Introduction
The idea for writing this article came to mind when someone asked me for a solution. When I posted this question to CodeProject for a solution I didn’t get any proper solution.
The problem was, we have two tables: employee and department.
Employee Table:
Empid
| Name
| Designation
|
1
| AAA
| Hr
|
2
| BBB
| Se
|
3
| CCC
| Md
|
4
| DDD
| Hr
|
5
| EEE
| Se
|
Department Table:
DesignationId
| DesignationName
|
1
| Hr
|
2
| Se
|
3
| Md
|
|
|
You can download the solution which has the sample database.
Now we need to show the details of all employees in a gridview. You can see in the figure below that the Designation column shows values which come from the Designation column of the Employee table as well as all the values which can be set for this column which basically come from the Department table. This means
the dropdownlist’s SelectedValue
is the value which is actually the value of the Designation column for each employee.
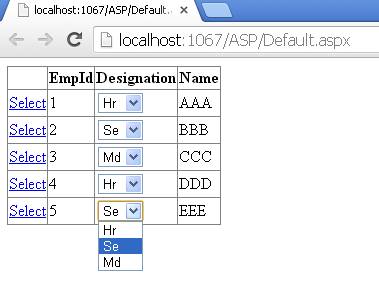
Background
This article includes an idea to add a control to a gridview dynamically to a column. The gridview also has a value from some datasource
and programmatically I need to add a dropdownlist to each and every row of the gridview with some values and there must be one selected value which may be different
for different rows. The added control should be available after postback and the control should maintain its value too. A user can change the value from the available option.
Design your page
So design your page as follows:
<form id="form1" runat="server">
<div>
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False"
DataKeyNames="EmpId" DataSourceID="SqlDataSource1"
ondatabound="GridView1_DataBound" >
<Columns>
<asp:CommandField ShowSelectButton="True" />
<asp:BoundField DataField="EmpId" HeaderText="EmpId" ReadOnly="True"
SortExpression="EmpId" />
<asp:BoundField HeaderText="Designation" />
<asp:BoundField DataField="Name" HeaderText="Name" SortExpression="Name" />
</Columns>
</asp:GridView>
<asp:SqlDataSource ID="SqlDataSource1" runat="server"
ConnectionString="<%$ ConnectionStrings:DatabaseConnectionString %>"
SelectCommand="SELECT * FROM [employee]"></asp:SqlDataSource>
</div>
</form>
Using the code
using System;
using System.Collections;
using System.Linq;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
using System.Data.SqlClient;
public partial class _Default : System.Web.UI.Page
{
SqlConnection con;
DataTable dt,dt2;
DataSet ds,ds2;
static Hashtable ht;
static ArrayList list;
static DropDownList dl;
protected void Page_Load(object sender, EventArgs e)
{
if (IsPostBack)
addControl();
else
{
con = new SqlConnection("Data Source=.\\SQLEXPRESS;AttachDbFilename=" +
"|Datadirectory|Database.mdf;Integrated Security=True;" +
"Connect Timeout=30;User Instance=True");
SqlCommand cmd=new SqlCommand("select * from desig",con);
SqlCommand cmd2 = new SqlCommand("select * from employee", con);
SqlDataAdapter da = new SqlDataAdapter(cmd);
SqlDataAdapter da2 = new SqlDataAdapter(cmd2);
ds = new DataSet();
ds2 = new DataSet();
da.Fill(ds);
da2.Fill(ds2);
dt = new DataTable();
dt2 = new DataTable();
dt=ds.Tables[0];
dt2 = ds2.Tables[0];
list = new ArrayList();
foreach (DataRow dr in dt.Rows)
{
list.Add(dr.ItemArray[1]);
}
ht = new Hashtable();
foreach (DataRow dr in dt2.Rows)
{
ht.Add(dr[0], dr[2]);
}
}
}
void addControl()
{
foreach (GridViewRow row in GridView1.Rows)
{
int key =(int) GridView1.DataKeys[row.RowIndex].Value;
if (row.RowType == DataControlRowType.DataRow)
{
dl = new DropDownList();
dl.DataSource = list;
dl.DataBind();
string str=ht[key].ToString();
dl.SelectedValue = str;
row.Cells[2].Controls.Add(dl);
}
}
}
protected void GridView1_DataBound(object sender, EventArgs e)
{
addControl();
}
}
}
History
- 12 Nov. 2013: Initial version.