Introduction
Every chance I get, I try to use the Language INtegrated
Query, or LINQ, which provides data querying tools inside the Microsoft .NET
Framework. Why? Because that’s the only way I can possibly make it a part of my
intuitive thinking in coding. I try to adhere to what Bjarne Stroustrup once said,
"Think in terms of algorithms, not loops." LINQ does that.
Anyway, it was time to make pancake with my daughter. We use
Aunt Jamima’s buttermilk mix: just add water. Since my daughter is now learning
fractions, pancake mix creates useful lessons in fractions. The instructions
say mix 2 cups of mix with 1.5 cups of water. Great. But that makes a lot of
pancakes. Some get thrown away. Not good. So, my question to her was, "Can you
reduce the recipe by ¼?"
Reducing 2 to 1.5 was easy, but not the other one. We had to
resort to paper and pencil--in case you're not smarter than a fifth grader, here is the solution:
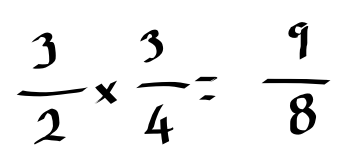
Got the answer, and we made the pancakes. And my
mind began to think about LINQ: pancake mix, water…list…reduce by ¼. How would
I code that? Loops?! No. How would I code it in LINQ in C#?
First I put the ingredients in a list:
List<double> numbers = new List<double>() { 2.0, 1.5 };
Done: 2.0 represents the 2 cups of mix, and 1.5 represents
the 1.5 cups of water.
And what’s the one-line LINQ statement that would give me
what I need? (Drumroll please):
var query = numbers.Select(x => { x = x * 3/4; return x; });
Look what a beautiful expression this is!
No loop! Look at that anonymous function
{ x = x * 3/4; return
x; }
applied to each element,
x
, of the list. "=>" is the operator that
applies the function to an element; it’s called the lambda operator.
A reviewer of this tip noted that it can be made even simpler by simply writing:
var query = numbers.Select(x => x * 3/4);
Even more beautiful! (However, in the first one-liner anonymous function is illustrated and I believe it's worthwhile to see that syntax.)
After writing such a query, I had to reward myself with a
loop ;) to display the result:
foreach (double x in query)
{
Console.WriteLine(x);
}
When you run the code, you get this:
1.5
1.125
The problem is that "1.125
" is not how it’s shown on a measuring
cup. I’ll simply modify the display routine (not modify the list!):
foreach (double x in query)
{
Console.WriteLine((int)x + " and 1/" + 1/(x-(int)x) );
}
There:
1 and 1/2
1 and 1/8
Incidentally, that’s how ancient Egyptians expressed mixed
fractions. It’s useful when displaying in ASCII, too. If I wrote "1 and 1/2" as "11/2"
it would be wrong, wouldn’t it?
If you introduced a new, mutable, variable inside the loop,
you’d also be raising the risk of creating a bug; if not now, later. So, please
be careful with loops.
Oh, we also ate our delicious pancakes!