Introduction
As a software developer or a User Interface developer, you need to generate sample data, especially if you are going to develop web pages that contain GridViews. So you can view your web page before creating the database.
In this tip, I am going to show you how to make a configurable data generator class that can be used to generate data either for
a DataTable
or directly for a GridView
.
Before You Start
Before you use this library, you should know the following assumptions:
- This library is developed for web applications, if you want to use it in different types of applications you have the code
and you can change the parts which depend on
HttpContext
or GridView
. - The library creates an empty datatable for any
gridview
bound columns,
other types of columns will not be bound. - The library generates data for a
datatable
based on column
names that match the text file names, so you can change the file name or the column names to match.
Using the Code
Simply add the DataGenerator
class to your web application in the App_Code folder, and the SampleData folder which contains the sample data.
Build your GridView
normally:
<asp:GridView ID="GridView1"
runat="server" AutoGenerateColumns="False"
BackColor="White" BorderColor="#999999"
BorderStyle="None" BorderWidth="1px"
CellPadding="3" GridLines="Vertical" Width="80%">
<AlternatingRowStyle BackColor="#DCDCDC" />
<Columns>
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="Age" HeaderText="Age" />
<asp:BoundField DataField="Country" HeaderText="Country" />
<asp:BoundField DataField="City" HeaderText="City" />
<asp:BoundField DataField="Notes" HeaderText="Notes" />
</Columns>
<FooterStyle BackColor="#CCCCCC" ForeColor="Black" />
<HeaderStyle BackColor="#000084"
Font-Bold="True" ForeColor="White" />
<PagerStyle BackColor="#999999"
ForeColor="Black" HorizontalAlign="Center" />
<RowStyle BackColor="#EEEEEE" ForeColor="Black" />
<SelectedRowStyle BackColor="#008A8C"
Font-Bold="True" ForeColor="White" />
<SortedAscendingCellStyle BackColor="#F1F1F1" />
<SortedAscendingHeaderStyle BackColor="#0000A9" />
<SortedDescendingCellStyle BackColor="#CAC9C9" />
<SortedDescendingHeaderStyle BackColor="#000065" />
</asp:GridView>
Code-Behind (cs)
You will do this in two steps:
- Build a
DataTable
using the current gridview
using
the GetDatableSchema
method - Generate the sample data by sending the
datatable
as a parameter and
the number of required rows to be generated.
DataTable dt = DataGenerator.GetDataTableSchema(GridView1);
DataGenerator.GenerateSampleData(dt, 100);
GridView1.DataSource = dt;
GridView1.DataBind();
Configure Your Sample Data
Just open the text files in the /SampleData folder and edit the data, add new lines or remove any lines.
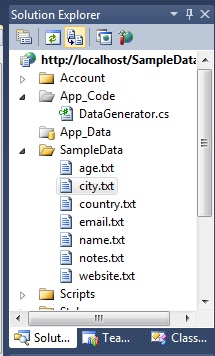
Now the Result

Coming Features
If you like the library, encourage me by sending ideas for more features to enhance the library for different types of controls, data, and languages.
Hazem Torab, founder & CEO at Enozom Software, a fast growing software company in Alexandria. Hazem has more than 6 years of experience in management and team leading, and 10+ years of professional experience in the software development field.
Hazem also is a co-founder and chairman of Ayaady for Investment and Agriculture, a crowd funded company and the first Egyptian online fresh meat shop directly from the farm to the consumers.
Before founding Enozom, Hazem was a co-founder & CEO of IRange Software. He also worked at ITWorx and Raya Software after his graduation in 2004 from Alexandria University, Faculty of Engineering, Computer and Systems Department.
https://www.enozom.com