Introduction
Some of you may have encountered exception DbContext has been disposed. Here is basic explanation of why this error occurs and how to fix it.
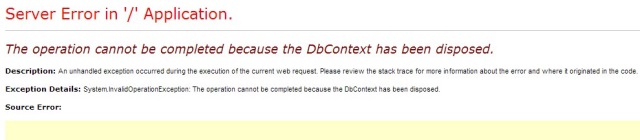
Background
For our needs, let’s use a simple ASP.NET MVC application and Entity Framework to access database.
Source Code
For instance, let’s assume we have source code that returns collection of all products, which have price greater than or equal to 0
.
public ActionResult Index()
{
return View(GetAllProducts());
}
private IEnumerable<Product> GetAllProducts()
{
using (var context = new Context.Context())
{
var list = context.Products.Where(p => p.Price >= 0);
return list;
}
}
If we set a breakpoint, we’ll see that query actually doesn’t execute, as some of you may have expected.

When such IEnumerable
/IQueryable
instance is passed into view, the above mentioned exception is raised. To execute this query, we simply cast such instance to list
.
public ActionResult Index()
{
return View(GetAllProducts());
}
private IEnumerable<Product> GetAllProducts()
{
using (var context = new Context.Context())
{
var list = context.Products.Where(p => p.Price >= 0).ToList();
return list;
}
}
And voila!
