Introduction
I want to make a very simple Server/Client application. Microsoft offers a great tool called Windows Communication Foundation (WCF) for communication between Server and Clients. I am trying to make a short introduction of WCF, but if you interested to know more, check out this link on MSDN for all details.
WCF has two parts:
- Services
- Clients
1) Services
Service has one or more endpoints. Endpoint means where message can receive or deliver. Service consists of a set of operations. Every service needs to host. There are four ways we can host and activate services:
- Windows Activation Service (WAS)
- As executables (.EXE), also called self-hosted service.
- Windows service
- COM+ components can also be hosted as WCF services
I am going to give a self-hosted service example here.
Self-Hosted Service has three parts to become live.
- Define a service contract
- Implement a service contract
- Host and run a service contract
2) Clients
WCF Clients is an application which can communicate with WCF Service operations as method.
Communicate with self-hosted service from WCF Clients has three steps:
- Create a WCF Client
- Configure a WCF Client
- Use a WCF Client
Background
I Googled and found lots of tutorials on WCF web hosting. Also most of them are in C#. Too much discussion on the technology. My aim is to make a WCF self-hosted service using VB.NET with a very basic example.
Using the Code
To make a WCF Service: Open Visual Studio 2010
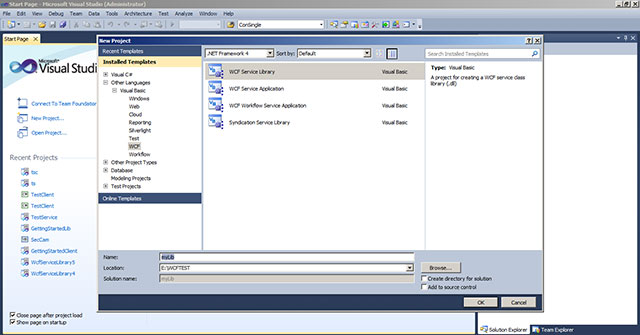
Create a new WCF Service Library project. Name: myLib

Right mouse click on project name and select Add Reference.

Select System.ServiceModel
and click OK.
1) Define a service contract
Now Open Service1.vb from Solution Explorer and copy this code:
Imports System
Imports System.ServiceModel
Public Class Service1
Implements IService1
Public Function GetName(ByVal value As String) As String Implements IService1.GetData
Console.WriteLine("Hello {0}", value)
Return String.Format("Hello {0}", value)
End Function
End Class
2) Implement a service contractOpen
IService1.vb from Solution Explorer and copy this code:
Imports System
Imports System.ServiceModel
<ServiceContract()>
Public Interface IService1
<OperationContract()>
Function GetData(ByVal value As String) As String
End Interface
3) Host and run a service contract

Add a new project to myLib
Project:

Select Console Application to display Host output. Name: wcHost

Right mouse click on wcHost
project and select Add Reference.

From Add Reference Projects, select myLib
and click OK.

Again right mouse click on wcHost
project and select Add Reference.

Select System.ServiceModel
and click OK.
Open Module1.vb from Solution Explorer and copy this code:
Imports System
Imports System.ServiceModel
Imports System.ServiceModel.Description
Imports myLib.Service1
Module Module1
Sub Main()
Dim baseAddress As New Uri("http://localhost:8000/SayHelloService")
Dim selfHost As New ServiceHost(GetType(myLib.Service1), baseAddress)
Try
selfHost.AddServiceEndpoint(GetType(myLib.IService1), _
New WSHttpBinding(), "HelloService")
Dim smb As New ServiceMetadataBehavior()
smb.HttpGetEnabled = True
selfHost.Description.Behaviors.Add(smb)
selfHost.Open()
Console.WriteLine("The service is ready.")
Console.WriteLine("Press <ENTER> to terminate service.")
Console.WriteLine()
Console.ReadLine()
selfHost.Close()
Catch ce As CommunicationException
Console.WriteLine("An exception occurred: {0}", ce.Message)
selfHost.Abort()
End Try
End Sub
End Module
Build wcHost
.
To make a WCF Client: Open Visual Studio 2010
1) Create a WCF Client

Create a new console application. Name: wcClient

Right mouse click on project name and select Add Reference.

Select System.ServiceModel
and click OK:

Right mouse click on project name and select Add Service Reference.

Put "http://localhost:8000/SayHelloService" in address.
Now run wcHost.exe, you can locate it in ..\wcHost\bin\Debug folder.
When wcHost
runs, then click go in add service reference window and wait.

When found Service1
then Click OK.
2)Configure a WCF ClientOpen wcClient app.config from Solution Explorer and copy this code:
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<system.diagnostics>
<sources>
<!-- This section defines the logging configuration for My.Application.Log -->
<source name="DefaultSource" switchName="DefaultSwitch">
<listeners>
<add name="FileLog"/>
<!-- Uncomment the below section to write to the Application Event Log -->
<!--<add name="EventLog"/>-->
</listeners>
</source>
</sources>
<switches>
<add name="DefaultSwitch" value="Information" />
</switches>
<sharedListeners>
<add name="FileLog"
type="Microsoft.VisualBasic.Logging.FileLogTraceListener, Microsoft.VisualBasic, Version=8.0.0.0, Culture=neutral, PublicKeyToken=b03f5f7f11d50a3a, processorArchitecture=MSIL"
initializeData="FileLogWriter"/>
<!-- Uncomment the below section and replace APPLICATION_NAME with the name of your application to write to the Application Event Log -->
<!--<add name="EventLog" type="System.Diagnostics.EventLogTraceListener" initializeData="APPLICATION_NAME"/> -->
</sharedListeners>
</system.diagnostics>
<system.serviceModel>
<bindings>
<wsHttpBinding>
<binding name="WSHttpBinding_IService1" />
</wsHttpBinding>
</bindings>
<client>
<endpoint address="http://localhost:8000/SayHelloService/HelloService"
binding="wsHttpBinding" bindingConfiguration="WSHttpBinding_IService1"
contract="ServiceReference1.IService1" name="WSHttpBinding_IService1">
<identity>
<userPrincipalName value="ERP001\Manager1" />
</identity>
</endpoint>
</client>
</system.serviceModel>
</configuration>
Note: Please change "ERP001\Manager1" according to your Server Computer user name in <identity> section of app.config file.
3) Use a WCF Client
Open wcClient
Module1.vb from Solution Explorer and copy this code:
Imports System
Imports System.ServiceModel
Imports wcClient.ServiceReference1
Module Module1
Dim Client As New Service1Client
Sub Main()
Console.WriteLine("Client Window")
Console.WriteLine("{0}", Client.GetData(Console.ReadLine()))
Client.Close()
Console.WriteLine()
Console.WriteLine("Press <ENTER> to terminate client.")
Console.ReadLine()
End Sub
End Module
Build and run wcClient.exe:
Enter "World" in wcClient
window.
Points of Interest
Visual Studio by default created two files service1.vb and Iservice1.vb when we create wcHost. If you rename the default file name or class name make sure you update all reference in entire solution. I use simple replace command from vs edit menu look into entire solution to replace "service" class name with "xxx" so that all the reference get updated. but you can do it manually. Just make sure you update all reference.