Introduction
In this tip, I provide a Sharepoint webpart solution developed using Visual Studio 2010. It contains a Sharepoint Treeview webpart that displays site collections and subsites based on permissions applied on these sites.
When a user is logged in to the page, he is going to view the sites and subsites of all site collections - that he has permission on it - in one place then when the user clicks on one of the titles, he will be navigated to the site in a new page.
Main Requirements
- The Tree View will display the sites titles based on permission of the logged in user.
- A user with Permission from Read only to Administration can click on the Title of the Site to navigate
- The site will be displayed in a new window.
- A user with no permission on a specific site will not be able to see the link.
- No configurations are required, can be added to any site at any level in a specific webapplication.
ScreenShot
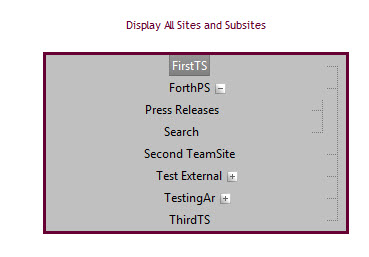
Deployment
- The solution will be deployed on site level (scope)
- A user control will be added in 14% in this location:
C:\Program Files\Common Files\Microsoft Shared\Web Server Extensions\14\TEMPLATE\CONTROLTEMPLATES\TreeSitesViwr\SitesViwrWP\ SitesViwrWPUserControl.ascx - A solution Assembly DLL will be deployed in the GAC (TreeSitesViwr.dll) C:\windows\assembly\GAC_msil\TreeSitesViwr\1.0.0.0__19d8525006072941
- A resource file will be added in the
App_GlobalResources
(SitesViwrRes .resx). - 2 DLLs will be added in the bin folder of the web application (Telerik.Web.Design.dll) and (Telerik.Web.UI.dll)
- Source control will also be added to the web.config file.
<SafeControl Assembly="TreeSitesViwr, Version=1.0.0.0, Culture=neutral,
PublicKeyToken=19d8525006072941" Namespace="TreeSitesViwr.SitesViwrWP"
TypeName="*" Safe="True" SafeAgainstScript="False" />
- Feature name is TreeSitesViwr Feature
Solution
- WSP file (TreeSitesViwr.wsp) (Download SitesViwrWP)
- Visual WebPart contains user control and code behind (Download TreeSitesViwr)
Code
First UserControl Design:
- Register Telerik Assemblies:
<%----%>
<%@ Register assembly="Telerik.Web.UI" namespace="Telerik.Web.UI" tagprefix="telerik" %>
<%@ Register assembly="Telerik.Web.Design" namespace="Telerik.Web.UI.Design"
tagprefix="telerik" %>
Add RadTreeView
control and define its properties:
<telerik:RadTreeView runat="server" ID="SiteTV" BackColor="Silver"
BorderColor="#660033" BorderStyle="Solid" ForeColor="Black" Width="300px">
</telerik:RadTreeView>
In the code behind, add these functions:
- In the
Page_Load
:
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BuildSiteTree();
}
SiteTV.CollapseAllNodes();
}
- Add this function
BuildSiteTree( )
:
private void BuildSiteTree()
{
int CountSites = 0;
int Count_No_perm = 0;
SPWebApplication WebApp = SPContext.Current.Web.Site.WebApplication;
SPSiteCollection TScoll = WebApp.Sites;
SPSecurity.RunWithElevatedPrivileges(delegate()
{
try
{
foreach (SPSite TSite in TScoll)
{
try
{
if (CountSites != 0)
{
bool hasperm = UserHasPerm(TSite.Url.ToString());
RadTreeNode rootNode = new RadTreeNode
(TSite.RootWeb.Title, TSite.Url);
rootNode.NavigateUrl = TSite.Url;
rootNode.Target = "_blank";
if (UserHasPerm(TSite.Url.ToString()))
{
SiteTV.Nodes.Add(rootNode);
}
using (SPWeb web = TSite.OpenWeb())
{
try
{
foreach (SPWeb subSite in web.Webs)
{
if (UserHasPerm(subSite.Url.ToString()))
{
try
{
AddNodes(subSite, rootNode.Nodes);
Count_No_perm = Count_No_perm + 1;
}
catch
{
throw;
}
finally
{
if (subSite != null)
subSite.Dispose();
}
}
}
}
catch
{
continue;
}
}
}
CountSites = CountSites + 1;
}
catch
{
continue;
}
}
if (Count_No_perm == 0)
{
WPpnl.Visible = false;
lblError.Visible = true;
lblError.Text = HttpContext.GetGlobalResourceObject
("SitesViwrRes", "NoSites").ToString();
}
}
catch
{
throw;
}
});
}
- Also add this function
UserHasPerm( )
for authentication check and it will return bool
value T
if the logged in user has permission and F
if not:
private bool UserHasPerm(string TSite)
{
SPWeb web = new SPSite(TSite).OpenWeb();
bool hasperm = web.DoesUserHavePermissions
(SPContext.Current.Web.CurrentUser.LoginName, SPBasePermissions.ViewPages);
return hasperm;
}