A TIFF image can contain one or more images. In this tip, you will see how to merge multiple images using VB.NET.
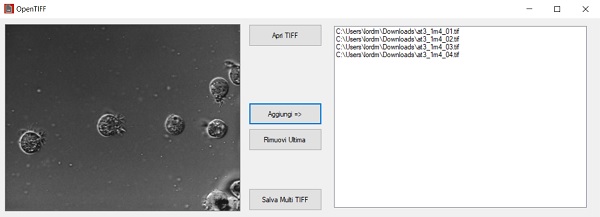
Introduction
In this period, PDF is used. There was a time when TIFF was also used. This Visual Basic application solves the problem of creating a multipage TIFF image from an ordered series of single TIFF images.
It should be remembered that TIFFs can be compressed, this article does not consider this aspect. The reference framework for this project code is .NET 4.8.
Using the Code
Two main functions are used in the project code: saveImageTIFF()
and GetEncoderInfo()
. The code is relatively simple. A Button1
button is used to choose the TIFFs to be used, selecting the first one and then the last one. There is no multiselect by choice as, by mistake, we may select the images not in the desired order. Button2
and Button3
respectively add and remove the full path of the image to a Listbox
always in order. Button4
calls the saveImageTIFF()
function. The TIFF image information is retrieved by the GetEncoderInfo ("image / tiff")
function which basically retrieves the correct TIFF codec from a set of codecs. The last sub shows the images selected in the Listbox
inside the PictureBox
.
The Full Code!
//
Imports System.Drawing.Imaging
Imports System.IO.File
Public Class Form1
Dim ThisTIFF As String = ""
Dim Counter As Integer = 0
Dim objfile As System.IO.File
Private Sub Button1_Click(sender As Object, e As EventArgs) Handles Button1.Click
OpenFileDialog1.Filter = "TIF Files|*.tif|TIFF Files|*.tiff"
OpenFileDialog1.FileName = ""
If OpenFileDialog1.ShowDialog() = DialogResult.OK Then
PictureBox1.Image = Image.FromFile(OpenFileDialog1.FileName.ToString)
PictureBox1.SizeMode = PictureBoxSizeMode.StretchImage
ThisTIFF = OpenFileDialog1.FileName.ToString
End If
End Sub
Private Sub Button2_Click(sender As Object, e As EventArgs) Handles Button2.Click
ListBox1.Items.Add(ThisTIFF)
Counter += 1
End Sub
Private Sub Button3_Click(sender As Object, e As EventArgs) Handles Button3.Click
If Counter <> 0 Then
ListBox1.Items.RemoveAt(Counter - 1)
Counter -= 1
End If
End Sub
Private Sub Button4_Click(sender As Object, e As EventArgs) Handles Button4.Click
saveImageTIFF()
End Sub
Private Sub saveImageTIFF()
Dim strFileName As String = ""
SaveFileDialog1.AddExtension = True
SaveFileDialog1.ShowDialog()
strFileName = SaveFileDialog1.FileName().ToString
Me.Cursor = System.Windows.Forms.Cursors.WaitCursor
PictureBox1.Image = Image.FromFile(ListBox1.Items.Item(0).ToString)
Dim saveTif As Bitmap = New Bitmap(PictureBox1.Image)
Dim myImageCodecInfo As ImageCodecInfo
Dim myEncoder As Encoder
Dim myEncoderParameter As EncoderParameter
Dim myEncoderParameters As EncoderParameters
myImageCodecInfo = GetEncoderInfo("image/tiff")
myEncoder = Encoder.SaveFlag
myEncoderParameters = New EncoderParameters(1)
myEncoderParameter = New EncoderParameter(myEncoder, CLng(EncoderValue.MultiFrame))
myEncoderParameters.Param(0) = myEncoderParameter
saveTif.Save(strFileName, myImageCodecInfo, myEncoderParameters)
Dim i As Integer
ListBox1.Items.RemoveAt(0)
Dim frameCount = ListBox1.Items.Count
Dim pageNO
Try
For i = 0 To frameCount - 1
pageNO = ListBox1.Items.Item(i)
PictureBox1.Image = Image.FromFile(ListBox1.Items.Item(i))
If objfile.Exists(strFileName) Then
Dim saveFrame As Bitmap = New Bitmap(PictureBox1.Image)
myEncoderParameter = New EncoderParameter_
(myEncoder, CLng(EncoderValue.FrameDimensionPage))
myEncoderParameters.Param(0) = myEncoderParameter
saveTif.SaveAdd(saveFrame, myEncoderParameters)
saveFrame.Dispose()
End If
Next
Catch ex As Exception
MsgBox(ex.Message)
End Try
saveTif.Dispose()
Me.Cursor = System.Windows.Forms.Cursors.Default
MsgBox("File salvato come " & strFileName)
End Sub
Private Shared Function GetEncoderInfo(ByVal mimeType As [String]) As ImageCodecInfo
Dim i As Integer
Dim encoders() As ImageCodecInfo
encoders = ImageCodecInfo.GetImageEncoders()
For i = 0 To (encoders.Length - 1)
If (encoders(i).MimeType = mimeType) Then
Return encoders(i)
End If
Next i
End Function
Private Sub ListBox1_SelectedIndexChanged(sender As Object, e As EventArgs) _
Handles ListBox1.SelectedIndexChanged
PictureBox1.Image = Image.FromFile(ListBox1.SelectedItem.ToString)
PictureBox1.SizeMode = PictureBoxSizeMode.StretchImage
End Sub
End Class
Points of Interest
I hope this tip will be of help to people wishing to become familiar with TIFFs. Remember the size of the final TIFF image depends on the sum of all the images used, the smaller they are, the lower the final weight.
History
- 21st November, 2021: First release and this article