Introduction
Though it may seem easy to set an image as a wallpaper, there are various small issues that need to be addressed to correctly set a wallpaper.
Through a simple app - Walltoday which allows a user to set a wallpaper for each day which changes automatically every morning,
this tip shows a number of points which will help in setting the wallpaper.
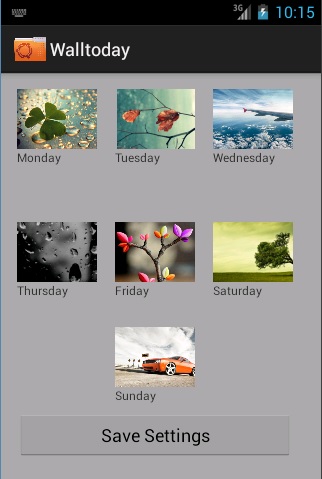
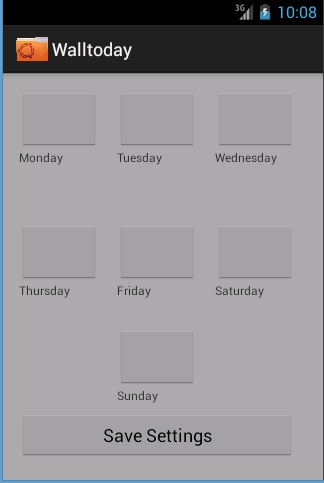
Background
The app can be downloaded via Google play store using the following links:
- market://details?id=com.app.walltoday
or:
Using the Code
1. Activity
Start activity to select an image from Image Gallery:
Intent intent = new Intent(Intent.ACTION_PICK,
android.provider.MediaStore.Images.Media.INTERNAL_CONTENT_URI);
startActivityForResult(intent, RESULT_LOAD_IMAGE);
Set the selected image from gallery:
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
try {
if (requestCode == RESULT_LOAD_IMAGE &&
resultCode == RESULT_OK && null != data)
{
Uri selectedImage = data.getData();
String[] filePathColumn = { MediaStore.Images.Media.DATA };
Cursor cursor = getContentResolver().query(
selectedImage,filePathColumn, null, null, null);
cursor.moveToFirst();
int columnIndex = cursor.getColumnIndex(filePathColumn[0]);
String picturePath = cursor.getString(columnIndex);
cursor.close();
ImageButton imgButton = (ImageButton) findViewById(ButtonIdToSet);
File file = new File(picturePath);
if(file.exists())
{
Bitmap bmp = decodeFile(file,
imgButton.getWidth(),imgButton.getHeight());
imgButton.setImageBitmap(bmp);
}
}
} catch (Exception e) {
e.printStackTrace();
}
}
2. Working with Bitmaps in Android
Resize (scale) bitmap
for given Height
and Width
:
public static Bitmap decodeFile(File file, int reqWidth, int reqHeight){
final BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true;
BitmapFactory.decodeFile(file.getPath(), options);
options.inSampleSize = calculateInSampleSize(options, reqWidth, reqHeight);
options.inJustDecodeBounds = false;
return BitmapFactory.decodeFile(file.getPath(), options);
}
private static int calculateInSampleSize(
BitmapFactory.Options options, int reqWidth, int reqHeight) {
final int height = options.outHeight;
final int width = options.outWidth;
int inSampleSize = 1;
if (height > reqHeight || width > reqWidth) {
final int heightRatio = Math.round((float) height / (float) reqHeight);
final int widthRatio = Math.round((float) width / (float) reqWidth);
inSampleSize = heightRatio < widthRatio ? heightRatio : widthRatio;
}
return inSampleSize;
}
3. Get height
and width
of display before setting the bitmap
:
DisplayMetrics metrics = appContext.getResources().getDisplayMetrics();
int height = metrics.heightPixels;
int width = metrics.widthPixels;
4. Set the bitmap
:
Bitmap bmp = decodeFile(file,width,height);
myWallpaperManager.suggestDesiredDimensions(width, height);
myWallpaperManager.setBitmap(bmp);