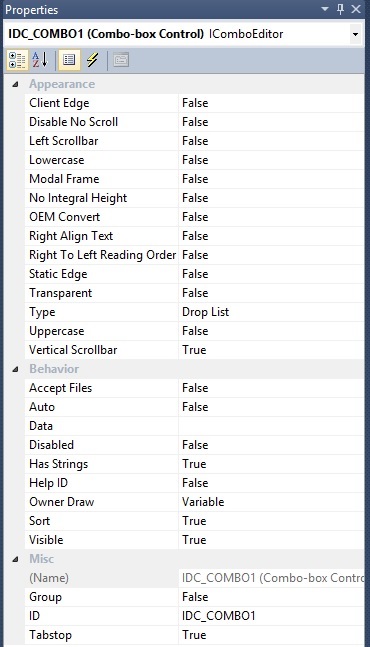
Include Combo.h and Combo.cpp to your project.
In these files, ChkCombo
class is described.
Create the example of this class in your application.
ChkCombo m_Combo;
The next step for using it is that you have to associate the standard ComboBox
with this class.
void CCCOMBODlg::DoDataExchange(CDataExchange* pDX)
{
DDX_Control(pDX, IDC_COMBO1, m_Combo);
......
CDialogEx::DoDataExchange(pDX);
}
So, now all ready for using this control.
At first, you need to add some string
s in ChkCombo
and for example, check some items.
m_Combo.AddString(L"Red");
m_Combo.AddString(L"Green");
m_Combo.AddString(L"Blue");
m_Combo.AddString(L"Black");
m_Combo.AddString(L"White");
m_Combo.AddString(L"Yellow");
m_Combo.AddString(L"Brown");
m_Combo.SetCheck(0, TRUE);
m_Combo.SetCheck(1, TRUE);
m_Combo.SetCheck(2, TRUE);
For send events, this control has a special message with code THIS_CHECKED
.
This message will be sent every time you check/uncheck some item.
Example of how to use it:
BEGIN_MESSAGE_MAP(CCCOMBODlg, CDialogEx)
...........................................................
ON_MESSAGE(THIS_CHECKED, &CCCOMBODlg::OnChecked)
...........................................................
END_MESSAGE_MAP()
LRESULT CCCOMBODlg::OnChecked(WPARAM wp, LPARAM lp)
{
int ID = (DWORD)lp;
int index = LOWORD(wp);
bool flag = HIWORD(wp);
wchar_t text_s[32];
memset(text_s, 0, 32 * sizeof(wchar_t));
wsprintf(text_s, L"%d __ %d", index, flag);
if(ID == IDC_COMBO1)
GetDlgItem(IDC_STATIC_CHK)->SetWindowText(text_s);
return 0;
}
This example shows how to get control ID and get information about what item changed and the current state.

I hope this class can help you in your work. :)
History
- 4th December, 2020: Initial version