Learn how to export CanvasJS charts to PDF using Puppeteer. This guide covers project setup, chart creation, and automated PDF generation for consistent, secure, and efficient reporting.
Introduction
Exporting web pages with dynamic charts to PDF can be a valuable feature for reporting and sharing data. In this guide, we'll walk you through the process of exporting a web page containing CanvasJS charts to a PDF file using Puppeteer, a Node.js library that provides a high-level API to control headless Chrome or Chromium.
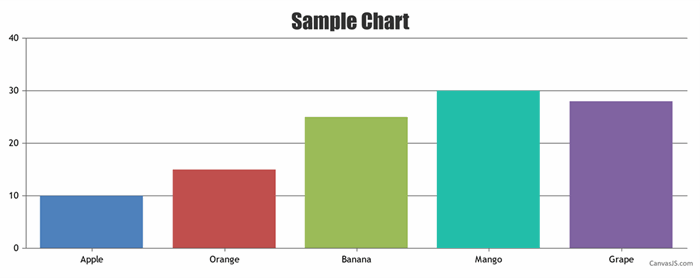
Background
Saving HTML as PDF on the server side offers several benefits:
1. Consistency and Control: By generating PDFs on the server, you ensure that the output is consistent across different devices and browsers. This is crucial for maintaining the integrity of reports and documents.
2. Automation: Server-side PDF generation can be automated, allowing for scheduled reports and batch processing. This is particularly useful for generating periodic reports without manual intervention.
3. Security: Sensitive data can be processed and converted to PDF on the server without exposing it to the client side. This reduces the risk of data breaches and ensures compliance with data protection regulations.
4. Performance: Offloading the PDF generation to the server can improve the performance of client-side applications, especially when dealing with large datasets or complex charts. This ensures a smoother user experience.
5. Integration: Server-side PDF generation can be easily integrated with other backend services, such as email servers for sending reports, or cloud storage for archiving documents.
Step-by-Step Tutorial
Step 1: Setting Up Your Project
First, create a new directory for your project and navigate into it:
mkdir canvasjs-to-pdf
cd canvasjs-to-pdf
Initialize a new Node.js project:
npm init -y
Install Puppeteer:
npm install puppeteer
Step 2: Creating the HTML Page
Create an index.html file with a CanvasJS chart:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>CanvasJS to PDF</title>
<script src="https://cdn.canvasjs.com/canvasjs.min.js"></script>
</head>
<body>
<div id="chartContainer" style="height: 370px; width: 100%;"></div>
<script>
window.onload = function () {
var chart = new CanvasJS.Chart("chartContainer", {
title: {
text: "Sample Chart"
},
data: [{
type: "column",
dataPoints: [
{ label: "Apple", y: 10 },
{ label: "Orange", y: 15 },
{ label: "Banana", y: 25 },
{ label: "Mango", y: 30 },
{ label: "Grape", y: 28 }
]
}]
});
chart.render();
}
</script>
</body>
</html>
Step 3: Writing the Puppeteer Script
Create a generate-pdf.js file to handle the PDF generation:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto(`file://${__dirname}/index.html`, { waitUntil: 'networkidle0' });
await page.pdf({ path: 'canvasjs-chart.pdf', format: 'A4' });
await browser.close();
})();
Step 4: Running the Script
Run the script to generate the PDF:
node generate-pdf.js
This will create a canvasjs-chart.pdf file in your project directory containing the rendered CanvasJS chart.
Points of Interest
The fun part was seeing Puppeteer magically capture the chart as a PDF after figuring out the waitUntil: 'networkidle0' trick. It felt like automating a mini printing press for charts—definitely a clever way to skip manual effort!
Download canvasjs-to-pdf.zip