Summary
This paper
would provide a small code snippet which will report all the files from VSS
(Visual SourceSafe 6.0) with shared reference instances. It will be useful for
migrating files from VSS to TFS.
Target Audience
This paper is
targeted at software developers with VB.NET and Visual SourceSafe 6.0 skills.
Introduction
VSS is one of
the key Microsoft products as a source code repository. Though the later
versions (Team Foundation Server) introduced on long back, still plenty of
source codes exist in VSS. Organizations are trying to migrate these codes to TFS.
There are
some core differences between VSS and TFS and one of them is shared references.
VSS allows files that can be shared across different folders/hierarchies. Through
this, a file can be shared between multiple folders/sub projects. So any
changes to a file can be reflected to all the references.
But TFS does
not allow direct shared (linked) references. Instead it suggests for a branching mechanism.
In branching, each instance of the file will be moved to individual
projects/folders. In order to move with branching for VSS codes, the old
shared reference files in VSS needs to be identified and altered. So if a
project contains multiple shared files with n number of references, it will be
hard to keep track of all the shared references. However, VSS provides an automation
mechanism through which the developer can identify all the shared references
and plan for a better migration.
Code
snippet to find out all the shared instances in VSS
The following
code snippet is written in VB.NET and the targeted framework is .NET 2.0.
It requires an interop library. The code uses Excel Interop for reporting purposes.
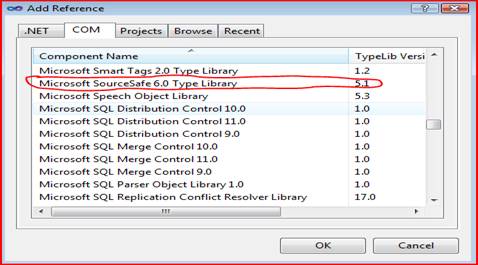
Imports SourceSafeTypeLib
Imports Microsoft.Office.Interop.Excel
Public Class frm_vss_files
Private Sub cmd_getdata_Click(sender As System.Object, e As System.EventArgs) Handles cmd_getdata.Click
_vss_ini_path = txt_ini_path.Text (LIKE "C:\VSS CODE$\SRCSAFE.INI")
_input_path = txt_root_path.Text (LIKE "$/ROOT/ABC")
_uid = txt_uid.Text (user name)
_pwd = txt_pwd.Text (password)
_chk_linked_files = chk_get_linked_files.Checked (Flag to identify shared files)
_i_xl_rw_err = 2
obj_vss_db.Open(_vss_ini_path, _uid, _pwd)
obj_vss_db.CurrentProject = _input_path
Try
obj_root = obj_vss_db.VSSItem(_input_path & "/", False)
Catch ex As Exception
MsgBox("SourceSafe DB access error!" + vbCrLf + ex.Message)
End Try
obj_excel.Visible = True
oWB = obj_excel.Workbooks.Add
oWB.Sheets(1).Cells(1, COL_FILE_NAME).Value = "File "
oWB.Sheets(1).Cells(1, COL_FOLDER_NAME).Value = "Folder"
oWB.Sheets(1).Cells(1, COL_VSS_PATH).Value = "VSS PATH"
oWB.Sheets(1).Cells(1, COL_SHARED).Value = "SHARED"
oWB.Sheets(1).Cells(1, COL_LINKS).Value = "Shared Paths"
oWB.Sheets(2).Cells(1, COL_ERR_FILE_NAME) = "File"
oWB.Sheets(2).Cells(1, COL_ERR_FOLDER_NAME) = "Folder"
oWB.Sheets(2).Cells(1, COL_ERR_VSS_PATH) = "VSS PATH"
oWB.Sheets(2).Cells(1, COL_ERR_LINKED_PATH) = "SHARED FILE PATH"
oWB.Sheets(2).Cells(1, COL_ERR_DESC) = "ERROR DESC"
oWB.Sheets(2).Name = "Error Details"
oWS = oWB.Worksheets(1)
_i_xl_rw = 2
Call get_sub_items(obj_root)
MsgBox("Completed successfully!", vbInformation, "")
End Sub
Private obj_vss_db As New VSSDatabase
Private obj_root As VSSItem
Private _vss_ini_path As String
Private _input_path As String
Private _uid As String
Private _pwd As String
Private _chk_linked_files As Boolean = False
Private obj_excel As New Microsoft.Office.Interop.Excel.Application
Private ows As New Worksheet
Private owb As Workbook
Private _i_xl_rw As Integer
Private _i_xl_rw_err As Integer
Const COL_FILE_NAME = 1
Const COL_FOLDER_NAME = 2
Const COL_VSS_PATH = 3
Const COL_SHARED = 4
Const COL_LINKS = 5
Const COL_ERR_FILE_NAME = 1
Const COL_ERR_FOLDER_NAME = 2
Const COL_ERR_VSS_PATH = 3
Const COL_ERR_LINKED_PATH = 4
Const COL_ERR_DESC = 5
Sub get_sub_items(root_item As VSSItem)
Dim ProjectItem As VSSItem
Dim strLinkList As String = ""
Dim sShared As Boolean
If root_item.Type = VSSItemType.VSSITEM_PROJECT Then
For Each ProjectItem In root_item.Items
Call get_sub_items(ProjectItem)
Next
Else
If root_item.Type = VSSItemType.VSSITEM_FILE Then
Dim objVSSLinkItem As VSSItem
Dim LinkCount As Integer
strLinkList = ""
LinkCount = 0
sShared = False
If _chk_linked_files = True Then
For Each objVSSLinkItem In root_item.Links
LinkCount = LinkCount + 1
If LinkCount = 2 Then sShared = True
On Error Resume Next
strLinkList = strLinkList + objVSSLinkItem.Parent.Spec + vbCrLf
If Err.Number = -2147166577 Then
owb.Sheets(2).Cells(_i_xl_rw_err, COL_ERR_FILE_NAME) = root_item.Name
owb.Sheets(2).Cells(_i_xl_rw_err, COL_ERR_FOLDER_NAME) = root_item.Parent.Name
owb.Sheets(2).Cells(_i_xl_rw_err, COL_ERR_VSS_PATH) = root_item.Spec
owb.Sheets(2).Cells(_i_xl_rw_err, COL_ERR_LINKED_PATH) = objVSSLinkItem.Spec
owb.Sheets(2).Cells(_i_xl_rw_err, COL_ERR_DESC) = Err.Description
_i_xl_rw_err = _i_xl_rw_err + 1
End If
Next
End If
End If
On Error Resume Next
ows.Cells(_i_xl_rw, COL_FILE_NAME).Value = root_item.Name
ows.Cells(_i_xl_rw, COL_FOLDER_NAME).Value = root_item.Parent.Spec
ows.Cells(_i_xl_rw, COL_VSS_PATH).Value = root_item.Spec
If sShared = True Then
ows.Cells(_i_xl_rw, COL_SHARED).Value = "SHARED"
ows.Cells(_i_xl_rw, COL_LINKS).Value = strLinkList
End If
_i_xl_rw = _i_xl_rw + 1
End If
End Sub
End Class
Conclusion
This paper is intended to provide a basic automation call for VSS objects to get shared references. Through these automation calls, developers can save time and increase productivity.