This tip demonstrates the simplest and shortest piece of code required to connect you .NET Web App to Application Insights hosted in localhost or Azure Web App.
Introduction
This tip demonstrates the simplest and shortest piece of code required to connect your .NET Web App to Application Insights, both from localhost and Azure Web App.
Using the Code
Right click on the following: Project >> Add >> Application Insights Telemetry
Microsoft.ApplicationInsights.AspNetCore
NuGet package will get added as Dependencies.
Update the package to the latest version if not added automatically.
Add the Application Insight Connection String to the appsettings.json file as follows:
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*",
"ApplicationInsights": {
"ConnectionString": "Add App Insight Connection String Here"
}
}
Add the Application Insight Connection String to the Azure Web App Configuration as shown below. The key name should be the same.
P.S. Connection String is the recommended approach to connect to Application Insights instead of Instrumentation Key. Certain features are available in Application Insights only when connected through connection string.
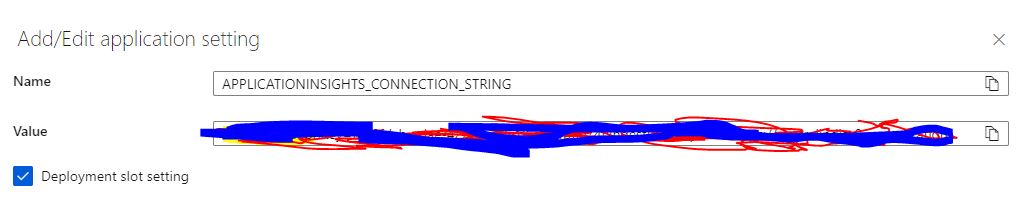
Add the following code to Program.cs file:
public class Program
{
public static void Main(string[] args)
{
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddApplicationInsightsTelemetry();
builder.Services.AddControllersWithViews();
var app = builder.Build();
if (!app.Environment.IsDevelopment())
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
app.Run();
}
}
How to Test the Above Approach?
- Create two Application Insight instances.
AppIns_1
and App_Ins_2
. - Add the connection string for
AppIns_1
in appsettings.json. - Add the connection string for
AppIns_2
in Azure Web App. - Build and execute the application in localhost, browse a few pages.
- Deploy the application in Azure Web App and browse a few pages.
- The logs from localhost will be found in
AppIns_1
- The logs from Azure Web App will be found in
AppIns_2
- Please note that there is a slight delay in the logs getting reflected in App Insights.
History
- 14th July, 2023: Initial version