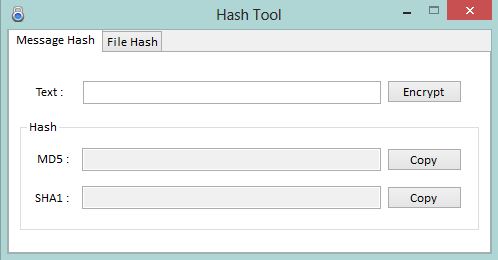
Introduction
This is my second article on CodeProject. Here I am demonstrating a tool to generate MD5 and SHA-1 checksums.
Using the code
You can use an executable directly. To edit code, you can import it in Visual Studio.
Points of Interest
To compute the hash of large files, the ComputeHash
function cannot be directly used since
the application will go unresponsive for large files.
To prevent this from happening, a hash is generated in another thread using a BackgroundWorker
.
private void button5_Click(object sender, EventArgs e) {
...
getFileMD5.DoWork += new DoWorkEventHandler(MD5_DoWork);
getFileMD5.RunWorkerCompleted += new RunWorkerCompletedEventHandler(MD5_Completed);
getFileMD5.RunWorkerAsync();
...
}
public void MD5_DoWork(object sender, DoWorkEventArgs e) {
...
while((readCount = stream.Read(buffer, 0, bufferSize)) > 0) {
algorithm.TransformBlock(buffer, 0, readCount, buffer, 0);
progressBar1.Invoke((MethodInvoker)delegate() {
if(progressBar1.Value < progressBar1.Maximum)
progressBar1.Value += 1;
else
progressBar1.Value = 0;
});
}
...
}
public void MD5_Completed(object sender, RunWorkerCompletedEventArgs e){
...
stream.Close();
textBox5.Text = ((string)e.Result).ToLowerInvariant();
System.Windows.Forms.Clipboard.SetText(textBox5.Text);
...
}
About this project
This tool I have written for my personal use and wanted to share with others. If it proves to be
of any help, please let me know.