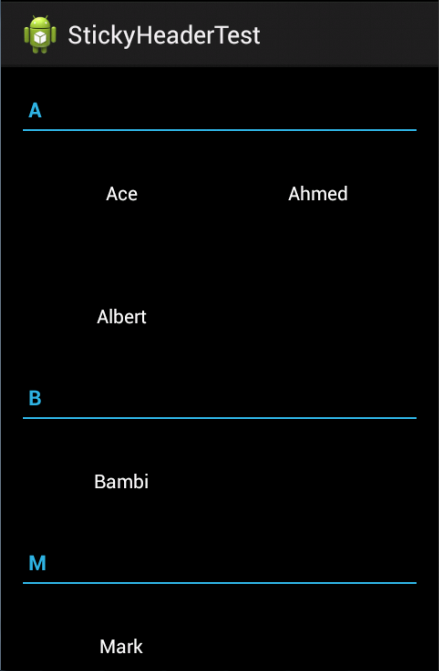
Introduction
I customized StickyGridHeaders
to group any type of data and group by any data.
Using the Code
I will explain every part of the code and classes that need to be changed in order to make it work as required.
We will change in the following classes:
StickyGridHeadersSimpleArrayAdapter
StickyGridHeadersSimpleAdapter
StickyGridHeadersSimpleWrapper
1. StickyGridHeadersSimpleArrayAdapter
- Let's get our class ready to hold our
ArrayList
data by changing the constructor and init()
functions.
private ArrayList<HashMap<String, String>> DataArray;
public StickyGridHeadersSimpleArrayAdapter(Context context,
int headerResId, int itemResId , ArrayList<HashMap<String, String>> data) {
init(context, headerResId, itemResId, data);
}
private void init(Context context, int headerResId,
int itemResId, ArrayList<HashMap<String, String>> data) {
this.mHeaderResId = headerResId;
this.mItemResId = itemResId;
this.DataArray = data;
mInflater = LayoutInflater.from(context);
}
- Change
getCount()
function to return the right count of our data ArrayList
.
@Override
public int getCount() {
return DataArray.size();
}
- Change
getItem()
function to return a specific Item of our data ArrayList
depending on position
parameter.
@Override
public HashMap<string> getItem(int position) {
return DataArray.get(position);
}
- Change
getHeaderId()
function to work with an element inside ArrayList
. In my example, it's "Name
" .
public CharSequence getHeaderId(int position) {
HashMap<string> item = getItem(position);
CharSequence value;
if (item.get("Name") instanceof CharSequence) {
value = (CharSequence) item.get("Name");
} else {
value = item.get("Name").toString();
}
return value.subSequence(0, 1);
}
- Change
getHeaderView()
function. One of the most important functions that will return the string
that will appear on the Header
.
@SuppressWarnings("unchecked")
public View getHeaderView(int position, View convertView, ViewGroup parent) {
HeaderViewHolder holder;
if (convertView == null) {
convertView = mInflater.inflate(mHeaderResId, parent, false);
holder = new HeaderViewHolder();
holder.textView = (TextView) convertView.findViewById(android.R.id.text1);
convertView.setTag(holder);
} else {
holder = (HeaderViewHolder) convertView.getTag();
}
HashMap<string> item = getItem(position);
CharSequence string;
if (item.get("Name") instanceof CharSequence) {
string = (CharSequence) item.get("Name");
} else {
string = item.get("Name").toString();
}
holder.textView.setText(string.subSequence(0, 1));
return convertView;
}
- Change
getView()
function. One of the most important functions that will return the data that will appear.
You can check More Information field below to change the header and data style or add an imageview
to display.
@Override
@SuppressWarnings("unchecked")
public View getView(int position, View convertView, ViewGroup parent) {
ViewHolder holder;
if (convertView == null) {
convertView = mInflater.inflate(mItemResId, parent, false);
holder = new ViewHolder();
holder.textView = (TextView) convertView.findViewById(android.R.id.text1);
convertView.setTag(holder);
} else {
holder = (ViewHolder) convertView.getTag();
}
HashMap<String, String> item = getItem(position);
if (item.get("Name") instanceof CharSequence) {
holder.textView.setText((CharSequence) item.get("Name"));
} else {
holder.textView.setText(item.get("Name").toString());
}
return convertView;
}
2. StickyGridHeadersSimpleAdapter
- Change the type of returned data from long to
CharSequence
.
CharSequence getHeaderId(int position);
3. StickyGridHeadersSimpleWrapper
- Change the type of "mapping" from
long
to CharSequence
.
protected HeaderData[] generateHeaderList(StickyGridHeadersSimpleAdapter adapter) {
Map<CharSequence, HeaderData> mapping = new HashMap<CharSequence, HeaderData>();
List<HeaderData> headers = new ArrayList<HeaderData>();
for (int i = 0; i < adapter.getCount(); i++) {
CharSequence headerId = adapter.getHeaderId(i);
HeaderData headerData = mapping.get(headerId);
if (headerData == null) {
headerData = new HeaderData(i);
headers.add(headerData);
}
headerData.incrementCount();
mapping.put(headerId, headerData);
}
return headers.toArray(new HeaderData[headers.size()]);
}
More Information
- You can change the Header Style from header.xml.
- You can change the item data to display from item.xml.
- You must include the
gridview com.example.stickyheadertest.StickyGridHeadersGridView
to get it work.
Thank you everyone for reading this tip, and hopefully it helps everyone out.