Introduction
In search engines, we often see the text/string we are searching for comes highlighted in the search result. In this tip, we will learn how to highlight the search text in search result.
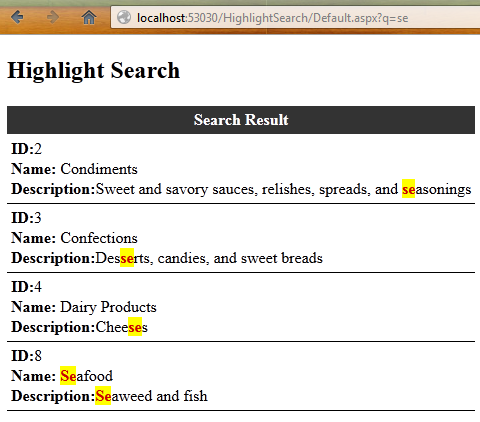
(Image:1)
Background
Before going through this tip, you should have basic knowledge of C# , ASP.NET Gridview
control and how to use template field in Gridview
.
Description
Let's create a GridView
in our page:
<asp:GridView ID="GridView1"
runat="server" AutoGenerateColumns="False"
BackColor="White" BorderColor="#CCCCCC"
BorderStyle="None" BorderWidth="1px"
CellPadding="4" EmptyDataText="No Record Found" ForeColor="Black"
GridLines="Horizontal">
<Columns>
<asp:TemplateField HeaderText="Search Result">
<ItemTemplate>
<strong>ID:</strong>
<%#Eval("CategoryID")%><br /><strong>
Name:</strong> <asp:Label ID="Label1" runat="server"
Text='<%# ModifyString(Container.DataItem,
"CategoryName") %>'></asp:Label><br />
<strong>Description:</strong><asp:Label ID="Label2"
runat="server" Text='<%# ModifyString
(Container.DataItem,"Description") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
</Columns>
<FooterStyle BackColor="#CCCC99" ForeColor="Black" />
<HeaderStyle BackColor="#333333"
Font-Bold="True" ForeColor="White" />
<PagerStyle BackColor="White"
ForeColor="Black" HorizontalAlign="Right" />
<SelectedRowStyle BackColor="#CC3333"
Font-Bold="True" ForeColor="White" />
<SortedAscendingCellStyle BackColor="#F7F7F7" />
<SortedAscendingHeaderStyle BackColor="#4B4B4B" />
<SortedDescendingCellStyle BackColor="#E5E5E5" />
<SortedDescendingHeaderStyle BackColor="#242121" />
</asp:GridView>
Here two things are important:
<%# ModifyString(Container.DataItem,"CategoryName") %>
<%# ModifyString(Container.DataItem,"Description") %>
ModifyString
method is used to bind string
from codebehind file to the Label
controls.
We can define the ModifyString
method in codebehind as follows:
protected string ModifyString(object dataItem,string Field)
{
string val = string.Empty;
string data = string.Empty;
data = DataBinder.Eval(dataItem, Field).ToString();
if (Request.QueryString["q"] != null)
{
val = Request.QueryString["q"].ToString();
data = HighlighText(data, val);
}
return data;
}
We have used two methods HighlighText
to replace the search text from our output string
ignoring the case.
private string HighlighText(string Source, string SearchText)
{
return Regex.Replace(Source, SearchText,
new MatchEvaluator(ReplaceKeyWords), RegexOptions.IgnoreCase);
}
public string ReplaceKeyWords(Match m)
{
string srcstring = @"http://www.google.com/?q=" + m.Value +
"&output=search#gs_rn=22&gs_ri=psy-ab&
tok=I8yrcWFtnI_HqIsJurjImw&pq=" +
m.Value + "&cp=11&gs_id=st&xhr=t&q=" + m.Value +
"&es_nrs=true&pf=p&safe=off&
sclient=psy-ab&oq=" + m.Value +
"&gs_l=&pbx=1&bav=on.2,or.r_cp.r_qf.&bvm=bv.49784469,
d.bmk&fp=4d30aa4c9d6b1c2c&biw=1366&bih=676";
string tagstring = @"<a target='_blank' class='srcLink'
title='Search with google' href='" +srcstring + "'>";
return tagstring+"<span class='src'>" + m.Value + "</span></a>";
}
We are replacing the search string with a span
with class src
tag and providing Google search link in our highlighted string
. For that, we have used these two following lines of code:
string srcstring = @"http://www.google.com/?q=" + m.Value +
"&output=search#gs_rn=22&gs_ri=psy-ab&tok=I8yrcWFtnI_HqIsJurjImw&pq=" + m.Value +
"&cp=11&gs_id=st&xhr=t&q=" + m.Value +
"&es_nrs=true&pf=p&safe=off&sclient=psy-ab&oq=" +
m.Value + "&gs_l=&pbx=1&bav=on.2,or.r_cp.r_qf.&bvm=bv.49784469,
d.bmk&fp=4d30aa4c9d6b1c2c&biw=1366&bih=676";
string tagstring = @"<a target='_blank' class='srcLink' title='Search with google' href='" +
srcstring + "'>";
Let's create our CSS style to format our highlighted string
:
<style type="text/css">
.src
{
background-color: #FFFF00;
font-weight: bold;
color: #CC0000;
}
.srcLink
{
font-weight: bold;
text-decoration:none;
}
.srcLink:hover
{
font-weight: bold;
text-decoration:underline;
}
</style>
Here is the whole code in our codebehind file:
protected void Page_Load(object sender, EventArgs e)
{
string val=string.Empty;
if(!IsPostBack)
{
if (Request.QueryString["q"] != null)
{
val = Request.QueryString["q"].ToString();
}
GridView1.DataSource =GetDataBySearch(val);
GridView1.DataBind();
}
}
IQueryable GetDataBySearch(string SrcString)
{
NORTHWINDEntities nwObj = new NORTHWINDEntities();
var qry = (from ep in nwObj.Categories where (ep.Description.Contains(SrcString)||
ep.CategoryName.Contains(SrcString))
select new { ep.CategoryID, ep.CategoryName, ep.Description });
return qry;
}
protected string ModifyString(object dataItem,string Field)
{
string val = string.Empty;
string data = string.Empty;
data = DataBinder.Eval(dataItem, Field).ToString();
if (Request.QueryString["q"] != null)
{
val = Request.QueryString["q"].ToString();
data = HighlighText(data, val);
}
return data;
}
private string HighlighText(string Source, string SearchText)
{
return Regex.Replace(Source, SearchText,
new MatchEvaluator(ReplaceKeyWords), RegexOptions.IgnoreCase);
}
public string ReplaceKeyWords(Match m)
{
string srcstring = @"http://www.google.com/?q=" + m.Value +
"&output=search#gs_rn=22&gs_ri=psy-ab&
tok=I8yrcWFtnI_HqIsJurjImw&pq=" +
m.Value + "&cp=11&gs_id=st&xhr=t&q=" + m.Value +
"&es_nrs=true&pf=p&safe=off&sclient=psy-ab&oq=" + m.Value +
"&gs_l=&pbx=1&bav=on.2,or.r_cp.r_qf.&bvm=bv.49784469,
d.bmk&fp=4d30aa4c9d6b1c2c&biw=1366&bih=676";
string tagstring = @"<a target='_blank' class='srcLink'
title='Search with google' href='" +
srcstring + "'>";
return tagstring+"<span class='src'>" + m.Value +
"</span></a>";
}
I have used EntityFrameWork
here to bind data to Gridview
, no matter you can follow any other way to bind data to Gridview
. That's it, we can get our search string highlighted in our Gridview
, just pass any value through Query String "q
" as shown in (Image:1) above.
Conclusion
Thank you for reading this tip. Please comment and make some suggestions to make this tip more interesting.