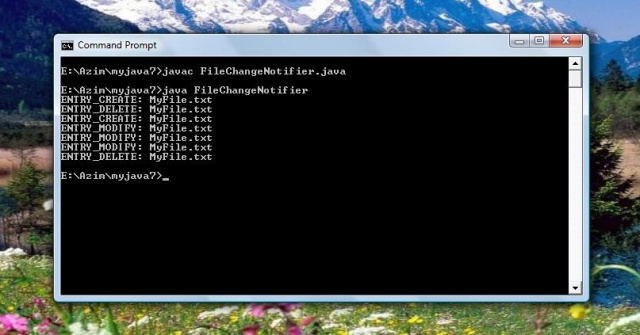
Introduction
File Change Notification is a new feature added in JDK 7. It is a part of the
new file system API (NIO 2.0). It uses the WatchService API to receive file change notification events like creation, modification, and deletion of files or directories.
Background
Following are the steps required to monitor the notification events:
- Create a
WatchService
as follows:
WatchService service = FileSystems.getDefault().newWatchService();
Get a reference of the file or directory to be monitored as follows:
Path path = Paths.get(dir);
Register the file/directory with the WatchService
, specifying the events to be monitored (Create, Modify, Delete) as follows:
path.register(watchService,StandardWatchEventKinds.ENTRY_CREATE,
StandardWatchEventKinds.ENTRY_MODIFY,
StandardWatchEventKinds.ENTRY_DELETE);
Create an infinite loop to monitor the events using the take()
method to retrieve a watchkey placed into the watch service's queue when an event occurs as follows:
while(true)
{
WatchKey key = service.take();
...
}
Inside the infinite loop, use another loop to print all events as follows: If the directory becomes inaccessible, the key will become invalid and the loop will be exitted.
for (WatchEvent event : key.pollEvents())
{
System.out.println(event.kind() + ": "+ event.context());
}
boolean valid = key.reset();
if (!valid)
{
break;
}
Using the code
Following is the complete java code:
import java.nio.*;
import java.io.*;
import java.nio.file.StandardWatchEventKinds.*;
import java.nio.file.*;
public class FileChangeNotifier
{
public static void main(String args[]) throws IOException, InterruptedException
{
watchDir("E:\\MyFolder");
}
public static void watchDir(String dir) throws IOException, InterruptedException
{
WatchService service = FileSystems.getDefault().newWatchService();
Path path = Paths.get(dir);
path.register(service,
StandardWatchEventKinds.ENTRY_CREATE,
StandardWatchEventKinds.ENTRY_MODIFY,
StandardWatchEventKinds.ENTRY_DELETE);
while(true)
{
WatchKey key = service.take();
for (WatchEvent event : key.pollEvents())
{
System.out.println(event.kind() + ": "+ event.context());
}
boolean valid = key.reset();
if (!valid)
{
break;
}
}
}
}
Points of Interest
The File Change Notification feature provides a simple and elegant way of monitoring file notification events.