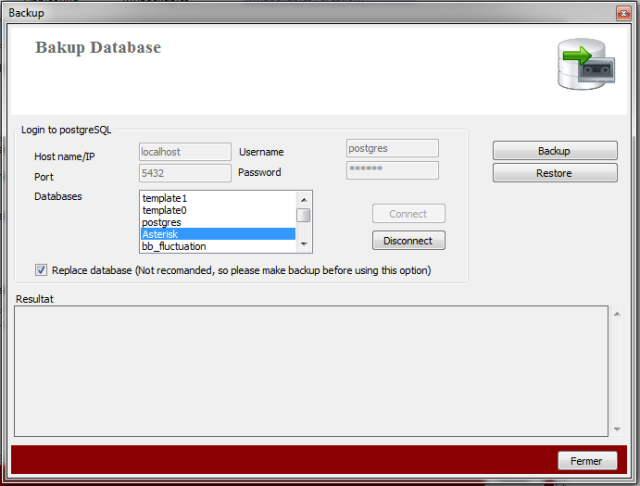
Introduction
This article provides developers the possibility to quick implement « Backup/Restore database » functionality in their applications. This library can be used from your application, and the backup operation runs in the local machine. It is not necessary to run backup or restore on the server.
Background
This library use some PostgreSQL files:
- pg_dump.exe
- pg_restore.exe
- libeay32.dll
- libiconv-2.dll
- libintl-8.dll
- libpq.dll
- Npgsql.dll
- ssleay32.dll
- zlib1.dll
In our case this solution
is tested on PostgreSQL 9.1. So if you have to use a different version, may be you
need to replace files with the correct version which could be found on your
PostgreSQL installation directory.
The backup/restore
operation is launched in NoShell mode and the result of the execution is intercepted
in an Output event which provides an output string.
Using the code
To launch Backup or Restore
operation, you have to fill a config object that contains the host, username,
port, and password. All public methods are written
in a static class named Control
.
To start backup, use the
method below:
void BackUp()
{
pgstore.Config cnf = new Config();
cnf.ServerName = txtHost.Text;
if (lstDB.Items.Count > 0 && lstDB.SelectedItem!=null)
cnf.DataBase = lstDB.SelectedItem.ToString();
cnf.UserName = txtUserName.Text;
cnf.Password = txtPass.Text;
cnf.Port = Convert.ToInt16(txtPort.Text);
pgstore.Control.CurrentConfig = cnf;
string FileName = pgstore.Control.CurrentConfig.DataBase + "_" + DateTime.Now.Day.ToString() +
"_" + DateTime.Now.Month.ToString() + DateTime.Now.Year.ToString() + ".backup";
pgstore.Control.Backup("D:\\backup" , FileName, true);
}
To start restore, use the method below:
void Restore(string path)
{
pgstore.Config cnf = new Config();
cnf.ServerName = txtHost.Text;
if (lstDB.Items.Count > 0 && lstDB.SelectedItem!=null)
cnf.DataBase = lstDB.SelectedItem.ToString();
cnf.UserName = txtUserName.Text;
cnf.Password = txtPass.Text;
cnf.Port = Convert.ToInt16(txtPort.Text);
pgstore.Control.CurrentConfig = cnf;
pgstore.Control.Restaure(path, false);
}
If you have a specific option, the right way is to change it in the StoreParameters
object in your
CurrentConfig.Parametres
.
Don’t forget to add the required PostgreSQL files to your setup project.